Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial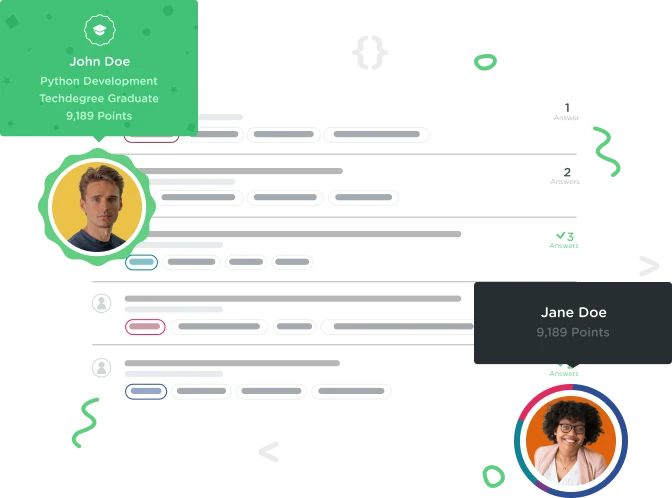

isaac brakha
6,781 PointsHello, why is my code challenge wrong? (functions course)
this is the objective, thank you in advance.
For this challenge, let's say we're building a component of an app that allows a user to type in a location of a famous landmark and get back the geographical coordinates.
To keep the scope limited, let's say we're only working with the following values.
Eiffel Tower - lat: 48.8582, lon: 2.2945 Great Pyramid - lat: 29.9792, lon: 31.1344 Sydney Opera House - lat: 33.8587, lon: 151.2140
Declare a function named getTowerCoordinates that takes a single parameter of type String, named location, and returns a tuple containing two Double values (Note: You do not have to name the return values)
Use a switch statement to switch on the string passed in to return the right set of coordinate values for the location.
For example, if I use your function and pass in the string "Eiffel Tower" as an argument, I should get (48.8582, 2.2945) as the value.
For the default case in the switch statement, return (0,0)
// Enter your code below
func getTowerCoordinates(location: String) -> (lat: Double, lon: Double) {
var lat: Double
var lon: Double
switch location {
case "Eiffel Tower": lat = 48.8582; lon = 2.2945
case "Great Pyramid": lat = 29.9792; lon = 31.1344
case "Sydney Opera House": lat = 33.8587; lon = 151.2140
default: 0.0;0.0
}
return (lat, lon)
}
2 Answers
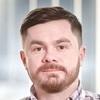
Pavel Fomchenkov
20,897 PointsYour lat
and lon
variables do not get initialized (ever assigned any value) before the return
statement in the default
case (because they are just left var
'ed and are not assigned to anything later). Initialize them to 0.0
at the declaration stage (var lat = 0.0
) and just don't do anything in the default
case (you cannot omit this case itself, so just don't do anything inside it like that: default: break
) or print some useful message with default: print(
"Unknown town, Isaac!")
.
Or just set them both to 0.0
in this case. Almost forgot about his opportunity.

Janam Dave
1,683 PointsI had the same problem, it really frustrated me. I went back to read the directions and they say you don't need to name the parameters of which you will return values of type Double. So, completely take out the variables lon and lat from your declaration and just simply say func getTowerLocation(Location: String) -> (Double, Double) {
Then, In your case statements, just return the appropriate longitude and latitude values instead of setting them equal to specific numbers. You have to do all this because of that piece in the directions, you're not wrong here (and your way works) it's just the problem can't check your answer correctly unless you do it this way, for some very stupid reason.
Brian Bangma
1,514 PointsBrian Bangma
1,514 PointsSame issue for me. Even with the above code, which works in a playground, it does not pass the challenge. The code produces a tuple but the challenge says it does not.