Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial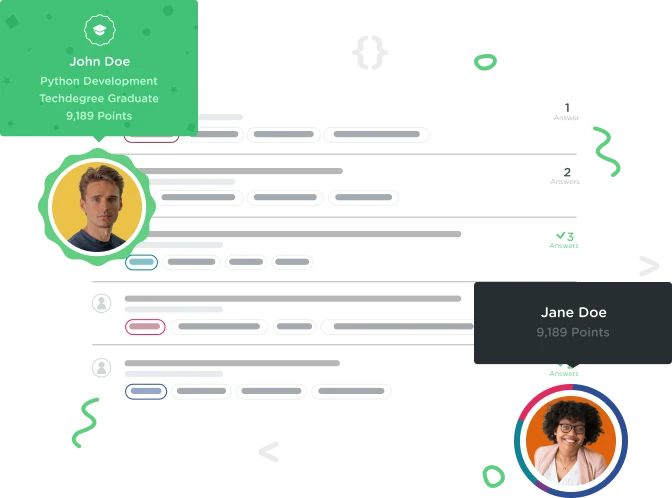

Scott Roriston
5,518 PointsHello, with the Python Dictionaries Word Count example, my code is not being accepted with "Didn't get the right" error
I tried the same code in the Python interpreter and got the{'i': 2, 'am': 2, 'that': 1} result. Am I missing something? Many thanks for the help.
# E.g. word_count("I am that I am") gets back a dictionary like:
# {'i': 2, 'am': 2, 'that': 1}
# Lowercase the string to make it easier.
# Using .split() on the sentence will give you a list of words.
# In a for loop of that list, you'll have a word that you can
# check for inclusion in the dict (with "if word in dict"-style syntax).
# Or add it to the dict with something like word_dict[word] = 1.
def word_count(string):
string_lower = string.lower()
string_list = string_lower.split()
word_count_list = {}
for word in string_list:
word_count = 1
if word in word_count_list:
word_count += 1
word_count_list[word] = word_count
return word_count_list
word_count("I am that I am")
3 Answers

Gunhoo Yoon
5,027 PointsAndreas cormack said he couldn't find anything wrong but there is a logic error in your code.
Your word count won't count higher than 2.
The bugged line is this
word_count = 1 # Reset the word_count on every iteration
Let's see what happens when string is "foo foo foo ..."
string_list
will be ['foo', 'foo', 'foo', 'foo', ...]foo is in
string_list
->word_count
set to 1 -> foo is not inword_count_list
->word_count_list
now has { 'foo': 1 }foo is in
string_list
->word_count
set to 1 -> foo is inword_count_list
,word_count
set to 2 ->word_count_list
now has { 'foo': 2 }foo is in
string_list
->word_count
set to 1 -> foo is inword_count_list
,word_count
set to 2 ->word_count_list
now has { 'foo': 2 }
And as more foo appears word_count
will still be 2.
Also, I would not call word_count_list
since it's actually a dictionary and Python has data type called list. It's confusing when you first encounter them.
You can refer to this if you need.
def word_count(string):
# string is not used anymore within function so no need to keep the integrity.
words = string.lower().split()
result = {}
# Only two cases exist: word is first seen or already seen.
for word in words:
if word in result:
result[word] += 1 # when word already exist.
else:
result[word] = 1 # when word first encountered and only if in that case.
return result

Scott Roriston
5,518 PointsTHANKS Gunhoo!
That was a great explanation and I was able to fix the problem.
Also thank you Andreas for looking over it anyway.

Gunhoo Yoon
5,027 PointsIt's common mistake don't worry about it. It's always good to test your code with varying range of examples. -Happy coding.
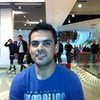
Andreas cormack
Python Web Development Techdegree Graduate 33,011 PointsI took Scott's code run it locally and with an indentation and an else statement got the correct output but like Gunhoo said the logic falls over for anything more than 2.
So well done Gunhoo Yoon for spotting that.
sradms0
Treehouse Project Reviewersradms0
Treehouse Project ReviewerMine was similar to this except i put the number of letters in a count variable, but it was wrong though? Could someone explain? I got the correct result in the interpreter: