Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial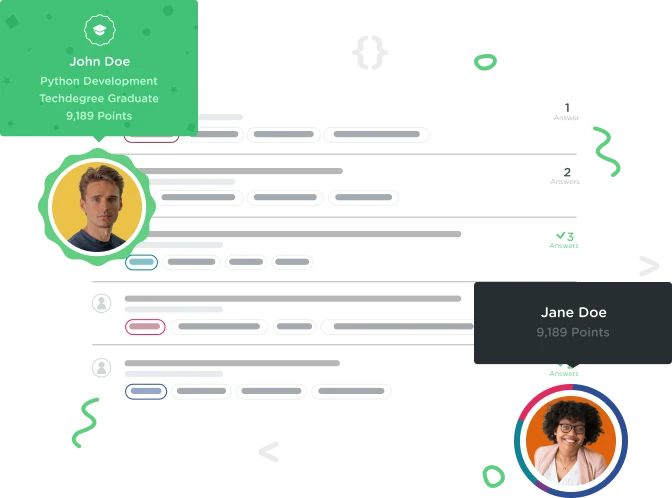

Kyle Lambert
1,969 PointsHello World App with Swift 2.0
Hey guys, I've almost finished my Hello World app, its working but its changing UILabel to something strange, hope someone can help out...
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var nameLabel: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func helloWorldAction(nameTextField: UITextField) {
nameLabel.text = "Hi \(nameTextField.text)"
}
}
The code runs fine but when I enter my name into the text field, my nameLabel should change to 'Hi Kyle' but it changes to 'Hi Optional("Kyle")'.
If someone knows whats going on here, help a brother out. Thanks :)
4 Answers

Greg Kaleka
39,021 PointsHi Kyle,
UITextField has a property text
, which you're accessing. However, the field is of type Optional(String), meaning it's an optional value, and could be nil. This is a very common thing in Swift, and you'll need to deal with optionals a lot in your code.
Aaron showed you the simplest way to do this. Adding an ! to the optional value will "force-unwrap" the optional, and convert it into a non-optional String.
@IBAction func helloWorldAction(nameTextField: UITextField) { nameLabel.text = "Hi \(nameTextField.text!)" }
This is dangerous, though, since trying to unwrap a value that actually is nil will crash your program.
Given you're writing a Hello World program, I know you're new to this, so this is probably a bit advanced, but you'll need to quickly get into the habit of properly managing optionals. Here's how I would handle this:
@IBAction func helloWorldAction(nameTextField: UITextField) {
if let nameText = nameTextField.text { // This checks if the text property is nil, and saves it in nameText if not
nameLabel.text = "Hi \(nameTextField.nameText)" // nameText is not an optional, so no ! needed
} else { // it was nil! good thing we didn't try to unwrap it.
nameLabel.text = "Hi there!" // Not ideal, but better than a crash.
}
}
You'll learn more about this as you progress in iOS development, but hopefully this is a nice preview .
Happy Coding
-Greg
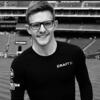
agreatdaytocode
24,757 PointsKyle,
You need to unwrap that value using the ! symbol.
@IBAction func helloWorldAction(nameTextField: UITextField) {
nameLabel.text = "Hi \(nameTextField.text!)"
}

Kyle Lambert
1,969 PointsThanks you everyone, yeah I completely understand now.. I remembered that our course teacher told us never to use force unwrapping, but I couldn't remember how to get around this issue. Seeing this is the case, you could also use a 'guard' ? Thanks

Greg Kaleka
39,021 PointsYes absolutely. Didn't want to throw a guard statement at you if you hadn't seen one already, but it would look like this:
@IBAction func helloWorldAction(nameTextField: UITextField) {
guard let nameText = nameTextField.text else {
nameLabel.text = "Hi there!"
}
nameLabel.text = "Hi \(nameTextField.nameText)"
}
Definitely cleaner - the thing we want to do isn't trapped in an if statement, and if something goes wrong, we're exiting early.

Kyle Lambert
1,969 PointsOkay awesome, thanks so much Greg! Been very helpful :)
agreatdaytocode
24,757 Pointsagreatdaytocode
24,757 PointsI like your answer better :)
Greg Kaleka
39,021 PointsGreg Kaleka
39,021 PointsHah thanks Aaron.