Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial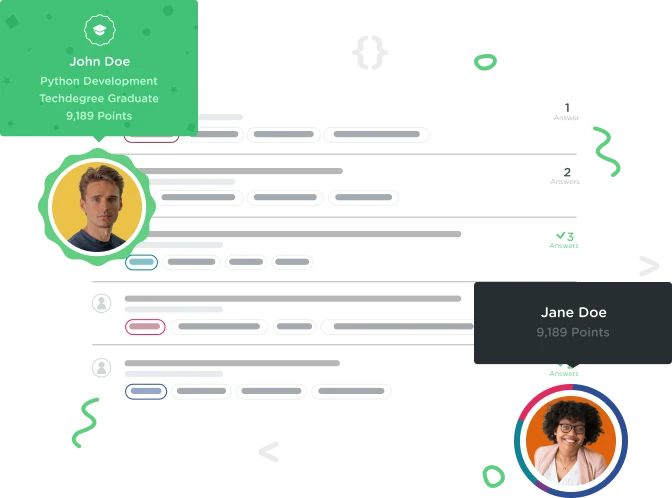
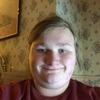
Richard Duffy
16,488 PointsHelp!
<?php
include_once 'inc/connect.php';
cd();
$sql = "SELECT `cat_name` FROM `post_category`";
$query = mysqli_query($mysqli,$sql)or die(mysqli_error($mysqli));
while($row = mysqli_fetch_array($query)) {
$cat = $row['cat_name'];
};
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title> Create a blog post</title>
</head>
<body>
<h1> Create a blog post</h1>
<form action="inc/create_post.php" method="post">
<input type="text" name="title">
<br/>
<br/>
<input type="text" name="author">
<br/>
<br/>
<select name="cat_name">
<?php
echo '<option>'.$cat.'</option>';
?>
</select>
<br/>
<br/>
<textarea rows="10" cols="20" name="post_text"></textarea>
<br/>
<br/>
<input type="submit">
</form>
</body>
</html>
Hello my while loop is only displaying one row from the database when I need it to show all the rows from that database where I have selected it to show from, there are no php errors of any kind.
7 Answers

Coco Jackowski
12,914 PointsYour while loop is running as many times as there are cat_name
s returned, but you're not storing them all. Each time that while loop runs, it's putting the most recently returned cat_name
into the variable $cat
, so when you echo $cat
in your <select>
element, you're just getting the last cat_name
.
Better to do something like this:
$cat_names = array();
while($row = mysqli_fetch_array($query)) {
$cat_names[] = $row['cat_name'];
};
And farther down in your HTML:
<select name="cat_name">
<?php
foreach ($cat_names as $cat_name) {
echo '<option>' . $cat_name .' </option>';
}
?>
</select>
This will store all the cat_name
s you get from your database query into an array, then loop through that array to display them.
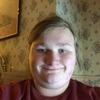
Richard Duffy
16,488 PointsThanks that has worked Well done!

Coco Jackowski
12,914 PointsNo problem. It's usually best to use arrays or objects to store data retrieved from a database when you know it will span multiple rows, so keep those things handy in your development toolbox.
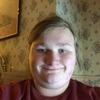
Richard Duffy
16,488 PointsHow do you do this for multiple items like this
<?php
include_once 'connect.php';
cd();
$sql = "SELECT `id`, `cat_name` FROM `post_category` ORDER BY `cat_name` DESC";
$query = mysqli_query($mysqli,$sql);
$cat_name = array();
while($row = mysqli_fetch_array($query)) {
$cat_name[] = $row['cat_name'];
$id = $row['id'];
}
foreach($cat_name as $cat){
echo '<a href="cat.php?id='.$id.'">'.$cat.'';
echo '<br/>';
}
?>

Coco Jackowski
12,914 PointsYou can create a multidimensional array, which is an array that contains arrays. Each row returned from your query can be represented by an array:
<?php
$individual_cat_info = array(
'id' => 1,
'cat_name' => 'Schmoopie'
);
?>
Then you can use the array_push()
function to push each $individual_cat_info
array into a containing $all_cats_info
array:
<?php
$all_cats_info = array();
array_push($all_cats_info, $individual_cat_info);
?>
You can do this dynamically in your while loop, as you're retrieving data from the table:
<?php
$all_cats_info = array();
while($row = mysqli_fetch_array($query)) {
$individual_cat_info = array(
'id' => $row['id'],
'cat_name' => $row['cat_name']
);
array_push($all_cats_info, $individual_cat_info);
}
?>
And then echo out the data:
<?php
foreach ($all_cats_info as $cat_info) {
echo '<a href="cat.php?id=' . $cat_info['id'] . '">' . $cat_info['cat_name'] . '</a>';
}
?>
Multidimensional arrays are extremely useful for retrieving complex data from databases.
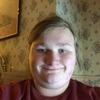
Richard Duffy
16,488 PointsThank you so much, this will help me with my other projects!

Coco Jackowski
12,914 PointsI'm glad you find it useful. The PHP documentation for arrays can be found at:
http://www.php.net/manual/en/language.types.array.php
The official documentation can be a bit dry and difficult, but it's worth looking at to get a better grasp on just how much you can do with arrays, what functions are available for working with arrays, and so on.