Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial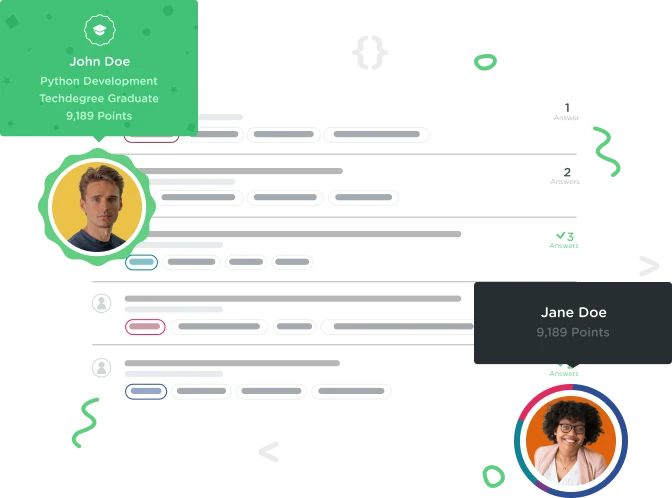
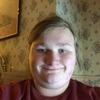
Richard Duffy
16,488 PointsHelp.
I am trying to retrieve data from open weather map and I keep getting this error: Uncaught SyntaxError: Unexpected end of input, below is my code.
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<script>
var x1 = new XMLHttpRequest();
x1.open('GET', 'http://api.openweathermap.org/data/2.5/weather?q=London');
x1.onreadystatechange = function () {
var w = JSON.parse(x1.responseText);
var h = '<ul>';
for(var i=0; i<w.length; i += 1){
h += '<li>';
h += w[i].temp;
h += '</li>';
}
h += '</ul>';
document.getElementById('box').innerHTML = h;
}
x1.send();
</script>
</head>
<body>
<div id="box">
</div>
</body>
</html>
3 Answers

Aaron Graham
18,033 PointsI think you are trying to parse the responseText into JSON before it is completely received. The way your code is currently structured, you are trying to parse responseText
into JSON every time your x1
object changes states, not just when it has finished receiving data. Try adding a check for readyState in your callback:
x1.onreadystatechange = function () {
if(x1.readyState === 4) {
var w = JSON.parse(x1.responseText);
var h = '<ul>';
for(var i=0; i<w.length; i += 1){
h += '<li>';
h += w[i].temp;
h += '</li>';
}
h += '</ul>';
document.getElementById('box').innerHTML = h;
};
}
You might also want to check for the response status code.
Also, depending on what you get back from the server, your for loop might not work. The variable w
is going to be an object, and as such, might not have a .length
attribute. Sometimes APIs will return a single object if you request a single item, or an array of objects if you request a collection of items. You might want to find out what the structure of the JSON response looks like to be sure there isn't a better way to extract the .temp
attribute.

Robert Covington
1,573 PointsYou appear to be missing a semicolon on line 18. Right before the line that says x1 = send();. Since the function is being assigned to x1.onreadystatechange, you need to be sure to close the assignment with a semicolon. Hope that helps!
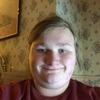
Richard Duffy
16,488 PointsThanks!