Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial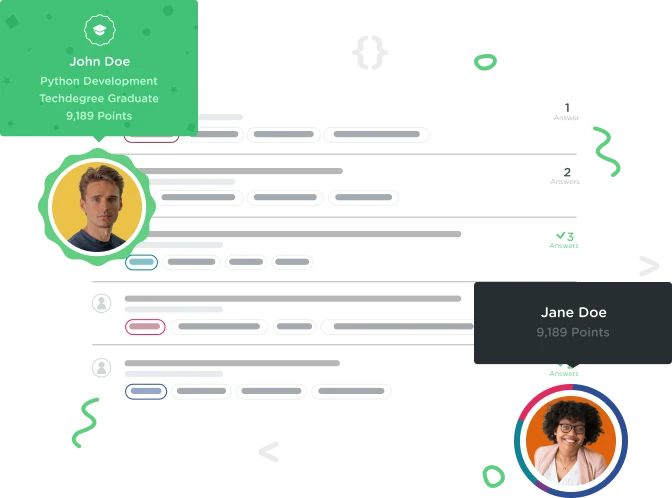

Terrence Adderley
Courses Plus Student 775 PointsHELP !
I believe I have all the parameters set up correctly just might have some wording incorrect.
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
3 Answers
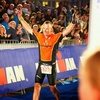
Steve Hunter
57,712 PointsHi Terrence,
Here, you need to create a new drive
method that uses the existing one.
The method needs to do take one lap from the Kart's ability. The existing drive
method has an integer passed to it to determine what is deducted from the Kart's ability. What we need now is the ability to quickly reduce that by one.
So:
public void drive() {
drive(1);
}
I hope that makes sense!
Steve.
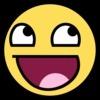
Michael Hess
24,512 PointsHello Terrence,
The challenge is asking you to create another drive method, that doesn't return anything ( a void method), to decrease the carts capability to complete a lap by 1 lap.
Try this:
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
// Calls existing drive method to decrease carts lap capability by 1 lap
drive(1);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
Hope this helps!

Damien Watson
27,419 PointsHi Terrence,
The challenge is asking you to create a new 'drive' method that takes no parameters but performs 1 lap.
Remember that you can have multiple methods with the same name but different arguments. This means you can create a new method without arguments that calls a method with arguments, saving you writing more code.
function drive() {
drive(1);
}

Damien Watson
27,419 PointsYeh, what Steve said. ;)