Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial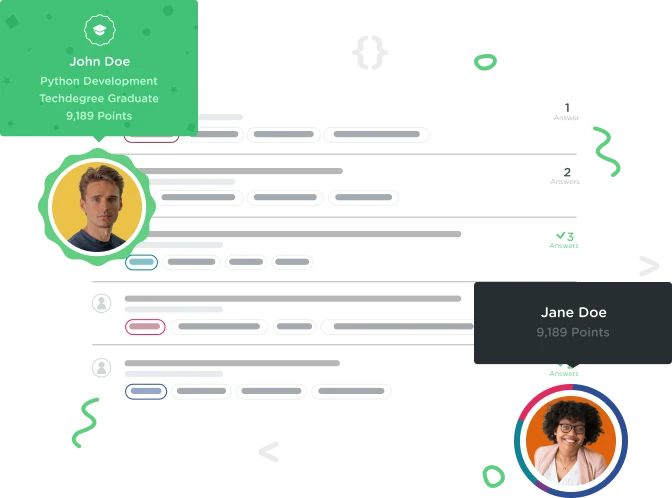

Rogelio Valdez
6,244 PointsHelp
Help!! I don't think I really understood the task for the challenge so I am messing my code up and rewriting it over and over again and now it's a complete mess!! I am getting all kinds of compile error and even if it didn't had them The program will still not do what it should since I'm not understanding what I should do.
Can any one help me out with what the taks is for this challenge? In Blog.java add a new method called getCategoryCounts. It should return a Map of category to count calculated by looping over all the posts.
P.S. Please don't spoil the answer I want to get there my self. Thanks.
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.Arrays;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
import com.example.BlogPost;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
public Map<String, List<BlogPost>> getCategoryCounts() {
Map<String, List<BlogPost>> postsByCategory = new HashMap<String, List<BlogPost>>();
for (BlogPost myPost : mPosts) {
postsByCategory = categoryCounts.getCategory());
if (postsByCategory == null) {
postsByCategory = new ArrayList<BlogPost>();
categoryCounts.put(categoryCounts.getCategory(), postsByCategory);
}
postsByCategory.add(categoryCounts);
}
return categoryCounts;
}
}
2 Answers

Seth Kroger
56,413 PointsThe challenge is asking for how many posts are in a category, not for the list of them. So you should be returning a Map<String, Integer>.

Rogelio Valdez
6,244 PointsSo that takes me a little closer.. I hope.
I am still getting something wrong since I am not understanding the for loops for this exercise (I did understood the use of them in the lesson or I least I think I did). I am making a public method, that returns a Map in the form String,Int called CategoryCounts receives no arguments. I created a new HashMap named categoryCounts. Then I have a type Blogpost for the variable blog. I should then get a String that is the categories(one at a time) and store them in categoryCounts and add to the counts if they are repeated.
If I submit my code like this I get this error:
./com/example/Blog.java:33: error: cannot find symbol
for (String categories : blog.getPosts()) {
^
symbol: method getPosts()
location: variable blog of type BlogPost
Note: JavaTester.java uses unchecked or unsafe operations.
Note: Recompile with -Xlint:unchecked for details.
1 error
public Map<String, Integer> getCategoryCounts() {
Map<String, Integer> categoryCounts = new HashMap<String, Integer>();
for (BlogPost blog : mPosts) {
for (String categories : blog.getPosts()) {
Integer count = categoryCounts.get(categories);
if (count == null) {
count = 0;
}
count++;
categoryCounts.put(categories, count);
}
return categoryCounts;
}
Rogelio Valdez
6,244 PointsRogelio Valdez
6,244 PointsThank you! This helps a lot since I had no idea what the exercise is asking. I am still having some trouble with the for loops. Could you help me out? (not giving me the answer)