Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial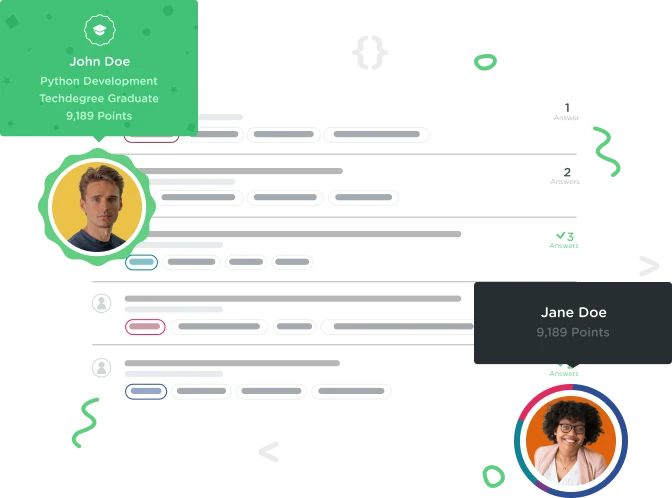

Andrew Alvarez
11,964 PointsHELP!
Challenge Task 1 of 1
Fill in the find_index method to return the index of a todo item in the @todo_items array given the name. The method should return the index of the item if the item is found and nil if it is not found.
Bummer! Try again!
what am i doing wrong uugh!
def find_index(name)
index = 0
found = false
todo_items.each do |todo_item| #add each method to loop through array
if todo_item.name == name
found = true
break;
end
index += 1
end
if found
return index
else
return nil
end
end
1 Answer

Tim Knight
28,888 PointsThere are a few ways you could do this, first though you want to make sure you're only increasing your index count if you're not meeting the condition so that should be within an else statement.
def find_index(name)
index = 0
found = false
todo_items.each do |todo_item|
if todo_item.name == name
found = true
end
if found
break
else
index += 1
end
end
if found
return index
else
return nil
end
end
That does solve the problem, though that solution might feel a bit verbose to you it's the solution from within the course.
You can bring it down by using ternary operators, like this:
def find_index(name)
index = 0
found = false
todo_items.each do |todo_item|
found = true if todo_item.name == name
found ? break : index += 1
end
return found ? index : nil
end
You sacrifice some readability depending on the developer and the readers familiarity with the language.