Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial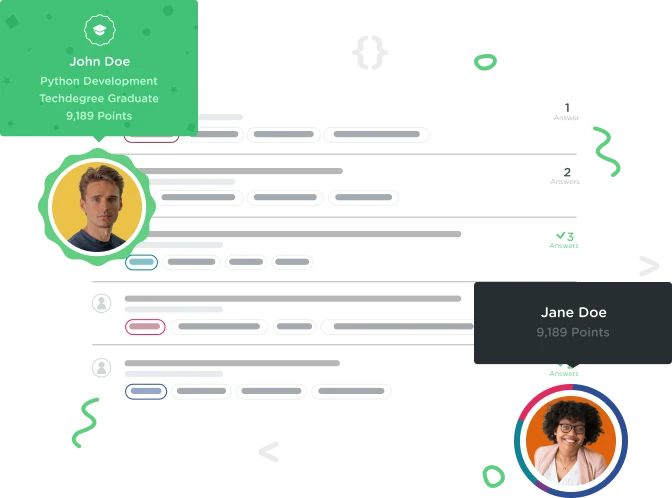

Stefan Vaziri
17,453 PointsHelp
Unsure how to have two arguments and count how many of the items in the list are in the other list. Used the count function but that didn't work either.
# You can check for dictionary membership using the
# "key in dict" syntax from lists.
### Example
# my_dict = {'apples': 1, 'bananas': 2, 'coconuts': 3}
# my_list = ['apples', 'coconuts', 'grapes', 'strawberries']
# members(my_dict, my_list) => 2
def members(d, l):
d = {'apples':1,'bananas':2,'coconuts':3}
l = ['apples','coconuts','grapes','strawberries']
return members(d,l))
3 Answers
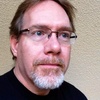
Chris Freeman
Treehouse Moderator 68,454 PointsThe code needs to iterate over the list and check if item is in the dict.
# members(my_dict, my_list) => 2
def members(d, l):
count = 0
# for each item in list
for item in l:
# check if otem is a dict key
if item in d: # same as 'if kitem in d.keys()'
# add to count
count += 1
return count

Khambrel Davis
24,572 Pointsdef members(d, l):
count = 0
for key in d:
if key in l:
count += 1
return count
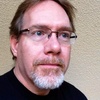
Chris Freeman
Treehouse Moderator 68,454 PointsI suggest reversing your use of l and d. If the dictionary is very large relative to the list, it will run quicker looking for each list item in the dict keys.

Khambrel Davis
24,572 PointsThanks, I see what you are saying.
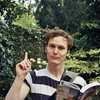
Bart Bruneel
27,212 PointsHello Stefan,
First of all, there is no need to the define the list and dictionary inside your function. These arguments will be passed to the function if it gets called in the evaluation. I would approach the problem as follows:
- first you'll need to extract the keys from the library. This can be done with the .keys() method. This method returns a list of the keys.
- Then you set a count to zero. -Subsequently you loop over the list-items in my_list.
- If the item is also in the keys-list, you add 1 to the count.
- return the count.
Happy coding.
Stefan Vaziri
17,453 PointsStefan Vaziri
17,453 PointsCan you explain to me why you used "count = 0" and "count +=1"?
Khambrel Davis
24,572 PointsKhambrel Davis
24,572 PointsThe goal of the function is to return a count of how many times we can find a key from the dictionary that is also listed in the list. The count variable is first set to zero then each time a key is found in the dictionary and list, the count variable is increased by one. The for loop does the search and the if condition checks each loop/cycle to see if there is a match.
Chris Freeman
Treehouse Moderator 68,454 PointsChris Freeman
Treehouse Moderator 68,454 PointsA standard way to accumulate a "count" is to add one and assign back to
count
:count = count + 1
The Python shorthand for this is to use the "
+=
" notation to mean the same thing:count += 1 # Same as count = count + 1
The final part is setting an initial value for
count
. This is done so the right-side of the equation "count + 1" does not raise an NameError error due tocount
not yet being set on the first iteration. The simplest way to setcount
is to give it a default starting value before the loop:count = 0