Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial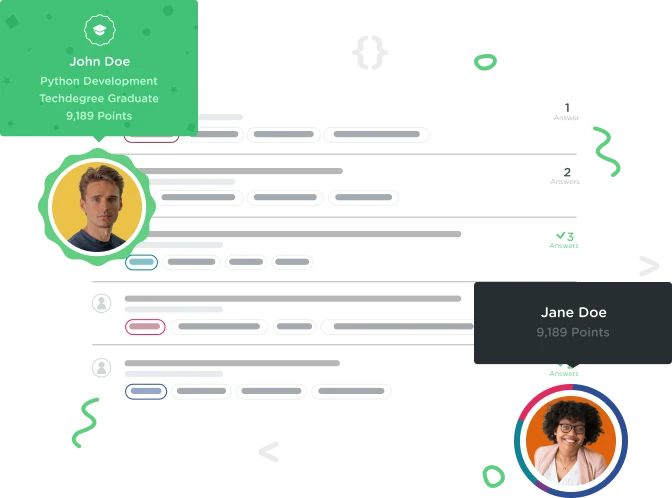

jjane
642 PointsHELP, covert greet function to arrow function- been stumped for a day
Hi need help with arrow function. Here's my answer, but it says I need to make the argument a string?
const greet(Hi, val) => {
const coder = `${val}!`;
return `Hi, ${val}!`;
}
greet(Hi, val);
3 Answers
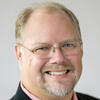
Jason Larson
8,361 PointsWhile Joseph Yhu is correct, his answer is a shorthand way to write an arrow function. It might help your understanding when looking at the code if it looked more like what you were originally given, so you can change the original function to look like this:
const greet = (val) => {
return `Hi, ${val}!`;
}
greet('cool coders');
Also (to be thorough), you'll note in the original code that they are calling greet()
with the value 'cool coders'
(with single quotes around it). In your code, you are passing in Hi, val
without quotes, which means that the system thinks you're passing in 2 values: the first is an undefined variable named Hi
and the second is an undefined variable named val
. Additionally, your rewrite is trying to accept 2 arguments: the first named Hi
and the second named val
. You only want to accept a single parameter (val
), and you want to pass it a string. Strings are always surrounded by quotes (single or double).
Finally, Remember that you are assigning the function to the const greet
, and anytime you declare a const, you have to use =
to assign a value.
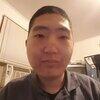
Joseph Yhu
PHP Development Techdegree Graduate 48,638 Pointsconst greet = val => `Hi, ${val}!`;
Also move the arrow function above the line calling it, i.e. greet('cool coders');
.

jjane
642 PointsThank you for the response but I don't understand passing the argument

jjane
642 PointsJason Larson, yes, that's exactly what I was thinking, thank you so much for this breakdown! You're too kind taking the time to explain that, I appreciate it very much!
Do you have an email/contact ppl can reach you at? Would love to keep in touch.
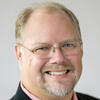
Jason Larson
8,361 PointsI do have several email addresses, but to be perfectly honest with you, I rarely check them, unless I'm expecting something. Additionally, I would prefer not to post a legitimate email on a public forum, as that is just asking for tons of extra spam, and I already get enough of that as it is. Having said that, you can email me at catchingspam@yahoo.com and I'll check that sometime in the next day or so and reply to you directly with a better address. Just be aware of what I said about not checking my mail very often, so if it's a technical question or something that is time-sensitive, you're better off asking in these forums, searching google, or asking on stackoverflow.
jjane
642 Pointsjjane
642 PointsThank you so much with this through response! I was trying to figure out how to pass an argument... I still think I don't get it completely lol.
Why does the argument string not matter as 'cool coders' = 'val'?
Also for string template literals, I thought the backtick was needed in the case of ${val} in "Hi, ${val}" which is why I was completely thrown off.
Jason Larson
8,361 PointsJason Larson
8,361 PointsI'm not sure what you mean when you say "Why does the argument string not matter as 'cool coders' = 'val'?", but I'll try to answer as best I can, based on what I think you might be thinking, based on the code you wrote. I am assuming you understand variable assignment, as in:
const someVariable = 'some value'
In this code, the variable named
someVariable
is assigned the string "some value", so if you writeconsole.log(someVariable)
you would get
some value
output in the console. You can think of the parameters of a function the same way. If you have the following code:you would get
some value
output in the console. In essence, the value 'some value' is assigned to the variable named paramA in the function. In the same way, you can pass a variable to a function. So with this code:you would get
some value
output in the console. In the same way, a function can accept more than one parameter, and you can pass more than one value into a function, if the parameters and the values are separated with a comma, as in the following code:This would also give
some value
output in the console. This is what you are doing in your code, because the values you put in the parentheses after the function name are not surrounded with quotes. You are passing a variable namedHi
and a variable namedval
(which are undefined). In your code, you are trying to assign a string to the variablecoder
, but that isn't necessary. For one thing, you never use the variable after the assignment. You are correct about the template literals needing the backticks (that's what makes it a template literal), and when a variable is entered in a template literal as${someVariable}
, it translates the variable into the string.A thought just occurred to me as I'm typing this. Is it possible that you mistook the single quotes around
cool coders
as backticks? I could see where you might think that you needed to do something special with the string if you thought the backticks were causing the system to perform some function on the words, but when you put just words within backticks, the system treats the value as a string, in much the same way that it would if you had used quotes.Does that help?
jjane
642 Pointsjjane
642 PointsJason Larson
Just shot over a quick email!