Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial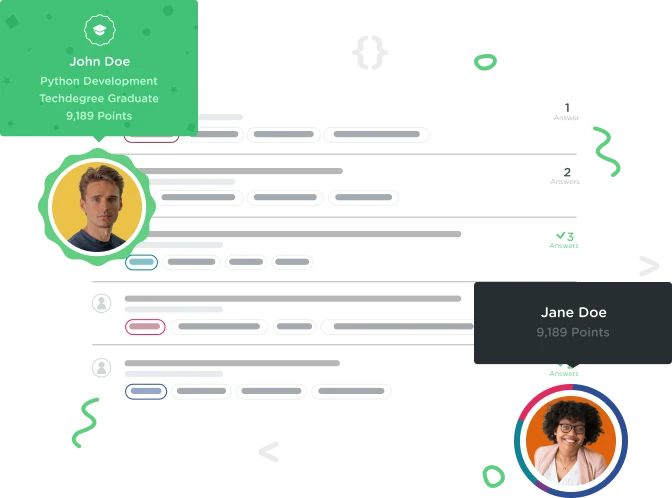

Jalen Wize
Courses Plus Student 2,559 PointsHelp eliminating vowels from words
Hi, I am trying to write a function that iterates through a given word and removes any vowels. Please let me know if you see anything I'm doing wrong! Thanks!
def disemvowel(word):
word_list = list(word)
index = 1
for letter in word_list:
if letter.lower() == "a" or letter.lower() == "e" or letter.lower() == "i" or letter.lower() == "o" or letter.lower() == "u":
word_list.remove(letter)
index +=1
return word
1 Answer

Benjamin Lange
16,178 PointsHi there,
Your method looks great! You properly removed the vowels from word_list. There is one minor issue that is causing your method to return the incorrect answer: you neglected to update the word variable with the result. Set the word variable equal to the remaining characters in word_list using the join method as demonstrated in the below code snippet.
word = ''.join(word_list)
Let me know if you have any further questions.
Quick edit: You can remove the index variable from your code. Python for loops do not typically require the use of an index variable.
Jalen Wize
Courses Plus Student 2,559 PointsJalen Wize
Courses Plus Student 2,559 PointsThank you for response! My code is running now but I'm running into an error where if there are consecutive vowels it will miss the second one. For example: Monsoon will return Mnson
Benjamin Lange
16,178 PointsBenjamin Lange
16,178 PointsHi there! It looks like I missed another detail! What's happening here:
Thankfully, this is an easy fix.