Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial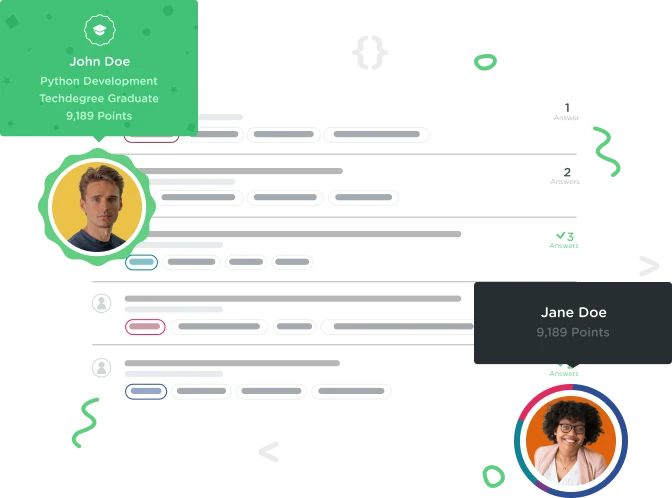
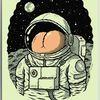
Tosin Peters
9,541 PointsHelp! Error - Not getting weather data
I've followed the step-by-step instructions in the video but when I run my app. The console generates an error and doesn't print out the data on the console like in the video.
08-13 19:34:00.520 10922-10922/? I/art: Not late-enabling -Xcheck:jni (already on) 08-13 19:34:00.695 10922-10934/com.droidtech.stormy I/art: Background sticky concurrent mark sweep GC freed 5244(338KB) AllocSpace objects, 0(0B) LOS objects, 11% free, 1001KB/1135KB, paused 6.429ms total 26.466ms 08-13 19:34:00.806 10922-10922/com.droidtech.stormy D/MainActivity: Main UI code is running 08-13 19:34:00.834 10922-10950/com.droidtech.stormy D/OpenGLRenderer: Render dirty regions requested: true 08-13 19:34:00.837 10922-10922/com.droidtech.stormy D/: HostConnection::get() New Host Connection established 0xa6736b30, tid 10922 08-13 19:34:00.846 10922-10922/com.droidtech.stormy D/Atlas: Validating map... 08-13 19:34:00.917 10922-10950/com.droidtech.stormy D/: HostConnection::get() New Host Connection established 0xa67c2b50, tid 10950 08-13 19:34:00.930 10922-10950/com.droidtech.stormy I/OpenGLRenderer: Initialized EGL, version 1.4 08-13 19:34:00.952 10922-10950/com.droidtech.stormy D/OpenGLRenderer: Enabling debug mode 0 08-13 19:34:00.975 10922-10950/com.droidtech.stormy W/EGL_emulation: eglSurfaceAttrib not implemented 08-13 19:34:00.975 10922-10950/com.droidtech.stormy W/OpenGLRenderer: Failed to set EGL_SWAP_BEHAVIOR on surface 0xa67c7ec0, error=EGL_SUCCESS 08-13 19:34:01.177 10922-10947/com.droidtech.stormy V/MainActivity: {"code":400,"error":"The given location is invalid."} 08-13 19:34:01.585 10922-10950/com.droidtech.stormy W/EGL_emulation: eglSurfaceAttrib not implemented 08-13 19:34:01.586 10922-10950/com.droidtech.stormy W/OpenGLRenderer: Failed to set EGL_SWAP_BEHAVIOR on surface 0xa67c7fc0, error=EGL_SUCCESS 08-13 19:34:03.043 10922-10950/com.droidtech.stormy D/OpenGLRenderer: endAllStagingAnimators on 0xa56b9680 (RippleDrawable) with handle 0xa67c2ef0
This is my mainActivity class code
public class MainActivity extends AppCompatActivity {
public static final String TAG = MainActivity.class.getSimpleName();
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
String apiKey = "7bd5e39b849d70b6211ebcaf98b00dd4";
double longitude =37.8267;
double latitude = -122.423;
String forecastUrl = "https://api.forecast.io/forecast/" + apiKey + "/" + latitude + "," + longitude;
if (isNetworkAvailable())
{
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder().url(forecastUrl).build();
Call call = client.newCall(request);
call.enqueue(new Callback()
{
@Override
public void onFailure(Call call, IOException e)
{
Log.d(TAG, "Error in the data code");
}
@Override
public void onResponse(Call call, Response response) throws IOException
{
try
{
//Response response = call.execute(); execute() is the synchronous method
Log.v(TAG, response.body().string());
if (response.isSuccessful())
{
//Log.v(TAG, response.body().string()); //string representation of the response body
} else
{
//Log.v(TAG,response.body().string());
alertUserAboutError();
}
} catch (IOException e)
{
Log.e(TAG, "Exception caught: ", e);
}
response.close();
}
});
}
else
{
Toast.makeText(this, R.string.network_unavailable_message, Toast.LENGTH_LONG).show();
}
Log.d(TAG, "Main UI code is running");
}
private boolean isNetworkAvailable()
{
ConnectivityManager manager = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected())
{
isAvailable=true;
}
return isAvailable;
}
private void alertUserAboutError()
{
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
}
Any help would be appreciated. Thanks!
1 Answer

andren
28,558 PointsIt's hard to diagnose coding issues when you don't include your code in the post, almost all of the messages you post are normal messages that are almost always generated when you run apps on an android emulator, and that are safe to ignore, the only message that is remotely helpful in diagnosing your issue is this one:
V/MainActivity: {"code":400,"error":"The given location is invalid."}
With is a message generated by your own code, that is the response you are getting back from the Forecast.io API, based on that response it seems that the latitude or longitude that you supply in the request to Forecast.io is invalid.
Making sure that the lat and long is set to a real location should fix that issue.
Tosin Peters
9,541 PointsTosin Peters
9,541 PointsYou are right! Thank you so much! :)
Yusuf Mohamed
2,631 PointsYusuf Mohamed
2,631 PointsJust one question andren, what do you mean by setting it to a real location? Also Tosin, how did you solve the problem.