Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial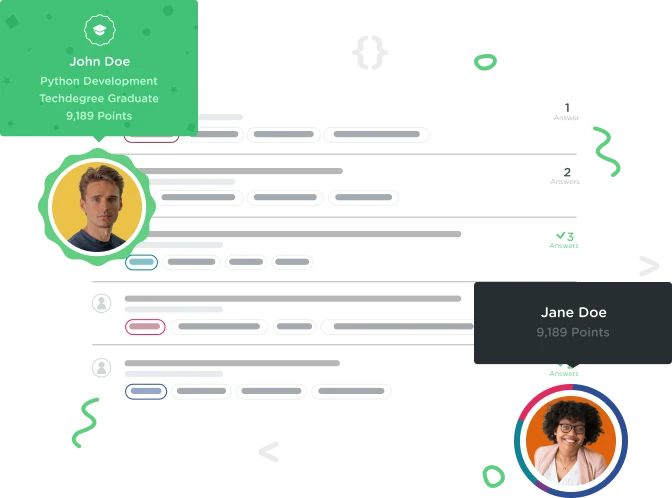
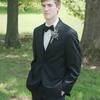
Jamison Imhoff
12,460 PointsHelp explaining a solution for Python Challenge
I was able to solve the python code challenge in the tuples section
"Create a function named combo() that takes two iterables and returns a list of tuples. Each tuple should hold the first item in each list, then the second set, then the third, and so on. Assume the iterables will be the same length."
my solution:
def combo(it1, it2):
output = []
for index, item in enumerate(it2):
output.append ((it1[index], item))
return output
Now I was mostly just trying different things to try to solve it... I would like an in depth explanation (line by line) as to what exactly is being processed and why using plain english (not too much code jargon as I am brand new to coding) as much as possible.
My interpretation is something like
def combo(it1, it2): #define the combo function
output = [] #place holder variable established
for index, item in enumerate(it2): #for position, item in broken down iterable 2 do the following **This step is still fuzzy in my understanding because I am a little confused about how the index and item are interpreted
output.append ((it1[index], item)) #take placeholder and add on (append) the it1 position and item tuples given **this step is still a little confusing to me as well because of the index and item part in the append()
return output #show result
My biggest confusion I guess is understanding the whole index definition (basically just a given position in something..?) and how exactly 'item' is used/interpreted in 'for' loops and even how to "read" how a 'for' loop is done. I like to kind of read through each line in plain english (interpret it) so that I understand step by step what exactly is being done.
It may seem odd to ask about this considering I managed to solve it, but I want to COMPLETELY understand as to why it worked so that in the future It is less trial and error and more step by step analysis of the problem and finding the solution, if that makes sense.
Thanks.
2 Answers
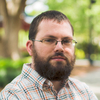
Kenneth Love
Treehouse Guest TeacherYour understand of it is correct. Let's break down what .enumerate()
does, though.
.enumerate()
steps through an iterable, or something that has multiple parts. Lists, dicts, tuples, and strings all have multiple parts and can be considered iterables. Numbers, on the other hand, only have one part, so they aren't and can't be.
OK, so .enumerate()
takes an iterable and makes a variable that we'll call index
. Since Python, like most programming languages, starts counting indexes at 0, index = 0
. Then .enumerate()
takes the iterable that you gave it, and makes a new variable that we'll call value
. value = iterable[index]
, so we get the value from the iterable at that index. Then .enumerate()
returns both of these values as a tuple pair.
So if we have an iterable that, say, a string of "Treehouse"
, and we call .enumerate()
on it, here's what happens.
- OK,
index
is 0,iterable[0]
is"T"
. Send back(0, "T")
. - Is there more to
iterable
? Yep. OK, incrementindex
, send back(1, "r")
sinceiterable[1]
is"r"
. - Is there more? Increment
index
again. Send back(2, "e")
. - Repeat until
index
is equal to the length ofiterable
.

Emmet Lowry
10,196 Pointsin the example Jamison Imhoff did is output = [] just for storing the information under it and i still dont quite understand how it works.
John Eleftheriou
4,369 PointsJohn Eleftheriou
4,369 PointsI literally jumped out of my chair cheering when I understood .enumerate() due to this explanation. Keep up, mate! :D
Merry Christmas btw!