Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial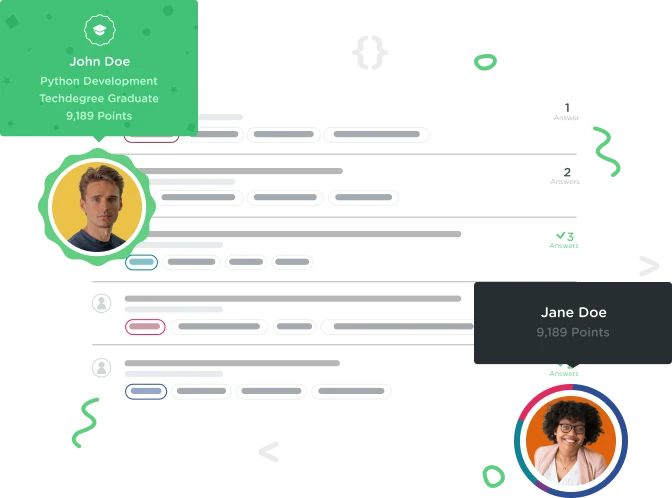

Daniele Bianchi
2,471 PointsHelp for parcelables array
Hello to everyone I've a error in my code, the error is: Caused by: java.lang.NullPointerException: Attempt to get length of null array
this is the code where I recive the error:
public class ElencoAutoActivity extends ActionBarActivity {
public static final String TAG = ElencoAutoActivity.class.getSimpleName();
public static final String ELENCO_AUTO = "ELENCO_AUTO";
private Forecast mForecast;
private Auto[] mAuto;
private AutoAdapter mListAdapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_elenco_auto);
Intent intent = getIntent();
Parcelable[] parcelables = intent.getParcelableArrayExtra(ElencoAutoActivity.ELENCO_AUTO);
mAuto = Arrays.copyOf(parcelables, parcelables.length, Auto[].class);
AutoAdapter adapter = new AutoAdapter(this, mAuto);
setListAdapter(adapter);
}
private void getForecast() {
Bundle bundle = getIntent().getExtras();
String id_segmento = bundle.getString("id_segmento");
String forecastUrl = "http://jam.yussfone-crm.com/remote/jam_to_go_auto_leasing.php?id_segmento=" + id_segmento;
if (isNetworkAvailable()) {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Request request, IOException e) {
alertUserAboutError();
}
@Override
public void onResponse(Response response) throws IOException {
try {
String jsonData = response.body().string();
Log.v(TAG, jsonData);
if (response.isSuccessful()) {
mForecast = parseForecastDetails(jsonData);
runOnUiThread(new Runnable() {
@Override
public void run() {
}
});
} else {
alertUserAboutError();
}
}
catch (IOException e) {
Log.e(TAG, "Exception caught: ", e);
}
catch (JSONException e) {
Log.e(TAG, "Exception caught: ", e);
}
}
});
}
else {
Toast.makeText(this, getString(R.string.network_unavailable_message),
Toast.LENGTH_LONG).show();
}
}
private Auto[] getAutoForecast(String jsonElenco) throws JSONException {
JSONObject forecast = new JSONObject(jsonElenco);
JSONObject autoObj = forecast.getJSONObject("auto");
JSONArray autol = autoObj.getJSONArray("auto");
Auto[] autos = new Auto[autol.length()];
for (int i = 0; i < autol.length(); i++) {
JSONObject jsonAuto = autol.getJSONObject(i);
Auto auto = new Auto();
auto.setMarca(jsonAuto.getString("marca"));
auto.setModello(jsonAuto.getString("modello"));
auto.setCanone(jsonAuto.getDouble("canone"));
//auto.setFoto(jsonAuto.getString("foto"));
autos[i] = auto;
}
return autos;
}
private Forecast parseForecastDetails(String jsonData) throws JSONException {
Forecast forecast = new Forecast();
return forecast;
}
Auto auto = new Auto();
private Auto getAutoDetails(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
JSONObject currently = forecast.getJSONObject("currently");
Auto current = new Auto();
current.setId(currently.getInt("mId"));
current.setQuotazione(currently.getInt("quotazione"));
current.setIdSegmento(currently.getInt("mIdSegmento"));
current.setMarca(currently.getString("mMarca"));
current.setModello(currently.getString("mModello"));
current.setAlimentazione(currently.getString("mAlimentazione"));
current.setEmissioni(currently.getString("mEmissioni"));
current.setDurata(currently.getInt("mDurata"));
current.setChilometraggio(currently.getInt("mChilometraggio"));
current.setCanone(currently.getDouble("mCanone"));
current.setAnticipo(currently.getDouble("mAnticipo"));
current.setPreview(currently.getString("mPreview"));
current.setFoto(currently.getString("mFoto"));
return current;
}
private void alertUserAboutError() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager)
getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()) {
isAvailable = true;
}
return isAvailable;
}
public void setListAdapter(AutoAdapter listAdapter) {
mListAdapter = listAdapter;
}
public AutoAdapter getListAdapter() {
return mListAdapter;
};
}
and this is the class auto:
public class Auto implements Parcelable { public int mId; public int mQuotazione; public int mIdSegmento; public String mMarca; public String mModello; public String mAlimentazione; public String mEmissioni; public int mDurata; public int mChilometraggio; public double mCanone; public double mAnticipo; public String mPreview; public String mFoto;
public Auto() {
}
public int getId() {
return mId;
}
public void setId(int id) {
mId = id;
}
public int getQuotazione() {
return mQuotazione;
}
public void setQuotazione(int quotazione) {
mQuotazione = quotazione;
}
public int getIdSegmento() {
return mIdSegmento;
}
public void setIdSegmento(int idSegmento) {
mIdSegmento = idSegmento;
}
public String getMarca() {
return mMarca;
}
public void setMarca(String marca) {
mMarca = marca;
}
public String getModello() {
return mModello;
}
public void setModello(String modello) {
mModello = modello;
}
public String getAlimentazione() {
return mAlimentazione;
}
public void setAlimentazione(String alimentazione) {
mAlimentazione = alimentazione;
}
public String getEmissioni() {
return mEmissioni;
}
public void setEmissioni(String emissioni) {
mEmissioni = emissioni;
}
public int getDurata() {
return mDurata;
}
public void setDurata(int durata) {
mDurata = durata;
}
public int getChilometraggio() {
return mChilometraggio;
}
public void setChilometraggio(int chilometraggio) {
mChilometraggio = chilometraggio;
}
public double getCanone() {
return mCanone;
}
public void setCanone(double canone) {
mCanone = canone;
}
public double getAnticipo() {
return mAnticipo;
}
public void setAnticipo(double anticipo) {
mAnticipo = anticipo;
}
public String getPreview() {
return mPreview;
}
public void setPreview(String preview) {
mPreview = preview;
}
public String getFoto() {
return mFoto;
}
public void setFoto(String foto) {
mFoto = foto;
}
@Override
public int describeContents() {
return 0;
}
@Override
public void writeToParcel(Parcel out, int flags) {
out.writeInt(mId);
out.writeInt(mQuotazione);
out.writeInt(mIdSegmento);
out.writeString(mMarca);
out.writeString(mModello);
out.writeString(mAlimentazione);
out.writeString(mEmissioni);
out.writeInt(mDurata);
out.writeInt(mChilometraggio);
out.writeDouble(mCanone);
out.writeDouble(mAnticipo);
out.writeString(mPreview);
out.writeString(mFoto);
}
public Auto[] newArray(int size) {
return new Auto[size];
}
public Auto(Parcel in) {
mId = in.readInt();
mQuotazione = in.readInt();
mIdSegmento = in.readInt();
mMarca = in.readString();
mModello = in.readString();
mAlimentazione = in.readString();
mEmissioni = in.readString();
mDurata = in.readInt();
mChilometraggio = in.readInt();
mCanone = in.readDouble();
mAnticipo = in.readDouble();
mPreview = in.readString();
mFoto = in.readString();
}
public static final Creator<Auto> CREATOR = new Creator<Auto>() {
@Override
public Auto createFromParcel(Parcel source) {
return new Auto(source);
}
@Override
public Auto[] newArray(int size) {
return new Auto[size];
}
};
}