Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial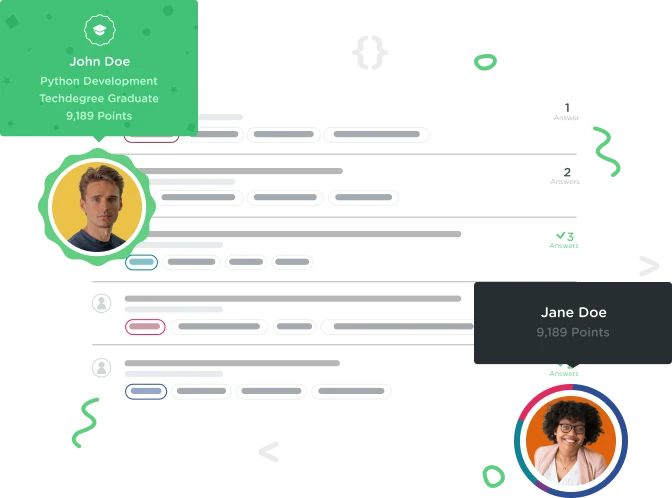
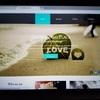
Kyle Jensen
Courses Plus Student 6,293 PointsHELP - getting the message: bummer, index was outside the bounds of the array message on course objective
I have to be missing something simple or there's a bug in this objective.
Override the Scan method to return true if any value in the array is a repeat of the value immediately preceding it.
namespace Treehouse.CodeChallenges {
class SequenceDetector {
public virtual bool Scan(int[] sequence) {
return true;
}
}
class RepeatDetector : SequenceDetector {
public override bool Scan(int[] sequence) {
for (int i = 0; i < sequence.Length; i++) {
if (sequence[i] > 0 && sequence[i] == sequence[i - 1]) {
return true;
}
}
return false;
}
}
}
3 Answers

andren
28,558 PointsIn your if statement conditions
sequence[i] > 0 && sequence[i] == sequence[i - 1]
You access sequence
[i-1] since i
starts at 0 your code will try to access sequence[-1]
on it's first run which is an invalid index. Also while it doesn't cause a direct issue with the task I'm not quite sure what the logic behind your first condition is. It's not something that has to be there to complete the objective.
It's also worth mentioning that you were actually meant to place the RepeatDetector
class is a separate file from the SequenceDetector
class, but doing so is not actually mandatory to complete the challenge.
If you start i
at 1 rather than 0 and remove your first condition like this:
namespace Treehouse.CodeChallenges
{
class SequenceDetector
{
public virtual bool Scan(int[] sequence)
{
return true;
}
}
class RepeatDetector : SequenceDetector{
public override bool Scan(int[] sequence) {
for(int i = 1; i < sequence.Length; i++) {
if(sequence[i] == sequence[i - 1]) {
return true;
}
}
return false;
}
}
}
Then your code will pass the challenge.
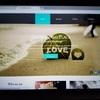
Kyle Jensen
Courses Plus Student 6,293 PointsYou and me both. :)

Jon Wood
9,884 PointsI think you're getting the error because you're doing a i - 1
in the loop. When the loop starts i
will be 0, so it's going to try to get sequence[-1]
which doesn't exist.
Here's a trick I used for when I did the challenge:
- Try using a
foreach
instead. I always find that to be so much easier to read than having to deal with indexes of the array.
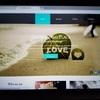
Kyle Jensen
Courses Plus Student 6,293 PointsI realized that I was trying to compare the item in the array to integer 0 instead of the value of i to the integer 0. I could have initialized the loop with i = 1, and it was a thought, but it made it more clear, to me, with what I was doing initially having it in the if statement - because I was thinking incorrectly about what I was comparing. Like I said, simple mistake or bug..simple mistake it was. :)

Jon Wood
9,884 PointsIt's been my experience where it's always a simple mistake. Sometimes, and I've had this happen quite a bit lately, I just need to take a break and come back to it with fresh eyes. :)