Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial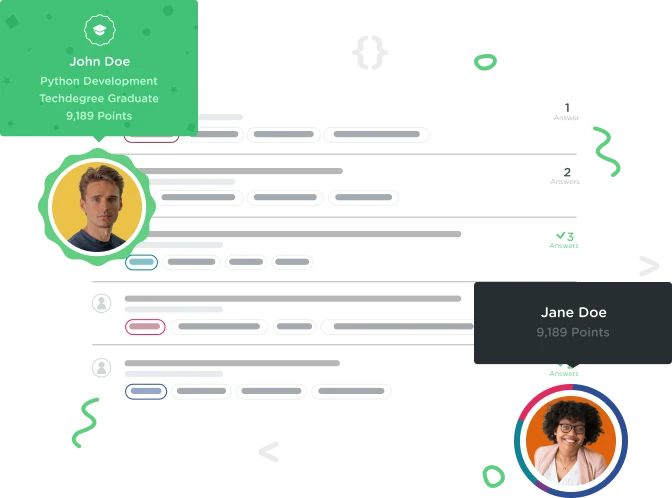

Solomon Asiedu-Ofei Jnr
1,015 PointsHELP!! I am supposed to return a true or false if my tiles already contain a tile I pass.
I have added a boolean to return a true when it contains a tile already in my tiles but how do I return a false??
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
// TODO: Add the tile to tiles
tiles += tile;
}
public boolean hasTile(char tile) {
// TODO: Determine if user has the tile passed in
boolean containTile = tiles.indexOf(tile) != -1;
return true;
}
}
3 Answers

Vladut Astalos
11,246 PointsYou don't need that if statement you basically check the same thing twice. When you say return tiles.indexOf(tile) != -1;
that expression is checked for his truth value and will return whatever the result is. If that expression is considered to be true, method will return true, if is considered to be false, method will return false. You don't need to check one more time.

Craig Dennis
Treehouse TeacherSince the result of your checking code is actually a true or false...you can just
return tiles.indexOf(tile) != -1;
That make sense?

Solomon Asiedu-Ofei Jnr
1,015 PointsMakes perfect sense. Thank you

Vladut Astalos
11,246 PointsIn your method you return true all the time. You need to return the result of that statement. Since you store that result in a variable, all you need to do is to return that variable instead of true, something like this
boolean containTile = tiles.indexOf(tile) != -1;
return containTile;
or simply
return tiles.indexOf(tile) != -1;

Solomon Asiedu-Ofei Jnr
1,015 PointsOk I get that bit. So how then would I be able to return a false statement. Will this make sense
boolean containTile = tiles.indexOf(tile) != -1;
return containTile;
if (containTile == true) {
return true;
}else {
return false;
}
Solomon Asiedu-Ofei Jnr
1,015 PointsSolomon Asiedu-Ofei Jnr
1,015 PointsOOHHHH I now get. so containTile will be true or false depending on the out come of tiles.index(tiles) != -1 , so if I return containTile it will return true or false depending on the condition!!!
Thank you so much Vladut.