Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial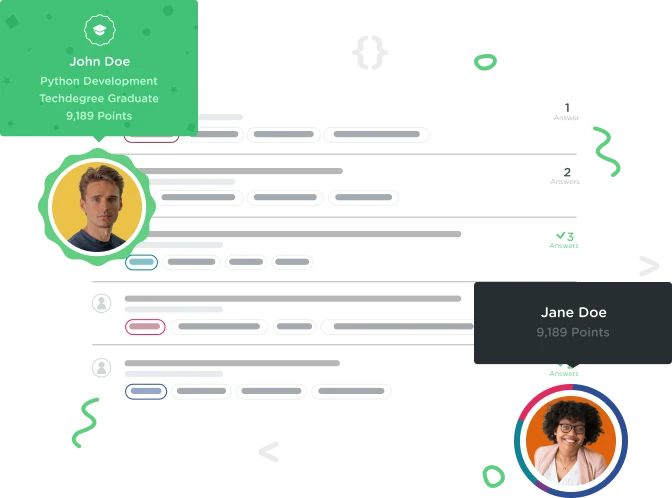

kyle madson
Full Stack JavaScript Techdegree Student 2,030 PointsHelp!!!... I couldn't add comment form to this project using DJANGO CBV...
I have been following this lessons to last and desided to work on a full plug-able article app using class based view...
Along the line i was stucked in adding comment form to the ArticleDetail please i need assistance here...
This my codes...
-------------------articles/views.py------------------
from django.contrib.auth.mixins import LoginRequiredMixin
from django.core.urlresolvers import reverse_lazy
from django.views import generic
#from . import mixins
from .models import Article, Comment
class ArticleList(generic.CreateView, generic.ListView):
fields = ("author", "title", "body", "published")
model = Article
context_object_name = 'Article'
template_name = "articles/article_list.html"
class ArticleDetail(generic.DetailView, generic.UpdateView):
fields = ("author", "title", "body", "published")
model = Article
context_object_name = 'Article'
template_name = 'articles/article_detail.html'
class ArticleCreate(LoginRequiredMixin, generic.CreateView):
fields = ("author", "title", "body", "published")
model = Article
class ArticleUpdate(LoginRequiredMixin, generic.UpdateView):
fields = ("author", "title", "body", "published")
model = Article
# Here we print out the name of the page as updated
def get_page_title(self):
obj = self.get_object()
return "Update {}".format(obj.name)
class ArticleDelete(LoginRequiredMixin, generic.DeleteView):
model = Article
success_url = reverse_lazy("articles:list")
# Here we overide the delete function to only work if a user is a superuser
def get_queryset(self):
if not self.request.user.is_superuser:
return self.model.objects.filter(author=self.request.user)
return self.model.objects.all()
---------------------artices/models.py----------------------
from django.db import models
from django.contrib.auth.models import User
from django.core.urlresolvers import reverse
from django.utils import timezone
from django.utils.text import slugify
# Create your models here.
class Article(models.Model):
author = models.ForeignKey(User, on_delete=models.CASCADE)
title = models.CharField(max_length=255)
slug = models.SlugField(max_length=255)
body = models.TextField()
created = models.DateTimeField(default=timezone.now)
published = models.DateTimeField(blank=True, null=True)
def publish(self):
self.published_date = timezone.now()
self.save()
def __str__(self):
return self.title
def get_absolute_url(self):
return reverse("articles:detail", kwargs={"slug": self.slug})
class Comment(models.Model):
article = models.ForeignKey(Article, related_name="comments")
user = models.ForeignKey(User, on_delete=models.CASCADE)
email = models.EmailField()
body = models.TextField()
created = models.DateTimeField(auto_now_add=True)
approved = models.BooleanField(default=False)
def approved(self):
self.approved = True
self.save()
def __str__(self):
return self.user
---------------------artices/admin.py----------------------
from django.contrib import admin
from .models import Article, Comment
# Register your models here.
class ArticleAdmin(admin.ModelAdmin):
list_display = ('author', 'title', 'published')
list_filter = ('author', 'created', 'published')
search_field = ('title', 'body')
prepopulated_fields = {'slug': ('title',)}
admin.site.register(Article, ArticleAdmin)
class CommentAdmin(admin.ModelAdmin):
list_display = ('user', 'email', 'body', 'approved')
admin.site.register(Comment, CommentAdmin)
---------------------artices/urls.py----------------------
from django.conf.urls import url, include
from articles import views
urlpatterns = [
url(r'^$', views.ArticleList.as_view(), name='list'),
url(r'^(?P<slug>\d+)/$', views.ArticleDetail.as_view(), name='detail'),
url(r'^create/$', views.ArticleCreate.as_view(), name='create'),
url(r'^edit/(?P<slug>\d+)/$', views.ArticleUpdate.as_view(), name='update'),
url(r'^delete/(?P<slug>\d+)/$', views.ArticleDelete.as_view(), name='delete'),
]