Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial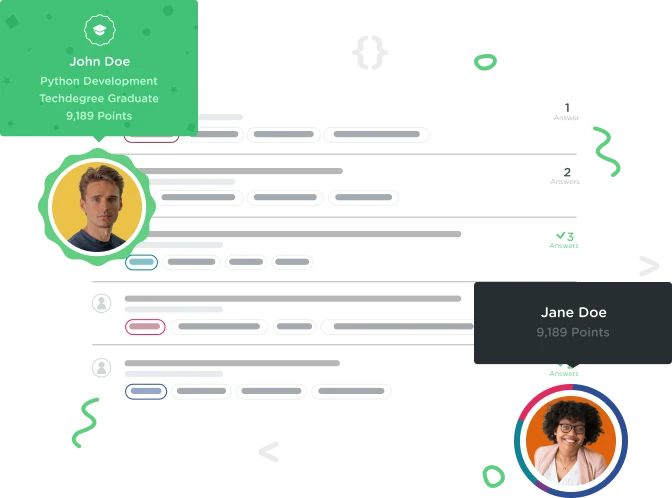

annapoff
13,552 PointsHelp! I need to finish this to complete my Java Track!
The question: Now I'd like to be able to get to the videos by their names, can you use the videosByTitle method to generate the proper map of title to video. We'll use it in the next step.
I keep getting hung up in this little block of code. I'm about to go into labor (39 weeks pregnant) and I REALLY want to finish this to complete my java track before this baby comes! The error I'm getting is that it doesn't recongnize List. List<Video> allVideosInTheCourse = course.getVideos();
Can someone please help me through this?
package com.example.model;
import java.util.List;
public class Course {
private String mName;
private List<Video> mVideos;
public Course(String name, List<Video> videos) {
mName = name;
mVideos = videos;
}
public String getName() {
return mName;
}
public List<Video> getVideos() {
return mVideos;
}
}
package com.example.model;
public class Video {
private String mTitle;
public Video(String title) {
mTitle = title;
}
public String getTitle() {
return mTitle;
}
public void setTitle(String title) {
mTitle = title;
}
}
import com.example.model.Course;
import com.example.model.Video;
import java.util.Map;
import java.util.HashMap;
public class QuickFix {
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
Video video = new Video("The Beginning Bits");
// TODO(2): Add the newly created video to the course videos as the second video.
course.getVideos().add(1, video);
}
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
}
public Map<String, Video> videosByTitle(Course course) {
Map<String, Video> videosByTitle = new HashMap<>();
List<Video> allVideosInTheCourse = course.getVideos();
for (Video video : allVideosInTheCourse) {
System.out.println(video);
}
return (Map<String, Video>) videosByTitle;
}
}
2 Answers

andren
28,558 PointsHow much help do you want? Do you just want assistance with the current error, or do you want help solving the entire task?
The reason why you get the current error is that you have not imported the List
class. If you add an import statement like this:
import java.util.List;
To the list of import statements near the top of the file then that error will go away.
Though it's worth noting that you don't really need to create a list in the first place, you can call course.getVideos()
directly when setting up the for
loop like this:
public Map<String, Video> videosByTitle(Course course) {
Map<String, Video> videosByTitle = new HashMap<>();
for (Video video : course.getVideos()) {
System.out.println(video);
}
return (Map<String, Video>) videosByTitle;
}
And it will have the same effect as using the list you made.
Your code still won't fulfill the task after that fix, but in case you want to continue working on it on your own I won't solve it for you in this post.
If you want more assistance then just reply and I'll answer any question you have.

Yanuar Prakoso
15,196 PointsHi Anna,
Just like what andren said you do not need the List because it is already part of the course class that was used as one of argument in the method. In addition you do not need to print out the video title and video relation since the task is only to Map the video title to the video. Thus you only need a HashMap for that and you already made a HashMap called videosByTitle. Therefore, you just use that to create a Map using the put() method like this:
public Map<String, Video> videosByTitle(Course course) {
Map<String, Video> videosByTitle = new HashMap<>();
for (Video video : course.getVideos()){
videosByTitle.put(video.getTitle(), video); //this to map the title as key and video as value
}
return videosByTitle;
This code will get you over the 2nd task of the challenge. I hope this will help
annapoff
13,552 Pointsannapoff
13,552 PointsI need help solving the task please! I've spent several hours on this (sad, i know)
andren
28,558 Pointsandren
28,558 PointsAlright that's fine. Your code is not actually that far off from the solution.
The task wants a map where the keys are titles of videos and the value is the
Video
object that corresponds to that title. TheVideo
object has a getter calledgetTitle
which can be used to get its title.Here is the solution to this task with some comments added:
There is a third task to this challenge, I'd recommend you try to solve it yourself first, but if you can't solve it then I can give you the solution to that too.