Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial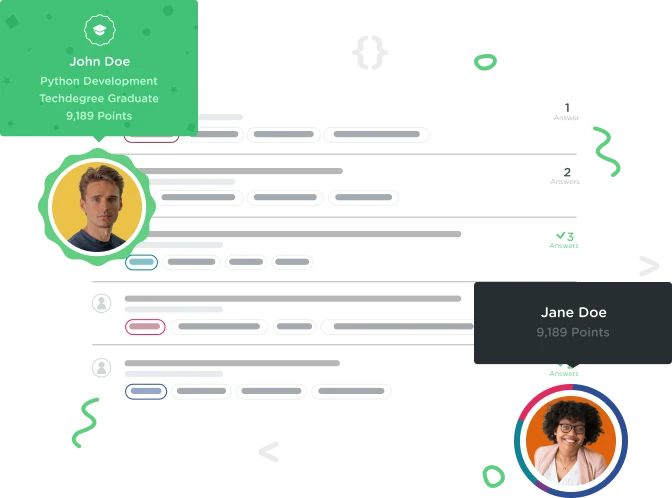
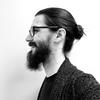
Joshua Rystedt
4,086 PointsHelp improve my code
Anyone have some ideas on how to improve my solution to this assignment? I really enjoyed this one and am proud of my work but I am curious what suggestions and tips you guys may have.
/*
Assignment
Imagine that you are asked to build a todo app that keeps track of tasks. A task is broken down into the following:
Description
Status: Doing, Pending, Completing
The Description of the task describes what needs to get done. The Status on the other hand can and should have only 3 values: Doing, Pending, Completed. Your assignment is to model a Task struct which will have two properties description and status. However, here's the catch to use Status you will have to create your own enum.
*/
struct Task {
var description : String
enum Status {
case Doing,
Pending,
Completed
init() {
self = .Pending
}
}
var status = Status()
init(description: String) {
self.description = description
}
func printStatus() {
let status = self.status
if status == Task.Status.Completed {
println("This task has been completed")
} else if status == Task.Status.Doing {
println("This task is being done")
} else if status == Task.Status.Pending {
println("This task is pending")
} else {
println("Error! I don't know what the status is.")
}
}
}
var assignment = Task(description: "Build a task manager")
assignment.printStatus()
assignment.status = .Doing
assignment.printStatus()
assignment.status = .Completed
assignment.printStatus()
Note: my reasoning for placing the Status enum within the struct was because I do not intend (nor see a use for) accessing Status independent of Task.
1 Answer

Enrique Munguía
14,311 PointsYour code looks good, the reasoning of the Enum within the Struct is correct given that other parts of the application won't use Status alone. One improvement I'd make is convert the if-else if-else structure of the function printStatus to switch statement, it'll make your code more readable, and under some circumnstances may have better performance.
func printStatus() {
switch self.status {
case .Completed:
println("This task has been completed")
case .Doing:
println("This task is being done")
case .Pending:
println("This task is pending")
default:
println("Error! I don't know what the status is.")
}
}
Joshua Rystedt
4,086 PointsJoshua Rystedt
4,086 PointsThank you, Enrique! That is an excellent suggestion!
Noel Deles
8,215 PointsNoel Deles
8,215 PointsI tried implementing the switch statement, and my output in playground is
{description "Learn Swift", (Enum Value)}
after having called it via
task.printStatus()
Isn't it only supposed to output either of hte 3 strings contained within the switch statements??