Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial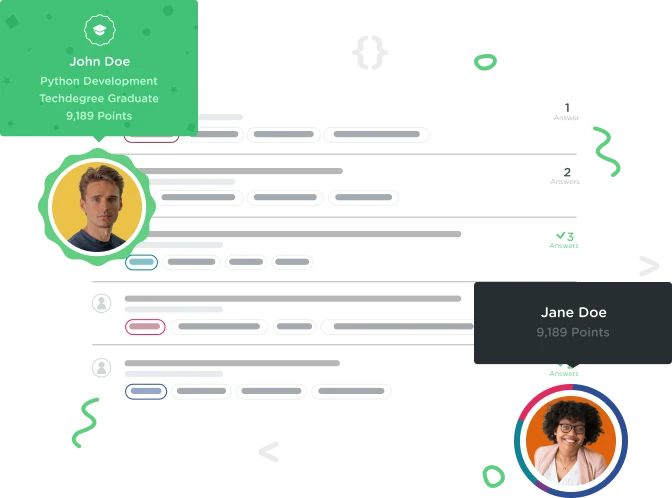

Tojo Alex
Courses Plus Student 13,331 PointsHelp in task 3 of 3
I am completely lost of what to do.
public class MainActivity extends AppCompatActivity implements NewsFragment.NewsListener {
// New tags for referring to fragments
public static final String NEWS_FRAGMENT = "news_fragment";
public static final String NEWSITEM_FRAGMENT = "newsitem_fragment";
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
FragmentManager fragmentManager = getFragmentManager();
NewsFragment savedFragment = (NewsFragment) fragmentManager.findFragmentById(R.id.placeholder);
if (savedFragment == null) {
NewsFragment newsFragment = new NewsFragment();
FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction();
fragmentTransaction.add(R.id.placeholder, newsFragment);
fragmentTransaction.commit();
}
}
@Override
public void onNewsItemSelected(int index) {
NewsItemFragment fragment = new NewsItemFragment();
// TODO: how do we pass arguments to the new Fragment?
Bundle bundle = new Bundle ();
bundle.putInt(NewsItemFragment.KEY_NEWS_ITEM_INDEX,index);
fragment.setArguments(bundle);
FragmentManager fragmentManager = getFragmentManager();
FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction();
fragmentTransaction.replace(R.id.placeholder, fragment);
fragmentTransaction.addToBackStack(null);
fragmentTransaction.commit();
}
}
public class NewsItemFragment extends Fragment {
public static final String KEY_NEWS_ITEM_INDEX = "news_item_index";
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
// TODO: Hmmm, how do we get this index?
int index = getArguments().getInt(NewsItemFragment.KEY_NEWS_ITEM_INDEX);
getActivity().setTitle(News.headlines[index]);
View view = inflater.inflate(R.layout.fragment_newsitem, container, false);
return view;
}
@Override
public void onStop() {
super.onStop();
getActivity().setTitle(getResources().getString(R.string.app_name));
}
}
public class News {
public static String[] headlines = new String[]{
"Treehouse student develops #1 App in Google App Store",
"Oracle and Google decide to hug it out",
"Java8 coming to Android! Is it too late?"
};
}