Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial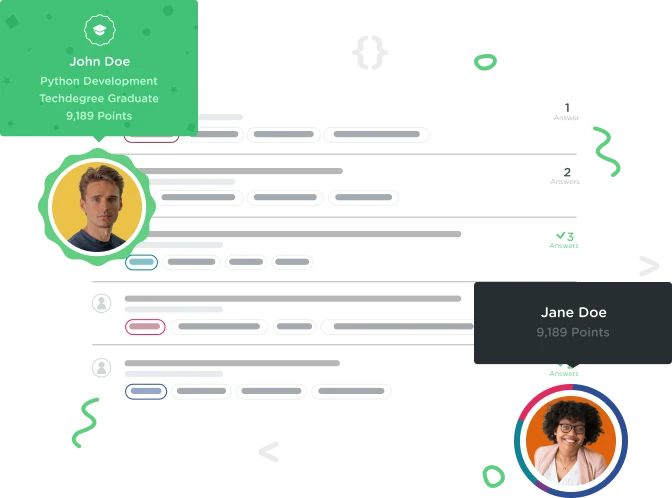

Avinash Pandit
6,623 PointsHelp in understanding this session
Can someone please explain what the following lines do:
a) var http = require("http"); In this what is variable http? It looks like an object the way it is used later on, but then shouldn't the declaration be something like var http = new require("http") In the same vein, is require a constructor function?
b) var newVariable = http.get("http://link", function(response) { console.log("text"); }); In this how is console.log running when we execute app.js?
1 Answer

Ognjen Jevremovic
13,028 PointsHello Avinash, how are you?
As someone who's still learning both the JavaScript and Node.js, here's how I understood and explain those questions to myself.
The http
variable that we're making, has a value defined being the function call and we're providing it with an argument - the "http" string.
Now if you actualy log out the content of the http variable to the console, you'll get an object literal containing the information about the request; like the methods, outgoing messages, all the possible statusCode outcomes, etc.
I understand the confusion for the need of new
keyword, we use in the prototypal inheritance (prototype-based programming, outlined as Object Oriented JavaScript) to actualy make a new instance of an object created through the constructor function. But I think that the require()
is not an actual constructor function per se, but rather it's the function defined in the Node application itself that we're only calling and providing with a string argument of http.
To try and answer the second question, I'd use a simple example. When we define a function, the function itself is not actualy run (unless we defined a self invoking function on the first place), but rather we just defined it and made it available to us for the later use (whenever we feel the need for that function) which we can then call or execute by simply typing the function name, followed with paranthesis - aka the function call. However, when we define a variable (unless the variable is a function expression, that is, if we define an anonymous function as a value of the variable, we "can't" run it) it's actually there; it does not need to be run, it's defined. Simple example would be:
var studentName = 'Avinash';
/ === /
function printStudent( studentName ) {
console.log('The aspiring web developer ' + studentName);
}
var student1 = printStudent( 'Avinash' );
See the pattern. If we would to run this code, the function would run, because we actualy called for it (by being a bit sneeaky and providing the function call as a value of a variable). I know it looks really simple, but the actual problem is complicated only as much as we actualy made it look = ).
newVariable = http.get('http://link', function(response) {
console.log('some text');
});
is run "imidiately", because the variable's value is the function call (just in the example I outlined above). The variable's value is the call for a function to run. To explain it even further, here's just another example of the previous code:
function printStudent( studentName ) {
return 'The aspiring web developer ' + studentName + '.';
}
var student1 = printStudent( 'Avinash' );
The only example I'd want to use this function call as a value of a variable would be if I wanted to, perhaps, print additional information for each student. We only did call the function like that, because we wanted to store it's value returned in a variable.
So if we run this in the Node, the value of student1
variable would be 'The aspiring web developer Avinash.'.
I hope that makes sense?
And I hope I didn't make the post too long and introduced some more confusion with it. I really tried to approach the problem on a simple matter. Or at least, that's how I realised the code behind it.
Hope that helps! = ) Cheers
Nattapol Kamolbal
15,528 PointsNattapol Kamolbal
15,528 PointsGreat answer. You help me understand this issue. Thank you very much. :)