Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial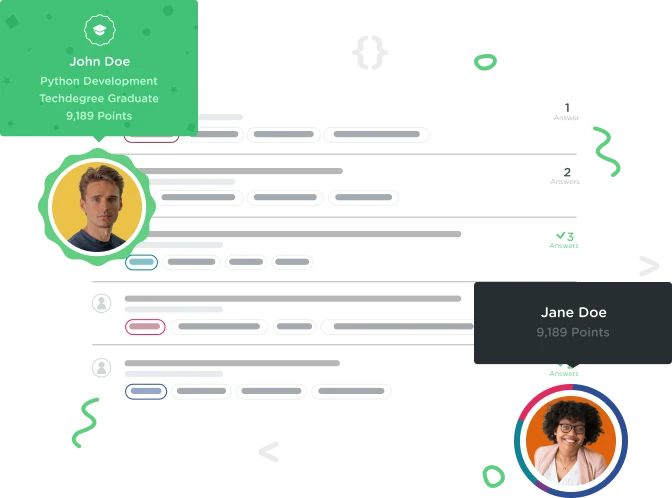
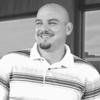
Ryan Breece
4,160 PointsHelp Initializing Tag Struct inside of Post Struct. What am I doing Wrong?
We learned about instance methods before. For this task, add an instance method to Post.
Name the method description. It takes no parameters and returns a string that describes the post instance. For example given a title: "iOS Development", author: "Apple", and a tag named "swift", the description would read as follows"
"iOSDevelopment by Apple. Filed under swift"
Once you have an instance method, call it on the firstPost instance and assign the result to a constant named postDescription
struct Tag {
let name: String
}
struct Post{
let title: String
let author: String
let tag: Tag
init(title: String, author: String, tag: String){
self.title = title
self.author = author
self.tag = Tag(name: tag) }
func description() ->String {
return (" \(title) by \(author). Filled under \(tag) ")
}
}
let firstPost = Post(title: "iOS Development", author: "Apple", tag: "Swift")
let postDescription = firstPost.description()
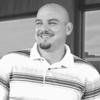
Ryan Breece
4,160 PointsThank you Juan.
12 Answers
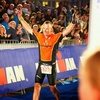
Steve Hunter
57,712 PointsHi Ryan,
You don't need the create an init
function. The code would look like:
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
func description() -> String{
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let firstPost = Post(title: "Treehouse blog", author: "S. Hunter", tag: Tag(name: "Swift, iOS"))
let postDescription = firstPost.description()
I like your code, that's a nice idea. But I don't think you can create the Tag
like that. It isn't a string, so you have to pass a completed Tag
instance into the Post
.
And, as Juan has said, the name of the Tag
instance needs accessing with dot notation in your interpolated string returned from description
.
I hope that helps.
Steve.
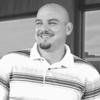
Ryan Breece
4,160 PointsMan Thank you! I was getting the error "Tag is not String", but I couldn't figure it our. This makes sense I needed to pass a completed Tag instance into the the Post. I have learned a ton from treehouse! Thanks Again.

Austin Money
2,304 PointsCorrect me if i'm wrong, but i believe the title, author, and tag should all be variables and not constants... see if that helps.
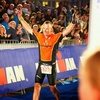
Steve Hunter
57,712 PointsHi Ali,
The first task is to create a new struct
called Post
which has three stored properties, title
and author
are both of type String
and the stored property tag
is of type Tag
which is a struct
that is already defined for us. The Tag
struct has one stored property, a String
called name
.
So, the Post
struct would look like:
struct Post{
let title: String
let author: String
let tag: Tag
}
That completes the struct
. The next part of the challenge says, create an instance of Post and assign it to a constant named firstPost. Constants are created using the keyword let
and it needs to be called firstPost
. That starts:
let firstPost =
Now we need to create the new instance of a Post
. It takes two strings and a Tag
. Let's start with the strings - they can be anything. the first is called title
and the second is author
:
let firstPost = Post(title: "A title", author: "An author" ...)
Lastly, it needs a Tag
instance passing in. A Tag
requires a name
stored property to be created, which would look like:
let aTag = Tag(name: "A name")
But we're doing this inside the Post
creation call. So we can just pass in the part to the right of the equals sign into our incomplete Post
creation:
let firstPost = Post(title: "A title", author: "An author", tag: Tag(name: "A name"))
And that's it! I'll update this with the second part of the challenge in a minute.
Steve.
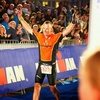
Steve Hunter
57,712 PointsThe second task requires a method adding to the struct. It is called description
. It takes no parameters and returns a string. Inside, it just takes the three stored properties and interpolates them into a string which it returns.
So, the method skeleton looks like:
func description() -> String {
}
Inside, gather the stored properties into a string like "\(title) by \(author). Filed under \(tag.name)"
. The last one needs a little thought as you can't interpolate the tag
; you want to use its name
property. Add that string inside the method and return
it:
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
Then you just create a constant and call the method on the firstPost
instance:
let postDescription = firstPost.description()
And that's it
Steve.
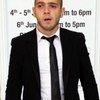
Giorgi Gulua
5,956 PointsWorks pretty fine in playgrounds but still can't pass the challenge
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
func description() -> String {
return "\(title) by \(author). filed under \(tag.name)"
}
}
let firstPost = Post(title: "SomeTitle", author: "Some Guy", tag: Tag(name: "TAGTAG")).description()
let postDescription = firstPost
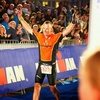
Steve Hunter
57,712 PointsHi there,
Your firstPost
constant holds a string, not a Post
because you have created a Post
then called .description()
on that post, which returns a string.
For firstPost
to hold a Post
remove the call to description
:
let firstPost = Post(title: "SomeTitle", author: "Some Guy", tag: Tag(name: "TAGTAG"))
Then to get the description for your postDescription
constant, call description
on firstPost
:
let postDescription = firstPost.description()
The challenge is expecting firstPost
to hold an instance of Post
but that's not what it's getting so the challenge is, correctly, failing.
Make sense?
Steve.
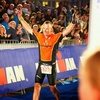
Steve Hunter
57,712 PointsHi Fiodar,
You need to pull out the name
part of the Tag
; you can't just put the Tag
instance in there. So, in your description
method, use tag.name
rather than just tag
.
Make sense?
Steve.

Fiodar Shkolnikau
3,426 Pointsstruct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
func description() -> String {
return "⧵(title) by ⧵(author). Filed under ⧵(tag)"
}
}
let firstPost = Post(title: "ios Development", author: "Apple", tag: Tag(name: "Swift"))
let postDescription = firstPost.description()
Something iam missing out? Keep getting an error.
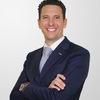
Mitchell Henderson
iOS Development Techdegree Student 4,058 PointsFiodar: You misspelled "iOSDevelopment"
let firstPost = Post(title: "iOSDevelopment", author: "Apple", tag: Tag(name: "swift"))
[MOD: edited code block - srh]
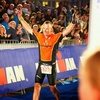
Steve Hunter
57,712 PointsHi Mitchell,
The strings used by way of example within the challenge are just that, examples. You can use whatever values you want as long as the name
element of the Tag
instance gets assigned into postDescription
, the challenge will pass. This works fine:
let firstPost = Post(title: "Title", author: "S Hunter", tag: Tag(name: "a tag"))
let postDescription = firstPost.description()
Make sense?
Steve.
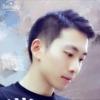
JUN MENG
10,249 Pointsstruct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
init(title: String, author: String, tag: String){
self.title = title
self.author = author
self.tag = Tag(name: tag)
}
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let firstPost = Post(title: "Development", author: "Apple", tag: "swift")
let postDescription = firstPost.description()
// I keep getting an error too
[MOD: edited code block - srh]
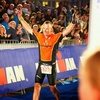
Steve Hunter
57,712 PointsHi Jun,
You are passing a String
into the init
method then creating a Tag
instance inside that method. The challenge is expecting to be able to pass a Tag
instance into the struct
to create the instance.
First, delete the init
method; you don't need it here. Then, where you create firstPost
, pass a new Tag
instance in, rather than a String
. Something like:
let firstPost = Post(title: "A title", author: "An author", tag: Tag(name: "aTag"))
Let me know how you get on.
Steve.

ali aboutaleb
4,025 PointsSteve i cant figure it out ive been trying... can you post the whole code and briefly explain it??
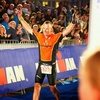
Steve Hunter
57,712 PointsGive me a second - and do you have the link to the current challenge? This one has been retired as it is covering an old Swift version.

ali aboutaleb
4,025 PointsThank you steve. I dont know why its not compiling though.... do think its a problem in the compiler?
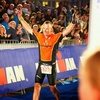
Steve Hunter
57,712 PointsThe challenges seem to be working here. Can you post your code?
Steve.

ali aboutaleb
4,025 Pointsstruct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
}
let aTag = Tag(name: "A name")
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
let firstPost = Post(title: "iOS Development", author: "Apple", tag: Tag(name: "swift"))
let postDescription = firstPost.description()
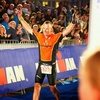
Steve Hunter
57,712 PointsOK - you need to delete the let aTag
line - that was just an example in my description, not part of this challenge.
Next, move the whole func
inside the struct
- that code is part of the Post
struct. On a style point, consider your indents - having correctly indented code makes it much easier to read. Indeed, some languages require indents for the code to work, e.g. Python.
The rest looks fine.
Steve.

ali aboutaleb
4,025 PointsThanks steve your the best!
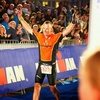
Steve Hunter
57,712 Points

Tafadzwa Nyazorwe
17,053 Points//you can try this one, it works
struct Tag { let name: String } struct Post { let title: String let author: String let tag: Tag func description() -> String { return "(title) by (author). Filed under (tag.name)" } } let firstPost = Post(title: "My First iOS App", author: "Rick Hanolt", tag: Tag(name: "Swift")) let postDescription = firstPost.description()
villa
6,171 Pointsvilla
6,171 PointsYou have tu call the name of the Tag. and on the init tag: is not type String . so first tag: Tag self.tag = tag and on your return value '''return "(title) by (author). Filed under (tag.name)'''