Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial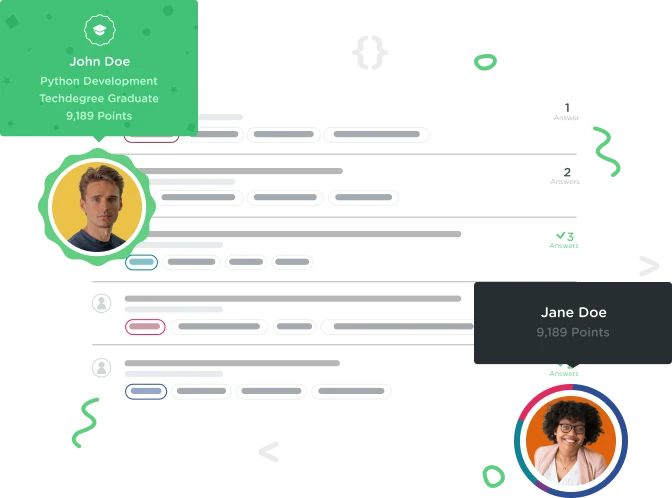
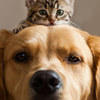
Devin Scheu
66,191 PointsHelp! (Java)
Question: Finally, uncomment the addPost method in Forum.java. Then for the last task, uncomment the main method in Example.java fixing the bugs as you go to use the new working code. For the forum post title and description, use that to shout out about how it feels to complete this challenge.
PS. You're amazing.
Code:
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public User getAuthor() {
return mAuthor;
}
public ForumPost(User author, String title, String description) {
mAuthor = author;
mTitle = title;
mDescription = description;
}
public String getDescription() {
return mDescription;
}
public String getTitle() {
return mTitle;
}
// TODO: We need to expose the description
}
public class User {
private String mFirstName;
private String mLastName;
public User(String firstName, String lastName) {
// TODO: Set the private fields here
mFirstName = firstName;
mLastName = lastName;
}
public String getFirstName(){
return mFirstName;
}
public String getLastName(){
return mLastName;
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public Forum(String topic) {
mTopic = topic;
}
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
// Forum forum = new Forum("Java");
// Take the first two elements passed args
// User author = new User();
// Add the author, title and description
// ForumPost post = new ForumPost();
// forum.addPost(post);
}
}
4 Answers
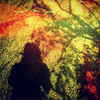
Kristen Law
16,244 PointsHey Devin! Looks like you did everything up to the point where you have to uncomment part of the main
method in Example.java
.
What you need to do is uncomment the lines that contain code, and make sure that the code is correct. This part of the challenge is basically making sure you know how to use constructors.
The first one, for example
Forum forum = new Forum("Java");
is correct, because if you take a look at Forum.java
, you can see that the Forum
constructor only takes one parameter, a string, and that is exactly what was passed in.
The User
and ForumPost
constructor calls in the next few lines will require some modifications. Take a look at the User.java
and ForumPost.java
files to see what you need to pass into their constructors to make it work.
Hope that clears it up! Feel free to ask if you need further clarification.
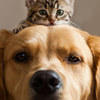
Devin Scheu
66,191 PointsIm still not getting it.
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
User author = new User("devin", "scheu");
ForumPost post = new ForumPost(Devin, "Bomb", "boom!");
forum.addPost(post);
}
}
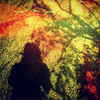
Kristen Law
16,244 PointsYou're close! For the User
, it wants you to pass in the first two elements in String[] args
. These elements can be accessed with args[0]
and args[1]
.
For the ForumPost
, the first parameter that you need to pass in should be a User
. You defined a User
in the line right above, so you can just pass in that variable.
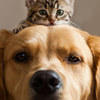
Devin Scheu
66,191 PointsSo how would the args be properly passed in, because my current code now is:
Forum forum = new Forum("Java");
User author = new User(args[0], args[1]);
ForumPost post = new ForumPost(author, "Finished" "Awesome!");
forum.addPost(post);
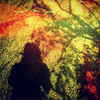
Kristen Law
16,244 PointsLooks good! I believe you forgot a comma between "Finished" and "Awesome!".
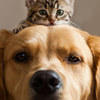
Devin Scheu
66,191 PointsThanks for the help Kristen! Much thanks!
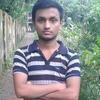
Niyamat Almass
8,176 PointsHey Devin Scheu .I am stuck same to you please tell me how you pass the challenge

supniger
4,511 PointsThx, helped alot.