Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial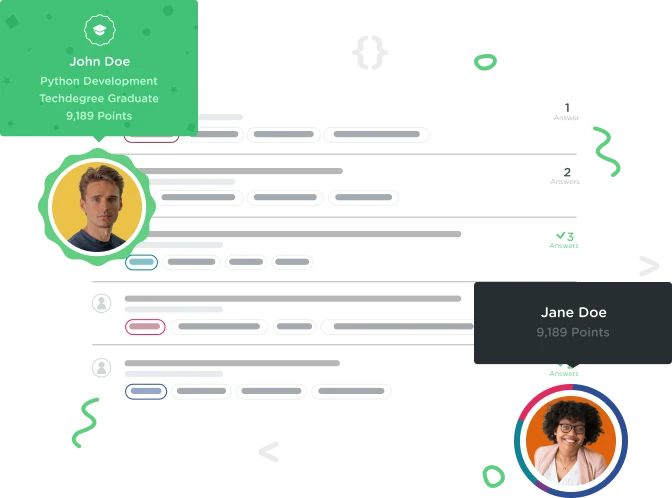
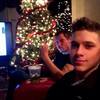
Andrew McCombs
4,309 PointsHelp - Manipulation An Array - Challenge
Challenge task 1 of 1 This block of code creates an array of ice cream flavors and displays them in ascending order. The owner of the ice cream shop would like this order changed so that the flavors are displayed in descending order instead, starting with Cookie Dough first and Jalapeno So Spicy last. Leave the $flavors array itself in the same order, but modify something else in this code block to achieve that.
Can someone please tell me what I did wrong. If I run a var_dump on the $reverse is shows the items descending order. I dont understand when I echo out the $reverse it shows 6 Arrays in a UL LI.
<html>
<body>
<?php
$flavors = array(
"Jalapeno So Spicy",
"Avocado Chocolate",
"Peppermint",
"Vanilla",
"Cake Batter",
"Cookie Dough"
);
$reverse = array_reverse($flavors);
?>
<ul>
<?php
$list_html = "";
foreach($flavors as $flavor) {
$list_html = $list_html . "<li>";
$list_html = $list_html . $reverse;
$list_html = $list_html . "</li>";
}
echo $list_html;
?>
</ul>
</body>
</html>
5 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Andrew,
You want to keep the working variable $flavor as is inside the foreach loop.
You only need to reference the $reverse variable instead of $flavors
foreach($reverse as $flavor) {
$list_html = $list_html . "<li>";
$list_html = $list_html . $flavor;
$list_html = $list_html . "</li>";
}

Petros Sordinas
16,181 PointsYour foreach() loop is referencing $flavors, but the reversed array has been stored in $reverse.
Try replacing
$reverse = array_reverse($flavors);
with $flavors = array_reverse($flavors);
and
$list_html = $list_html . $reverse;
with $list_html = $list_html . $flavor;
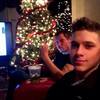
Andrew McCombs
4,309 PointsBummer! It looks like the flavors array has been reversed. You are using the right function, but you need to leave the flavors array itself unmodified. Try creating another variable like $flavors_reversed.
I attempted the $flavors_reversed but then I got this
Bummer! Something is not quite right in the output. Try using a native PHP function to reverse the array.

Jason Anello
Courses Plus Student 94,610 PointsYou can't modify the $flavors array.

Petros Sordinas
16,181 PointsIf you have to keep the flavours array unmodified, then try the following (change the foreach and the string):
<body>
<?php
$flavors = array(
"Jalapeno So Spicy",
"Avocado Chocolate",
"Peppermint",
"Vanilla",
"Cake Batter",
"Cookie Dough"
);
$reverse = array_reverse($flavors);
?>
<ul>
<?php
$list_html = "";
foreach($reverse as $reverse_element) {
$list_html = $list_html . "<li>";
$list_html = $list_html . $reverse_element;
$list_html = $list_html . "</li>";
}
echo $list_html;
?>
</ul>
</body>
</html>```

Petros Sordinas
16,181 PointsAh, just saw Jason's reply... even better
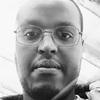
Christophe Kwizera
4,044 Pointsmine doesn t even load the code challenge
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsKeep this line:
$reverse = array_reverse($flavors);
it's correct