Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial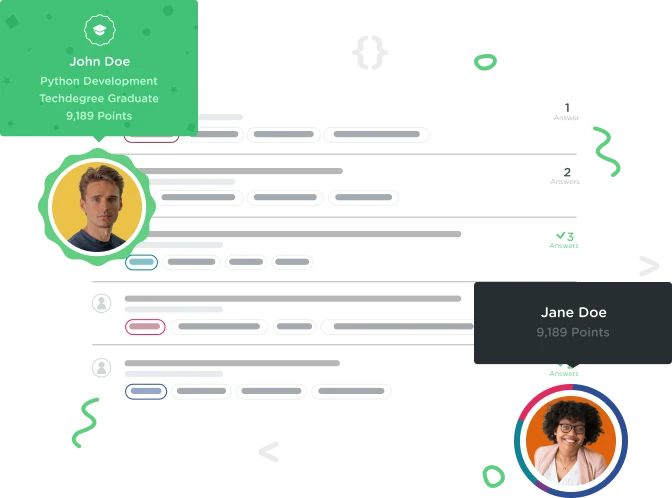
3 Answers

jinhwa yoo
10,042 Points}
// Part 1: order the blog post's creation date chronologically, oldest first.
return mCreationDate.compareTo(blogpost.mCreationDate); //-------------> what does this mean to be more specific...???
}
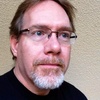
Chris Freeman
Treehouse Moderator 68,426 PointsTask 1 asks: Let's allow our BlogPost to be sorted. In this first step, just make the class implement Comparable interface, and produce a compareTo method that accepts an object as a parameter. Have it always return 1 for this first step, we'll do more in the next step.
Part 1: make the class implement Comparable interface. This is done by adding the key word Comparable
to the class signature:
public class BlogPost implements Comparable {
// ...
}
Part 2: produce a compareTo method that accepts an object as a parameter. Have it always return 1. So the method will be public, return an int, be named compareTo, and accept an Object obj
as a parameter:
public int compareTo(Object obj) {
return 1
}
Task 2 asks: In this step, cast the Object passed to the compareTo method to a com.example.BlogPost. Check for equality first, if they are equal return 0. Leave the default return of 1 in place for this step.
public int compareTo(Object obj) {
// Part 1: Cast the Object passed to the compareTo method to a com.example.BlogPost.
BlogPost blogpost = (BlogPost) obj;
// Part 2: Check for equality first, if they are equal return 0.
if (equals(blogpost)) {
return 0;
}
// Part 3: Leave the default return of 1 in place
return 1
}
Task 3 asks: Alright, now let's order the blog post's creation date chronologically, oldest first.
public int compareTo(Object obj) {
BlogPost blogpost = (BlogPost) obj;
if (equals(blogpost)) {
return 0;
}
// Part 1: order the blog post's creation date chronologically, oldest first.
return mCreationDate.compareTo(blogpost.mCreationDate);
}
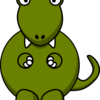
luis martinez
2,480 PointsSo what i did before i checked this comment for help was
public int compareTo(Object obj) {
if (equals(obj))
{
return 0;
}
return mCreationDate.compareTo(obj.mCreationDate);
}
//Why doesn't this work. I am still trying to understand why you would Object obj in the first place. Is it like a copy of the BlogPost? If you could answer my question it would be very helpful. Thank you.
[MOD: added ```java markdown formatting -cf]]
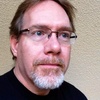
Chris Freeman
Treehouse Moderator 68,426 PointsLuis, the compareTo()
method only works on like objects, so the obj
argument needs to be cast as this type of BlogPost object before the comparison. Adding the casting line fixes your solution.

Gavin Ralston
28,770 PointsHere's maybe another way to look at what's happening with a simplified example. You can compile it and run it.
Just remember "something.compareTo(somethingElse)" can be read aloud like this:
"Is something greater than, equal to, or less than something else?"
ToughGuy.java
public class ToughGuy implements Comparable {
Integer mStrength;
public ToughGuy(int strength) {
mStrength = strength;
}
public Integer getStrength() {
return mStrength;
}
@Override
public int compareTo(Object o) {
ToughGuy opponent = (ToughGuy) o;
return mStrength.compareTo(opponent.getStrength());
}
}
Main.java
public class Main {
public static void main(String[] args) {
ToughGuy guy1 = new ToughGuy(1);
ToughGuy guy2 = new ToughGuy(10);
System.out.println("Negative means less, Positive means greater, Zero means equal");
System.out.print("Tuffy One compared to Tuffy Two: ");
System.out.println(guy1.compareTo(guy2));
System.out.print("Tuffy One compared to himself: ");
System.out.println(guy1.compareTo(guy1));
System.out.print("Tuffy Two compared to Tuffy One: ");
System.out.println(guy2.compareTo(guy1));
}
}
Output
Negative means less, Positive means greater, Zero means equal
Tuffy One compared to Tuffy Two: -1
Tuffy One compared to himself: 0
Tuffy Two compared to Tuffy One: 1
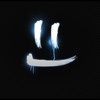
Grigorij Schleifer
10,365 PointsVery cool example !!!!
Gavin Ralston
28,770 PointsGavin Ralston
28,770 PointsRemember that mCreationDate is a Date, and it's a member of your BlogPost class.
And getCreationDate() returns a date from the other BlogPost object, so you're comparing two dates.
So looking at it as if we were the object, we'd think of the 'mCreationDate' as "My Creation Date" and it may help to read it aloud like that.
"Return whether or not My Creation Date is newer (greater) or older (less) than Other Blog Posts Creation Date"
Now pretend, for simplicity's sake, that each method call returns something that is maybe more intuitive, like "today" and "yesterday" for dates. This is kind of how it breaks down when it is run.
So now when you take a BlogPost and call compareTo() on another BlogPost, it knows by what criteria you want to compare them. In this case, newer blog posts are "greater" than older blog posts. Now you have a way to sort them based on age.
Chris Freeman
Treehouse Moderator 68,426 PointsChris Freeman
Treehouse Moderator 68,426 PointsThere are two comparisons going on. At the class level we have defined a
compareTo()
method (and added the implements Comparable) so that two *BlogPost*s may be compared. Inside this method we are free to determine how this comparison is made.Inside our comparison method, we chose is to use the member field
mCreationDate
for the basis of our comparison . This field is type Date which has it's own method of comparing itself to other Data objects also calledcompareTo()
.So the line:
return mCreationDate.compareTo(blogpost.mCreationDate);
says, "When comparing two
BlogPost
s, use the member fieldmCreationDate
as the basis by comparing the Date value to the corresponding Date value on the other blogpost using the Date fieldcompareTo()
method."luis martinez
2,480 Pointsluis martinez
2,480 PointsThank you. This helped clear somethings out.