Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial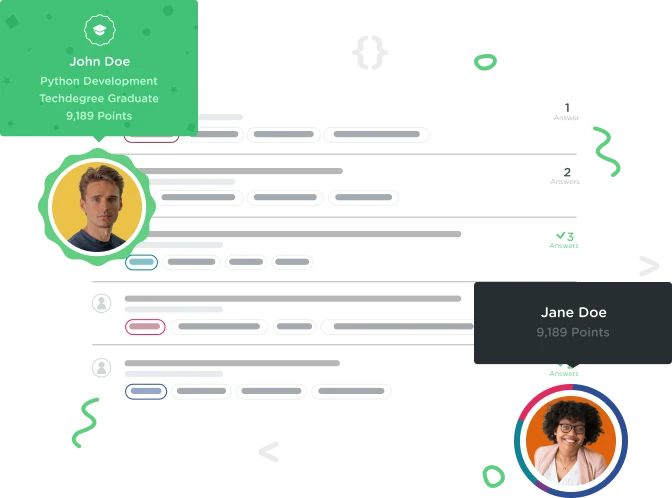
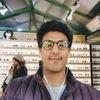
Shreemangal Sethi
Full Stack JavaScript Techdegree Student 15,552 PointsHelp me clearly understand the While loop.
In the the 2nd line of the code we create a variable named randomNumber and assign that to getRandomNumber(upper), which is also the name of function after the variables. When we create the while loop, why do we assign 'var guess = getRandomNumber' ? I understand that we need to run the while loop till it fulfills the condition where 'guess' is equal to 'getRandomNumber(upper)'. Does that also mean that we can also write a the program where we equate 'guess' to 'randomNumber' and not 'getRandomNumber' as in the 2nd line of the code we assigned getRandomNumber to variable randomNumber?
So shouldn't we be equating the guess and getRandomNumber(upper) using the '===' instead of the assignment operator (=)?
var upper = 10000 var randomNumber = getRandomNumber(upper) var guess; var attempts = 0;
function getRandomNumber(upper){ return math.floor(math.random() x upper) + 1; }
while(guess!==randomNumber){ guess = getRandomNumber(upper); attempts +=1; }
3 Answers

andren
28,558 PointsIt sounds like you are a bit confused about what the loop is actually doing, the condition of the loop is not that guess
should equal getRandomNumber(upper),
the condition is this part of the code: guess !== randomNumber
which is a condition that states that the loop will run as long as guess
is not equal to randomNumber
.
This line inside of the loop: guess = getRandomNumber(upper)
is setting guess
equal to a number generated by the getRandomNumber
function, not comparing them. That's why the =
operator is used instead of the ===
operator.
Maybe it will make more sense if I break down the code a bit. A key thing to keep in mind for this code example is that when you assign a function call to a variable you are assigning the value the function returns, not the function itself. Meaning that in the case of randomNumber = getRandomNumber(upper)
, randomNumber
gets set to whatever specific number that getRandomNumber
generated during that run.
So referencing randomNumber
and referencing getRandomNumber(upper)
is not the same. Every time you reference the randomNumber
variable it still has the same number in it that was assigned at the start, it does not change over time. Every time you call getRandomNumber(upper)
it generates a new random number.
The way the code works is that at the start randomNumber
is assigned a specific random number (that does not change after that point), then within the loop the guess
variable is assigned a number generated by getRandomNumber(upper)
. That function call will generate a new random number each time. So each time the loop runs guess
will be assigned a new number.
The goal of the loop is to see how many iterations it takes before guess
ends up being assigned the same number that randomNumber
was assigned at the start of the program.
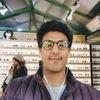
Shreemangal Sethi
Full Stack JavaScript Techdegree Student 15,552 PointsThanks Andren, this really clarifies a lot of things. I wrote the code again and what you said clearly makes sense. But i just want to understand one more thing is that when in the loop we are setting a condition and using the "not equal to" operator for (guess !== randomNumber) can we also rewrite it as (guess !==getRandomNumber(upper)). Would this mean the same thing as in the loop we want it to keep executing till "guess = getRandomNumber(upper)".
Thanks

andren
28,558 PointsNo it would not mean the same thing. When guess = getRandomNumber(upper)
is ran guess
is set equal to a random number that getRandomNumber(upper)
generates, the point of the comparison is to see if that number is equal to the random number that randomNumber
was assigned at the start.
If you compare guess
(which is assigned a new random number each loop) to getRandomNumber(upper)
(which generates a new random number each time it is called) then both of the numbers being compared will change each time the loop runs. Which reduces the chances they will match.
Essentially with the normal code randomNumber
is assigned a random number, let's say it is assigned 8. Then within the loop guess
will be assigned a random number, let's say it's 6. The loop will check if 6 is not equal to 8 (Which is true
) so the loop will run again. Let's say that this time guess
is assigned 8. The loop will check if 8 is not equal to 8 (Which is false
) so the loop ends.
With the code that you propose the randomNumber
is assigned a random number which is then never used. Then within the loop guess
will be assigned a random number, let's say it's 5. The loop will check if 5 is not equal to some random number let's say 2 (Which is true
) so the loop will run again. Let's say that this time guess
is assigned 2. The loop will check if 2 is not equal to some new random number let's say 5 (Which is true
) so the loop will run again. Eventually both of the getRandomNumber(upper)
calls would generate the same number and the loop would end, but that is a rarer scenario than with the first code where the number being compared against remains static.
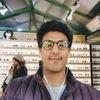
Shreemangal Sethi
Full Stack JavaScript Techdegree Student 15,552 PointsThanks! Andren completely understand it now. Thanks for taking effort to explain it extensively. Just loving it :)

Immanuel Jaeggi
5,164 PointsWell explained!