Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial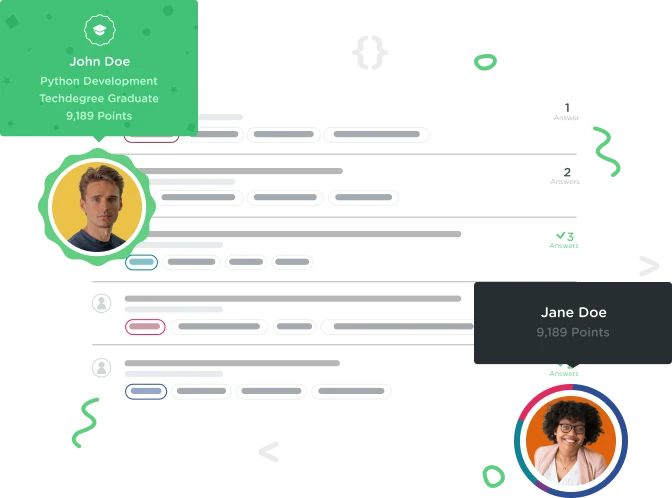

maor baruch
747 Pointshelp me i stuck java objects
i stuck in java objects i dont know what to do please help
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public int getCountOfLetter(char Letter) {
for(char letter : tiles.toCharArray()) {
if(letter == Letter) {
letter++;
System.out.println("they are the same");
}
}
return Letter;
}
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
2 Answers
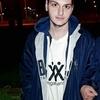
Tornike Shelia
2,781 PointsSo, I'll try to explain it , first lets start with what the quiz asks us to do : Correct the existing hasTile method to return true if the tile is in the tiles field, and false if it isn't. You can solve this a few ways, however, I'd like you to practice returning the result of the expression that uses the index of a char in a String.
There's a lot of ways you can solve this , but let's do it the way our teacher wants us to do - "I'd like you to practice returning the result of the expression that uses the index of a char in a String."
Understanding the problem and finding a way how to solve it is the first move you want to make when coding, don't just start to code, try to understand what's the problem first and wright down the solutions .
So what the teacher says , is that we have to "return" either true or false if the tile is in the tiles field or not , and we have to do this with "indexOf" method . You should know by now the indexOf method, if you dont here's the reminder -
The method indexOf() is used for finding out the index of the specified character or substring in a particular String. Remember that index-ing in Strings starts with 0 . the method will return -1 if the specified character was not found , and will return anything other than -1 if it is found.
Example:
String name = "Craig";
System.out.println(name.indexOf('a'));
//This will print 2 , because 'a' in String name is at index 2 ('C' - index 0 , 'r' - index 1 , and 'a' - index 2)
System.out.println(name.indexOf('z'));
//This will print -1 , because there is no 'z' in 'Craig'.
now that we know how indexOf() works , let's solve this problem .
return tiles.indexOf(tile)>=0;
This is the solution, let's break it into pieces and try to understand what it does . 'tiles' , is the String from where we want to find the character, 'tile' is the character that we want to find , so - tiles.indexOf(tile) means that we want to find the character - 'tile' from the String 'tiles' , Don't get confused, they both are variables which hold String and Char .Now remember that this will return number -1 if there is no 'tile' in 'tiles' , and will return the corresponding index of 'tile' if there is 'tile' in 'tiles' (sorry for bad English) . Now we want to try a way that this method will return either false or true if whatever 'tiles' variable holds , does have a Char 'tile' , How to do it ? We just say- ' >= 0' . So basically what we are doing is that , we are checking that whatever tiles.indexOf(tile) will return , will be more than, or equal to 0 (zero is index , don't forget), if it is below 0 , which will be -1 , it means that 'tile' is not in the 'tiles' and the statemant will return false because -1 is not more than or equal to 0, if it returns anything other than -1 , it will mean that 'tile' is in fact in the 'tiles' , and will return the corresponding index number which will be more than or equal to 0 and the statement will return true. I know this might seem confusing but here are some examples so you can understand it better :
String tiles = "Pen" //this creates String variable 'tiles' and sets it to the 'Pen'
Char tile = "z" //this creates the Char variable 'tile' and sets it to the character 'z'
return tiles.indexOf(tile); // this will return -1 because there is no z in the Pen;
return tiles.indexOf(tile)>=0; // this will return false, because -1 is not more than or equal to 0 . -1>=0 false
Char secondTile = 'P';
return tiles.indexOf(secondTile); //this will return 0 because 'P' is the zero'th index in the String tiles , ('P' - 0 , 'e' - 1 , 'n' - 2);
return tiles.indexOf(secondTile)>=0 ; //this will return true, because 'P' is in fact in the tiles variable in the index 0 . So it will say 0>=0 and it's true.
Hope this cleared it up, if you have any questions I'll try to answer it . Good luck :)
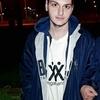
Tornike Shelia
2,781 PointsCan you be more specific , what you don't understand so I can try and help you ?

maor baruch
747 Pointsnvm i don't need help any more but still thank you :)
maor baruch
747 Pointsmaor baruch
747 Pointsno i am not talking about this method i'm talking about getCountOfLetter method