Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial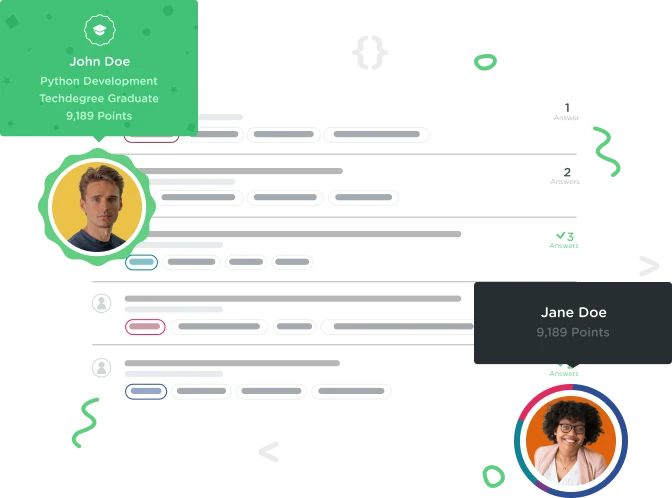
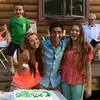
David Axelrod
36,073 PointsHELP ME IMPROVE!!!
using the context of the first code challenge in this module, here's the code i wrote
def nchoices(iterable, integer):
import random
list = []
counter = 0
while integer > counter:
list.append(random.choice(iterable))
counter +=1
return list
It passes but i have a feeling its a little long-winded and i'd like to improve my code. How can i make it more efficient? I'd like to use enumerate a bit more but i dont understand it 1000%
Thanks in advance!!
1 Answer
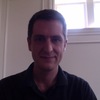
Max Hirsh
16,773 PointsWell, one improvement to make (this is discussed later in the python series when Kenneth goes over PEP-8, so keep going!) is that it's best practice to import modules at the top of your code, before defining functions or variables. You don't necessarily have to know that at this point though. You could make your code more compact by writing it in a for loop instead of adding a counter, e.g. "for i in range(n)". The function "range(n)" creates a list of number starting from 0 up to n-1, with a length n. Because of this, the line "for i in range(n):" performs whatever is inside the loop n times. Beyond that it's important to realize that sometimes the most "compact" code is not the most readable code. Sometimes it's better to write code that's longer or more repetitive if it makes it easier to edit or expand later.
Skidrow Triangle
657 PointsSkidrow Triangle
657 PointsYou could use a for loop and range to get rid of the counter and make it more compact:
Also, you should probably import random at the very beginning of your program instead of inside a function.