Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial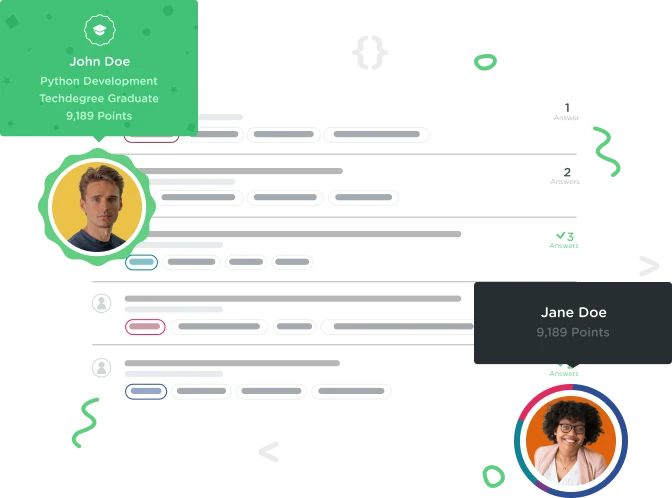
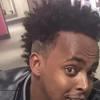
Abdijabar Mohamed
1,462 PointsHelp me in debugging my code
//I want to write "Dr. Smith"
class Person { let firstName: String let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below class Doctor: person { let theinitial: String override init(firstName: String, lastName: String) { self.theinitial = "Dr." super.init(firsName: firstName, lastName: lastName) }
override func getFullName() -> String {
return "\(theinitial) \(lastName)"
}
}
let someDoctor = Doctor(firstName: "Sam", lastName: "Smith")
3 Answers
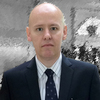
Nathan Tallack
22,160 PointsMy comments for corrections inline with the code. You were VERY close. Keep up the great work!
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}
class Doctor: Person { // The parent class is case sensitive.
let theinitial: String
override init(firstName: String, lastName: String) {
self.theinitial = "Dr."
super.init(firstName: firstName, lastName: lastName) // Missing t in firstName label.
}
override func getFullName() -> String {
return "\(theinitial) \(lastName)"
}
}
let someDoctor = Doctor(firstName: "Sam", lastName: "Smith")
print(someDoctor.getFullName()) // This uses the method to get your Dr. Smith to print.
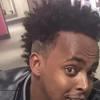
Abdijabar Mohamed
1,462 PointsThank you so much Nathan. Appreciated.
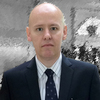
Nathan Tallack
22,160 PointsNo problem. Feel free to bring anything else to the forums. We love to help. :)
Try to surround your code with swift before the first line and
after the last line with an empty line above and below so that it formats correctly like it did when i pasted it. Helps us to read it. ;)
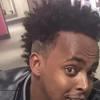
Abdijabar Mohamed
1,462 PointsLike this? //Swift .............. ........ ..... //Swift