Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial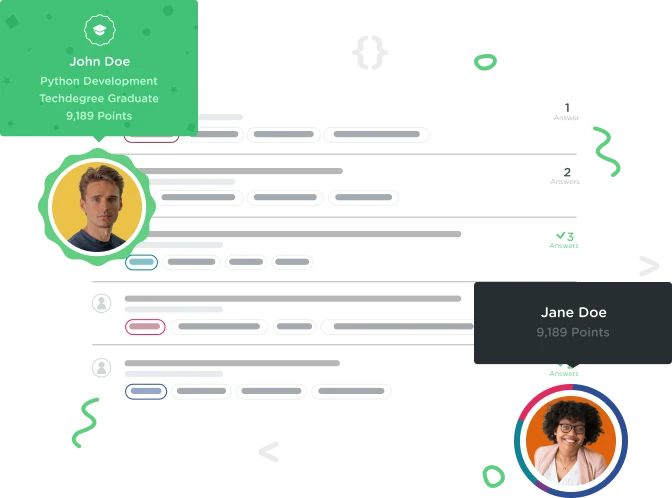
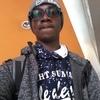
Elvin Mazwimairi
15,541 PointsHelp me out, I can't seem to find my problem in Task 2 of the flask rest api
It is returning this Bummer: IngredientList.get()
should return a string; Ingredient.get()
should accept an id
and return a string
import datetime
from peewee import *
DATABASE = SqliteDatabase('recipes.db')
class Recipe(Model):
name = CharField()
created_at = DateTimeField(default=datetime.datetime.now)
class Meta:
database = DATABASE
# TODO: Ingredient model
# name - string (e.g. "carrots")
# description - string (e.g. "chopped")
# quantity - decimal (e.g. ".25")
# measurement_type - string (e.g. "cups")
# recipe - foreign key
class Ingredient(Model):
name = CharField()
description = CharField()
quantity = DecimalField()
measurement_type = CharField()
recipe = ForeignKeyField(Recipe)
class Meta:
database = DATABASE
from flask import Blueprint
from flask.ext.restful import (
Resource, Api, reqparse, inputs,
marshal, marshal_with, fields
)
import models
ingredient_fields = {
'name': fields.String,
'description': fields.String,
'measurement_type': fields.String,
'quantity': fields.Float,
'recipe': fields.String
}
class IngredientList(Resource):
def __init__(self):
self.reqparse = reqparse.RequestParser()
self.reqparse.add_argument(
'name',
required=True,
location=['form', 'json']
)
self.reqparse.add_argument(
'description',
required=True,
location=['form', 'json']
)
self.reqparse.add_argument(
'measurement_type',
required=True,
location=['form', 'json']
)
self.reqparse.add_argument(
'quantity',
type=float,
required=True,
location=['form', 'json']
)
self.reqparse.add_argument(
'recipe',
type=inputs.positive,
required=True,
location=['form', 'json']
)
super().__init__()
def get(self):
ingredient = [marshal(ingreidient, ingredient_fields) for ingredient in models.Ingredient.select()]
return {'ingredients': ingredient}
@marshal_with(ingredient_fields)
def post(self):
args = self.reqparse.parse_args()
ingredient = models.Ingredient.create(**args)
return ingredient
class Ingredient(Resource):
def __init__(self):
self.reqparse = reqparse.RequestParser()
self.reqparse.add_argument(
'name',
required=True,
location=['form', 'json']
)
self.reqparse.add_argument(
'description',
required=True,
location=['form', 'json']
)
self.reqparse.add_argument(
'measurement_type',
required=True,
location=['form', 'json']
)
self.reqparse.add_argument(
'quantity',
type=float,
required=True,
location=['form', 'json']
)
self.reqparse.add_argument(
'recipe',
type=inputs.positive,
required=True,
location=['form', 'json']
)
super().__init__()
@marshal_with(ingredient_fields)
def get(self, id):
ingredient = models.Ingredient.get(models.Ingredient.id==id)
return ingredient
ingredients_api = Blueprint('resources.ingredients', __name__)
api = Api(ingredients_api)
api.add_resource(IngredientList, '/api/v1/ingredients')
api.add_resource(Ingredient, '/api/v1/ingredients/<int:id>')
1 Answer
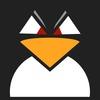
mhjp
20,372 PointsI think you are over complicating this. The Challenge is just asking you to define the Resource class and get methods.
from flask.ext.restful import Resource
import models
class IngredientList(Resource):
def get(self):
return 'Got Ingredients?'
class Ingredient(Resource):
def get(self, id):
return 'Got Milk?'
Elvin Mazwimairi
15,541 PointsElvin Mazwimairi
15,541 PointsOkay, thank you mhjp . It seems , yeah, i have been way off course. I mistakenly interlooped that with my answer to following challenge