Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial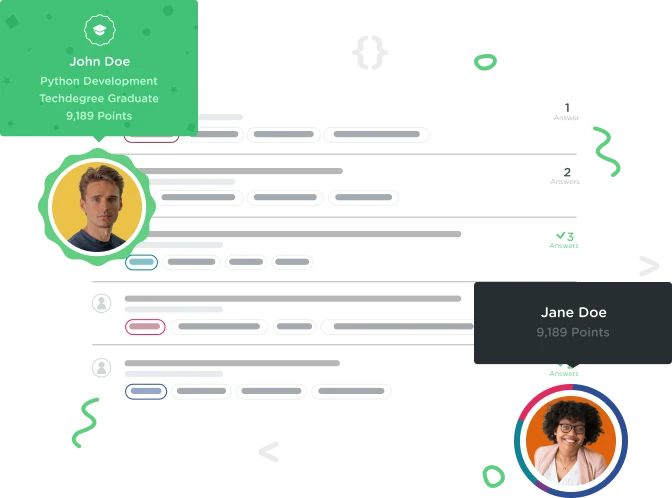
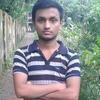
Niyamat Almass
8,176 PointsHelp me please.My app is crash!!!!!!!!
I finished Build a simple weather app course.But on the last my app is crash. After a sometime loading my app is crash!
package com.almass.niyamat.stormy;
import android.app.Activity;
import android.content.Context;
import android.graphics.drawable.Drawable;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.ProgressBar;
import android.widget.TextView;
import android.widget.ImageView;
import android.widget.Toast;
import com.squareup.okhttp.Call;
import com.squareup.okhttp.Callback;
import com.squareup.okhttp.OkHttpClient;
import com.squareup.okhttp.Request;
import com.squareup.okhttp.Response;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import butterknife.Bind;
import butterknife.ButterKnife;
public class MainActivity extends Activity {
public static final String TAG = MainActivity.class.getSimpleName();
private CurrentWeather mCurrentWeather;
@Bind(R.id.timeLabel) TextView mTimeLabel;
@Bind(R.id.temperatureLabel) TextView mTemperatureLabel;
@Bind(R.id.humidityValue) TextView mHumidityValue;
@Bind(R.id.precipValue) TextView mPrecipValue;
@Bind(R.id.summaryLabel) TextView mSummaryLabel;
@Bind(R.id.iconImageView) ImageView mIconImageView;
@Bind(R.id.refreshImageView) ImageView mRefreshImageView;
@Bind(R.id.progressBar) ProgressBar mProgressBar;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ButterKnife.bind(this);
mProgressBar.setVisibility(View.INVISIBLE);
final double latitude = 37.8267;
final double longitude = -122.423;
mRefreshImageView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
getForecast(latitude, longitude);
}
});
getForecast(latitude, longitude);
Log.d(TAG, "Main UI code is running");
}
private void getForecast(double latitude, double longitude) {
String apiKey = "b4de63a98386f7e128deaa126a3e23dd";
String forecastUrl = "https://api.forecast.io/forecast/" + apiKey + "/" + latitude + "," + longitude;
if (isNetworkAvailable()) {
toggleRefresh();
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Request request, IOException e) {
runOnUiThread(new Runnable() {
@Override
public void run() {
toggleRefresh();
}
});
alertUserAboutError();
}
@Override
public void onResponse(Response response) throws IOException {
runOnUiThread(new Runnable() {
@Override
public void run() {
toggleRefresh();
}
});
try {
String jsonData = response.body().string();
Log.v(TAG, jsonData);
if (response.isSuccessful()) {
mCurrentWeather = getCurrentDetails(jsonData);
runOnUiThread(new Runnable() {
@Override
public void run() {
updateDisplay();
}
});
updateDisplay();
} else {
alertUserAboutError();
}
} catch (IOException e) {
Log.e(TAG, "Exception caught: ", e);
}
catch (JSONException e) {
Log.e(TAG, "Exception caught: ", e);
}
}
});
}
else {
Toast.makeText(this, getString(R.string.network_error_message), Toast.LENGTH_LONG).show();
}
}
private void toggleRefresh() {
if (mProgressBar.getVisibility() == View.INVISIBLE) {
mProgressBar.setVisibility(View.VISIBLE);
mRefreshImageView.setVisibility(View.INVISIBLE);
}
else {
mProgressBar.setVisibility(View.INVISIBLE);
mRefreshImageView.setVisibility(View.VISIBLE);
}
}
private void updateDisplay() {
mTemperatureLabel.setText(mCurrentWeather.getTemperature() + "");
mTimeLabel.setText("At " + mCurrentWeather.getFormattedTime() + " it will be");
mHumidityValue.setText(mCurrentWeather.getHumidity() + "");
mPrecipValue.setText(mCurrentWeather.getPrecipitation() + "%");
mSummaryLabel.setText(mCurrentWeather.getSummery());
Drawable drawable = getResources().getDrawable(mCurrentWeather.getIconId());
mIconImageView.setImageDrawable(drawable);
}
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
Log.i(TAG, "From Json:" + timezone);
JSONObject currently = forecast.getJSONObject("currently");
CurrentWeather currentWeather = new CurrentWeather();
currentWeather.setHumidity(currently.getDouble("humidity"));
currentWeather.setTime(currently.getLong("time"));
currentWeather.setIcon(currently.getString("icon"));
currentWeather.setPrecipitation(currently.getDouble("precipProbability"));
currentWeather.setTemperature(currently.getDouble("temperature"));
currentWeather.setSummery(currently.getString("summary"));
currentWeather.setTimeZone(timezone);
Log.d(TAG, currentWeather.getFormattedTime());
return currentWeather;
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()) {
isAvailable = true;
}
return isAvailable;
}
private void alertUserAboutError() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(), "error");
}
}
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin"
tools:context=".MainActivity"
android:background="#fffc970b"
android:id="@+id/relativeLayout">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="--"
android:id="@+id/temperatureLabel"
android:layout_centerVertical="true"
android:layout_centerHorizontal="true"
android:textColor="@android:color/white"
android:textIsSelectable="false"
android:textSize="150sp"/>
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/degreeImageView"
android:layout_toEndOf="@+id/temperatureLabel"
android:paddingTop="40dp"
android:src="@drawable/degree"
android:layout_alignTop="@+id/temperatureLabel"
android:layout_toRightOf="@+id/temperatureLabel"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="..."
android:id="@+id/timeLabel"
android:layout_above="@+id/temperatureLabel"
android:layout_centerHorizontal="true"
android:textColor="#80ffffff"
android:textSize="18sp"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Alcatraz Island,CA"
android:id="@+id/locationLabel"
android:layout_above="@+id/timeLabel"
android:layout_centerHorizontal="true"
android:paddingBottom="60dp"
android:textColor="@android:color/white"
android:textSize="24sp"/>
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/iconImageView"
android:layout_alignTop="@+id/locationLabel"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:src="@drawable/cloudy_night"/>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/temperatureLabel"
android:layout_alignParentRight="true"
android:layout_alignParentEnd="true"
android:layout_marginTop="10dp"
android:weightSum="100"
android:id="@+id/linearLayout">
<LinearLayout
android:orientation="vertical"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_weight="50">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="HUMIDITY"
android:id="@+id/humidityLabel"
android:textColor="#80ffffff"
android:gravity="center_horizontal"/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="--"
android:id="@+id/humidityValue"
android:textColor="@android:color/white"
android:textSize="24sp"
android:gravity="center_horizontal"/>
</LinearLayout>
<LinearLayout
android:orientation="vertical"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_weight="50">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="RAIN/SNOW?"
android:id="@+id/precipValue"
android:textColor="#80ffffff"
android:gravity="center_horizontal"/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="--"
android:id="@+id/precipValue"
android:textColor="@android:color/white"
android:textSize="24sp"
android:gravity="center_horizontal"/>
</LinearLayout>
</LinearLayout>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Getting current weather..."
android:id="@+id/summaryLabel"
android:layout_below="@+id/linearLayout"
android:layout_centerHorizontal="true"
android:layout_marginTop="40dp"
android:textColor="@android:color/white"
android:textSize="18sp"
android:gravity="center_horizontal"/>
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/refreshImageView"
android:src="@drawable/refresh"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"/>
<ProgressBar
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/progressBar"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_alignBottom="@+id/refreshImageView"/>
</RelativeLayout>
and the error of logcat
08-16 07:33:51.592 1207-1207/com.almass.niyamat.stormy D/dalvikvm﹕ Late-enabling CheckJNI
08-16 07:33:52.744 1207-1207/com.almass.niyamat.stormy D/dalvikvm﹕ GC_FOR_ALLOC freed 123K, 6% free 3896K/4124K, paused 2ms, total 2ms
08-16 07:33:52.808 1207-1207/com.almass.niyamat.stormy D/MainActivity﹕ Main UI code is running
08-16 07:33:54.568 1207-1207/com.almass.niyamat.stormy D/libEGL﹕ loaded /system/lib/egl/libEGL_genymotion.so
08-16 07:33:54.576 1207-1207/com.almass.niyamat.stormy D/﹕ HostConnection::get() New Host Connection established 0xb9062b28, tid 1207
08-16 07:33:55.148 1207-1207/com.almass.niyamat.stormy D/libEGL﹕ loaded /system/lib/egl/libGLESv1_CM_genymotion.so
08-16 07:33:55.148 1207-1207/com.almass.niyamat.stormy D/libEGL﹕ loaded /system/lib/egl/libGLESv2_genymotion.so
08-16 07:33:55.684 1207-1207/com.almass.niyamat.stormy W/EGL_genymotion﹕ eglSurfaceAttrib not implemented
08-16 07:33:55.684 1207-1207/com.almass.niyamat.stormy E/OpenGLRenderer﹕ Getting MAX_TEXTURE_SIZE from GradienCache
08-16 07:33:55.688 1207-1207/com.almass.niyamat.stormy E/OpenGLRenderer﹕ MAX_TEXTURE_SIZE: 16384
08-16 07:33:55.692 1207-1207/com.almass.niyamat.stormy E/OpenGLRenderer﹕ Getting MAX_TEXTURE_SIZE from Caches::initConstraints()
08-16 07:33:55.692 1207-1207/com.almass.niyamat.stormy E/OpenGLRenderer﹕ MAX_TEXTURE_SIZE: 16384
08-16 07:33:55.692 1207-1207/com.almass.niyamat.stormy D/OpenGLRenderer﹕ Enabling debug mode 0
08-16 07:33:56.856 1207-1207/com.almass.niyamat.stormy I/Choreographer﹕ Skipped 75 frames! The application may be doing too much work on its main thread.
08-16 07:34:16.224 1207-1207/com.almass.niyamat.stormy I/Choreographer﹕ Skipped 124 frames! The application may be doing too much work on its main thread.
08-16 07:34:21.795 1207-1226/com.almass.niyamat.stormy W/dalvikvm﹕ VFY: unable to find class referenced in signature (Ljava/nio/file/Path;)
08-16 07:34:21.795 1207-1226/com.almass.niyamat.stormy W/dalvikvm﹕ VFY: unable to find class referenced in signature ([Ljava/nio/file/OpenOption;)
08-16 07:34:21.795 1207-1226/com.almass.niyamat.stormy I/dalvikvm﹕ Could not find method java.nio.file.Files.newOutputStream, referenced from method okio.Okio.sink
08-16 07:34:21.795 1207-1226/com.almass.niyamat.stormy W/dalvikvm﹕ VFY: unable to resolve static method 2133: Ljava/nio/file/Files;.newOutputStream (Ljava/nio/file/Path;[Ljava/nio/file/OpenOption;)Ljava/io/OutputStream;
08-16 07:34:21.795 1207-1226/com.almass.niyamat.stormy D/dalvikvm﹕ VFY: replacing opcode 0x71 at 0x000a
08-16 07:34:21.795 1207-1226/com.almass.niyamat.stormy W/dalvikvm﹕ VFY: unable to find class referenced in signature (Ljava/nio/file/Path;)
08-16 07:34:21.795 1207-1226/com.almass.niyamat.stormy W/dalvikvm﹕ VFY: unable to find class referenced in signature ([Ljava/nio/file/OpenOption;)
08-16 07:34:21.795 1207-1226/com.almass.niyamat.stormy I/dalvikvm﹕ Could not find method java.nio.file.Files.newInputStream, referenced from method okio.Okio.source
08-16 07:34:21.795 1207-1226/com.almass.niyamat.stormy W/dalvikvm﹕ VFY: unable to resolve static method 2132: Ljava/nio/file/Files;.newInputStream (Ljava/nio/file/Path;[Ljava/nio/file/OpenOption;)Ljava/io/InputStream;
08-16 07:34:21.795 1207-1226/com.almass.niyamat.stormy D/dalvikvm﹕ VFY: replacing opcode 0x71 at 0x000a
08-16 07:34:22.723 1207-1226/com.almass.niyamat.stormy D/dalvikvm﹕ GC_FOR_ALLOC freed 343K, 11% free 4034K/4484K, paused 19ms, total 19ms
08-16 07:34:22.723 1207-1226/com.almass.niyamat.stormy V/MainActivity﹕ {"latitude":37.8267,"longitude":-122.423,"timezone":"America/Los_Angeles","offset":-7,"currently":{"time":1439710462,"summary":"Partly Cloudy","icon":"partly-cloudy-night","nearestStormDistance":369,"nearestStormBearing":112,"precipIntensity":0,"precipProbability":0,"temperature":65.12,"apparentTemperature":65.12,"dewPoint":56.31,"humidity":0.73,"windSpeed":2.09,"windBearing":264,"visibility":9.34,"cloudCover":0.59,"pressure":1012.17,"ozone":292.53},"minutely":{"summary":"Partly cloudy for the hour.","icon":"partly-cloudy-night","data":[{"time":1439710440,"precipIntensity":0,"precipProbability":0},{"time":1439710500,"precipIntensity":0,"precipProbability":0},{"time":1439710560,"precipIntensity":0,"precipProbability":0},{"time":1439710620,"precipIntensity":0,"precipProbability":0},{"time":1439710680,"precipIntensity":0,"precipProbability":0},{"time":1439710740,"precipIntensity":0,"precipProbability":0},{"time":1439710800,"precipIntensity":0,"precipProbability":0},{"time":1439710860,"precipIntensity":0,"precipProbability":0},{"time":1439710920,"precipIntensity":0,"precipProbability":0},{"time":1439710980,"precipIntensity":0,"precipProbability":0},{"time":1439711040,"precipIntensity":0,"precipProbability":0},{"time":1439711100,"precipIntensity":0,"precipProbability":0},{"time":1439711160,"precipIntensity":0,"precipProbability":0},{"time":1439711220,"precipIntensity":0,"precipProbability":0},{"time":1439711280,"precipIntensity":0,"precipProbability":0},{"time":1439711340,"precipIntensity":0,"precipProbability":0},{"time":1439711400,"precipIntensity":0,"precipProbability":0},{"time":1439711460,"precipIntensity":0,"precipProbability":0},{"time":1439711520,"precipIntensity":0,"precipProbability":0},{"time":1439711580,"precipIntensity":0,"precipProbability":0},{"time":1439711640,"precipIntensity":0,"precipProbability":0},{"time":1439711700,"precipIntensity":0,"precipProbability":0},{"time":1439711760,"precipIntensity":0,"precipProbability":0},{"time":1439711820,"precipIntensity":0,"precipProbability":0},{"time":1439711880,"precipIntensity":0,"precipProbability":0},{"time":1439711940,"precipIntensity":0,"precipProbability":0},{"time":1439712000,"precipIntensity":0,"precipProbability":0},{"time":1439712060,"precipIntensity":0,"precipProbability":0},{"time":1439712120,"precipIntensity":0,"precipProbability":0},{"time":1439712180,"precipIntensity":0,"precipProbability":0},{"time":1439712240,"precipIntensity":0,"precipProbability":0},{"time":1439712300,"precipIntensity":0,"precipProbability":0},{"time":1439712360,"precipIntensity":0,"precipProbability":0},{"time":1439712420,"precipIntensity":0,"precipProbability":0},{"time":1439712480,"precipIntensity":0,"precipProbability":0},{"time":1439712540,"precipIntensity":0,"precipProbability":0},{"time":1439712600,"precipIntensity":0,"precipProbability":0},{"time":1439712660,"precipIntensity":0,"precipProbability":0},{"time":1439712720,"precipIntensity":0,"precipProbability":0},{"time":1439712780,"precipIntensity":0,"precipProbability":0},{"time":1439712840,"precipIntensity":0,"precipProbability":0},{"time":1439712900,"precipIntensity":0,"precipProbability":0},{"time":1439712960,"precipIntensity":0,"precipProbability":0},{"time":1439713020,"precipIntensity":0,"precipProbability":0},{"time":1439713080,"precipIntensity":0,"precipProbability":0},{"time":1439713140,"precipIntensity":0,"precipProbability":0},{"time":1439713200,"precipIntensity":0,"precipProbability":0},{"time":1439713260,"precipIntensity":0,"precipProbability":0},{"time":1439713320,"precipIntensity":0,"precipProbability":0},{"time":1439713380,"precipIntensity":0,"precipProbability":0},{"time":1439713440,"precipIntensity":0,"precipProbability":0},{"time":1439713500,"precipIntensity":0,"precipProbability":0},{"time":1439713560,"precipIntensity":0,"precipProbability":0},{"time":1439713620,"precipIntensity":0,"precipProbability":0},{"time":1439713680,"precipIntensity":0,"precipProbability":0},{"time":1439713740,"precipIntensity":0,"precipProbability":0},{"time":1439713800,"precipIntensity":0,"precip
08-16 07:34:22.763 1207-1226/com.almass.niyamat.stormy I/MainActivity﹕ From Json:America/Los_Angeles
08-16 07:34:22.787 1207-1226/com.almass.niyamat.stormy D/MainActivity﹕ 12:34 AM
08-16 07:34:22.787 1207-1226/com.almass.niyamat.stormy W/dalvikvm﹕ threadid=11: thread exiting with uncaught exception (group=0xa4d57b20)
08-16 07:34:22.999 1207-1226/com.almass.niyamat.stormy D/dalvikvm﹕ GC_FOR_ALLOC freed 532K, 14% free 4014K/4652K, paused 2ms, total 2ms
08-16 07:34:22.999 1207-1226/com.almass.niyamat.stormy E/AndroidRuntime﹕ FATAL EXCEPTION: OkHttp Dispatcher
Process: com.almass.niyamat.stormy, PID: 1207
android.view.ViewRootImpl$CalledFromWrongThreadException: Only the original thread that created a view hierarchy can touch its views.
at android.view.ViewRootImpl.checkThread(ViewRootImpl.java:6024)
at android.view.ViewRootImpl.requestLayout(ViewRootImpl.java:820)
at android.view.View.requestLayout(View.java:16431)
at android.view.View.requestLayout(View.java:16431)
at android.view.View.requestLayout(View.java:16431)
at android.view.View.requestLayout(View.java:16431)
at android.widget.RelativeLayout.requestLayout(RelativeLayout.java:352)
at android.view.View.requestLayout(View.java:16431)
at android.widget.TextView.checkForRelayout(TextView.java:6600)
at android.widget.TextView.setText(TextView.java:3813)
at android.widget.TextView.setText(TextView.java:3671)
at android.widget.TextView.setText(TextView.java:3646)
at com.almass.niyamat.stormy.MainActivity.updateDisplay(MainActivity.java:145)
at com.almass.niyamat.stormy.MainActivity.access$500(MainActivity.java:31)
at com.almass.niyamat.stormy.MainActivity$2.onResponse(MainActivity.java:111)
at com.squareup.okhttp.Call$AsyncCall.execute(Call.java:170)
at com.squareup.okhttp.internal.NamedRunnable.run(NamedRunnable.java:33)
at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1112)
at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:587)
at java.lang.Thread.run(Thread.java:841)
Please help me!!
3 Answers
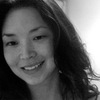
michikoote
20,646 PointsBy looking at your log file I'd guess that
08-16 07:34:22.999 1207-1226/com.almass.niyamat.stormy E/AndroidRuntime﹕ FATAL EXCEPTION: OkHttp Dispatcher Process: com.almass.niyamat.stormy, PID: 1207
is causing the crash.
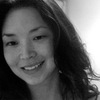
michikoote
20,646 PointsIt says "FATAL EXCEPTION: OkHttp Dispatcher Process" go over the OkHttp library to see if its doing anything wrong. Good luck!
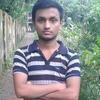
Niyamat Almass
8,176 PointsI found the problem.There are some problem for copy and pasting
Niyamat Almass
8,176 PointsNiyamat Almass
8,176 PointsBut what is it? And how to solve the error.