Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial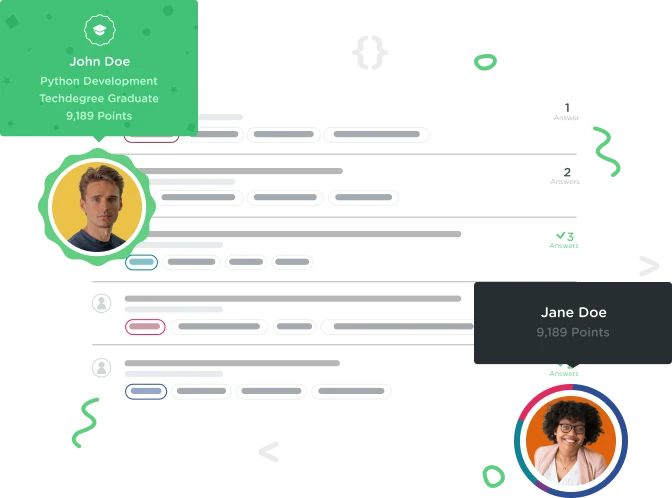

siva sanka
4,099 Pointshelp me regarding this
please find details in attachments
# EXAMPLE
# random_item("Treehouse")
# The randomly selected number is 4.
# The return value would be "h"
import random
random_item=("Treehouse")
random_number=random.randint(0,(len(random_item)-1))
return random_item[random_number]
1 Answer
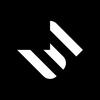
Michal Strnad
1,784 PointsFirst things first. Our first task is to import random and create a function. Functions are created by using:
def name_of_the_function(arguments):
That function has to be named random_item and it has to take one argument (its name is up to you). So the following code will now look like:
import random
def random_item(iterable):
...
And now the most important part - the functionality itself. We need to use the random.randint() to get a random number between 0 and the length of the iterable that will be passed to it. Iterable is something that you can iterate through so it can be a list, tuple or a dictionary. It's basically something that holds more variables at once(more numbers, strings etc.).
And now you just have to get a random number between 0 and the length of the iterable and return a member of the iterable itself that has the index of the random number.
So the following code will look like:
import random
def random_item(iterable):
random_number = random.randint(0, (len(iterable)-1))
return iterable[random_number]
Feel free to ask if there's something that you still don't understand.