Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial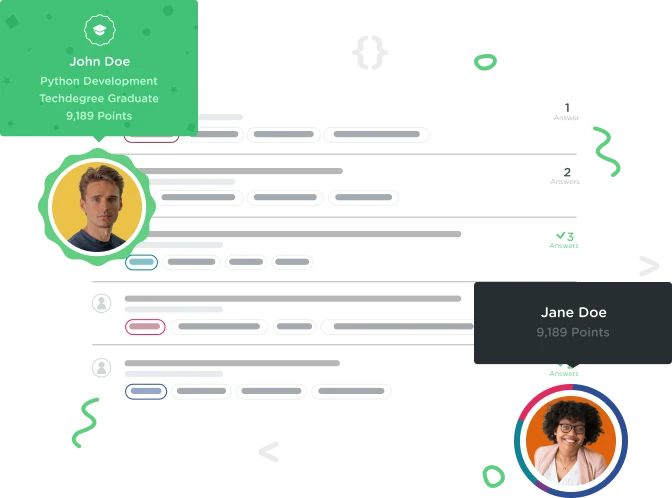

mryoung
4,156 PointsHelp me understand how this function is interpreted
So, I'm following the videos and have written this out:
function exec(func, arg) {
func(arg);
}
exec((something) => {
console.log(something);
}, 'greetings');
I understand this section below is required for the rest of the function to execute. In the console it say that it's needed to define the variable exec.
function exec(func, arg) {
func(arg);
}
So, is there a difference between how the variable is declared here
var f = function exec (something) {
console.log(something)
};
and how exec is the name of the function here?
function exec (something) {
console.log(something)
};
I'm just not understanding how the first half is required, since a variable actually isn't defined, only a function is defined, and then in the second half it's passed arguments to perform.
1 Answer
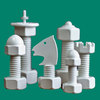
Steven Parker
229,732 PointsThese are two different ways of creating a function. Your first example is a function expression:
var f = function exec(something) {
console.log(something)
};
This creates "f" as the function. The function has a name of "exec" (f.name) but otherwise the word "exec" is not defined elsewhere in the program. "f" can be called after this operation is performed.
Your second example is a function declaration:
function exec(something) {
console.log(something)
};
In this case, the function "exec" is created. And a declaration is hoisted which means "exec" can be called from anywhere in the program, even before the declaration.
For more details, see the MDN pages on function expression and function declaration.