Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial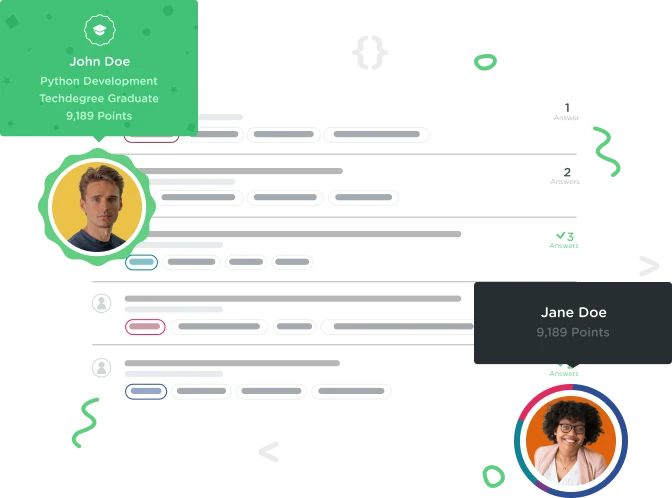

Travis Joyner
14,553 PointsHelp me understand how we use $_GET to create a product detail page.
I've watched the "Building the Shirt Details Page" video several times, and have been able to use the code to create my own detail page, but the explanation for HOW it works is still muddy.
I understand this bit of code, and how it pulls in the product ID from our array and generates a new page with it when we click on the anchor:
<a href="shirt.php?id=<?php echo $product_id; ?>">
But I don't understand exactly what this code on the detail page itself is doing and how we're passing the key from page to page. Can someone explain like I'm 5 years old?
$product_id = $_GET["id"];
$product = $products[$product_id];
4 Answers
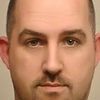
Steven Snary
17,540 PointsThe assumptions made in this answer are as follows...
- Just to clarify to any readers, the first piece of code listed above is from the shirts.php page and the second bit of code came from the top of the shirt.php page. We click on the shirt listed on the shirts.php page to see the details of that shirt on the shirt.php page.
- Throughout this answer I'll assume the user clicked on the shirt with an id of 108.
So let's focus on the second piece of code... The first line of code would equate to this
$product_id = 108
How was the value of 108 passed to the shirt.php page from the shirts.php page - here's how...
The way the id of 108 gets passed from the shirts.php page to the shirt.php page is by your first line of code..
<a href="shirt.php?id=108">
- (I've just switched out the php code from your first line with the id that would have been generated assuming that we clicked on the shirt with id 108). This code passes the id of 108 from the shirts.php page using the
$_GET
variable to the shirt.php file. That's what the ? in the URL stands for - $_GET.
So by clicking on the anchor for shirt 108 from the shirts.php page we are taken to the shirt.php page using the id of 108 that is stored in the $_GET variable.
The last line of code accomplishes the following...
This line of code will set the $product variable (on the shirt.php page) equal to $products[108] array. (remember that the square brackets [] indicate an array is being set. This pulls in all the information from our products.php file (which is that array of all the shirts for the site because products.php was included at the top of our shirt.php page).
So this line of code will set the $product variable equal to $products[108]. This will "import" or set the values of name, img, price, paypal and sizes from the products page so that only the values for shirt 108 are used on the shirt.php page.
** EDIT ** Thanks for the tip Travis - now if someone could point me to the information on how to import the nice pieces of code as listed in the question that would be great.
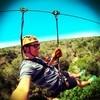
thomascawthorn
22,986 PointsCracking answers here.
There are a two methods you're going to come across when passing data between pages as a beginner. There are a few more ways but the course you're on will cover these two.
$_GET describes variables coming in through the url string. I see you've picked up how these are written, but you can expand a little further. What hasn't been mentioned just yet is that you can send any number of parameters through the url by concatenating with an ampersand (&). You only need a single question mark at the beginning to start the sequence. This string should always go at the end of the url.
www.test.com/i-love-folders/shirt.php?id=108&color=red&size=large&favourite=true"
If you were to var_dump($_GET) - which i suggest you do to really see what's going on - you'll see it's just like any other array. Dumping the above would give you:
<?php
// var_dump($_GET);
// exit;
// will output:
array (
'id' => '108',
'color' => 'red',
'size' => 'large',
'favourite' => 'true'
)
variables passed through the url - be they integers, booleans or strings - will always be converted to strings (not important now... but good to know!)
All the data from the url is wrapped up warm and snug for you to call from anywhere. $_GET is specific just to parameters passed into the url, and it's called super global. If you're unfamiliar with scoping, this essentially means you can use $_GET anywhere in your code without worry. Most things in CAPS in PHP are constants / very important. $_GET & $_POST are described as reserved or predefined variables. Essentially you can't use $_GET as your own variable because it's special.
In the Shirts4Mike example, you grab a specific value from the $_GET array (the shirt id) and use that value to select data from another array.
$_POST is the same but different.. The information is passed through the request "behind the scenes". It is generally used for form submissions because you don't want huge long message body strings to go through the URL, nor do you want things like passwords to show up in the URL!
You'll come to $_POST when you create the contact form for Shirts4Mike, so I won't delve too much right now.
you can read more about $_POST and $GET on the PHP Manual
Although potentially confusing at first, referring to the php manual will get a lot easier with time!
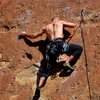
Shawn Flanigan
Courses Plus Student 15,815 PointsWhenever you see $_GET["something"];
, you're pulling a variable from the url. So, for example, if somebody goes to http://example.com?name=Travis
, then $_GET["name"]
will give us a value of Travis
.
In this exercise, if you go to shirts.php?id=101
, then $_GET["id"]
will give you a value of 101
.
The code you posted above pulls the id
from the url (101 in my example), stores it in a variable (called $product_id
) and then gets a product from your $products
array with the key of 101
.
Hope that helps, but if it's still not clear I can try again. :)

Travis Joyner
14,553 PointsThanks! This is helpful. So $_GET is ALWAYS referencing the URL? That's what I wasn't getting.
Also, when we use example.com?name=101, could "name" be anything we want? I used "id" but I'm assuming it's a variable like anything else.
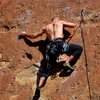
Shawn Flanigan
Courses Plus Student 15,815 PointsYup. It's just like any other variable. Call it whatever you want as long as it matches in your php.

ammarkhan
Front End Web Development Techdegree Student 21,661 PointsShawn Flanigan Still confused as i get the id of clicked item, but it gives me NULL when i assign it to other variable.
<?php
$product_id = $_GET['id'];
$product = $products[$product_id];
echo $product_id;
var_dump($product);
the var_dump is NULL but $product_id is giving the right value. The error says
Notice: Undefined variable: products
also i don't understand the $products array, is it coming from last page like we said
$products as $products.
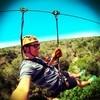
thomascawthorn
22,986 Points"also i don't understand the $products array, is it coming from last page like we said "
If you haven't defined $productis on the page you're currrently on, then that would explain the error you're getting. Variables don't persist between pages so you'll need to call the function that gives you all of your product details again on this page.
Better still, create a new function that will find just one product by it's ID ;)
Hope this helps :-)

ammarkhan
Front End Web Development Techdegree Student 21,661 PointsTom Cawthorn But if u see the video, there isn't any element name $products assigned variable before $products['$product_id'] , time seek 01:02
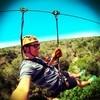
thomascawthorn
22,986 PointsI imagine that $products is defined within inc/products.php ?
The code you provided above doesn't include this inclusion - so maybe that's your problem :-)
Tom
Travis Joyner
14,553 PointsTravis Joyner
14,553 PointsThank you, that helps a lot. If you're asking how to format the dark blocks of code, you just put three tick marks before and after the code (the tick mark is the key to the left of the number 1 on most keyboards).
Steven Snary
17,540 PointsSteven Snary
17,540 PointsThanks for that advice on the 3 ticks - I did try that but maybe I didn't have the right spaces or something - I'll try again and figure it out.
<p>Test Code</p>
Well - there we go :-)