Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial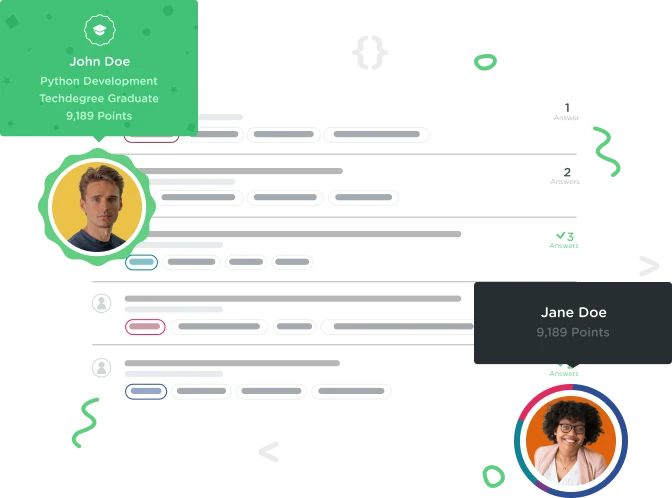
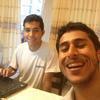
Wilfredo Casas
6,174 PointsHelp me with my code! Secret number game
Hello! I did my own version of the secret number game, it works almost perfectly, but there is a minor issue that appeared:
If I type a letter on the 2nd or 3rd try, the message "what? write a number man" appears, however on the 1rst try this error appears: local variable "index" referenced before assigment. What's happening? Thanks!
import random
def main():
randome = random.randint(1,10)
index = 0
while index < 3:
try:
guess = int(input("What's your number? "))
except ValueError:
print("what? write a number man")
index -= 1
#compare both numbers
if guess == randome:
print("jackpot!")
break
#give hit or miss
elif index == 2:
print("My number was {}, haha".format(randome))
print("GAME OVER")
again = input("play again? yes or no: ")
if again == "yes":
main()
break
else:
index += 1
if guess < randome:
print("higher!")
if guess > randome:
print("lower!")
print("nope, you have {} more trys".format(3- index))
main()
1 Answer
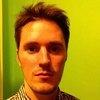
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsHere's what's happening when I do it:
What's your number? a
what? write a number man
Traceback (most recent call last):
File "game.py", line 37, in <module>
main()
File "game.py", line 15, in main
if guess == randome:
UnboundLocalError: local variable 'guess' referenced before assignment
So what's happening is you wrapped your guess input in a try except block. But when it fails, the guess variable doesn't get initalized at all. So then on line 15 when you try to compare something to a variable that doesn't exist, it breaks. If you guess a letter on the 2nd or 3rd guess, it was already successfully initialized on the 1st guess so you're okay. My solution, which may not be the ideal solution, is to add a line that initializes the guess variable when you start the program, but makes it something that will never be the answer...
def main():
randome = random.randint(1,10)
index = 0
guess = -1
....
Not sure if that's the ideal solution but it works. I also tried initializing it with None instead of an integer value, but that broke the code later in a different spot because it wasn't an integer.
Wilfredo Casas
6,174 PointsWilfredo Casas
6,174 PointsAwesome! Thanks for taking the time!
Joey B
5,275 PointsJoey B
5,275 PointsI ran into the same problem. Is this what Kenneth is talking about in the teacher's notes?
Did you catch the possible problem where we take a user's guess during the game? Since we convert their input()'d guess into an int right away, if they give us something that can't be turned into a number, it'll throw a ValueError. So far, so good, 'cause that's what our try is for, right?
Except in the except ValueError: we used the guess variable, which will be undefined and throw it's own new error. Oh no! Just take out the string formatting for that error message, though, and you should be good to go.
What does he mean by just take out the string formatting...?