Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial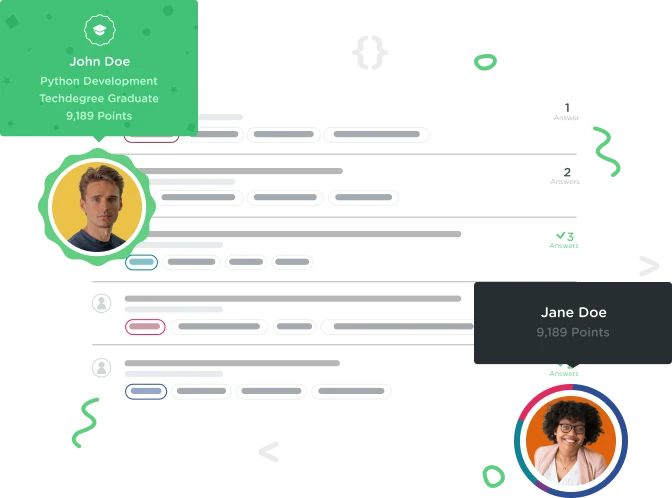

swiftdev
3,655 PointsHelp me with the "override" thing!
So I'm on this challenge and I really don't know or understand what I'm doing. I watched the video again, but I still don't understand what "override" does. Also while I'm trying to type things into playground and error keeps popping up that says "Consecutive declarations on a line must be separated by ';'" or "Expected declaration". I'm not trying to put any commands on the same line and I don't know why it's expecting another declaration. Can somebody help me with both of these?!
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
func incrementBy(points: Double){
width += points
height += points
}
}
class RoundButton: Button {
var cornerRadius: Double = 5.0
override func incrementBy(points: Double = 7.0){
width += points
height += points
}
1 Answer
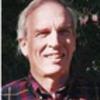
jcorum
71,830 PointsThis is a bit tricky. First, you are missing a closing curly brace at the end of your function. When that is fixed the compiler error is this:
swift_lint.swift:21:17: error: argument names for method 'incrementBy(points:)' do not match those of overridden method 'incrementBy'
override func incrementBy(points: Double = 7.0){
Yes, the documentation says default values can be done the way, you are doing it, but not when overriding. As the error message say, when overriding the argument names must be the same. What you need is this:
override func incrementBy(points: Double) {
width += 7.0
height += 7.0
}
What override does is create a function in a subclass with the same name and method signature (number and type of arguments or parameters) and a different code block. Actually, the code could be the same but then there would be little reason to do the override.
swiftdev
3,655 Pointsswiftdev
3,655 PointsThank you! I get it now.