Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial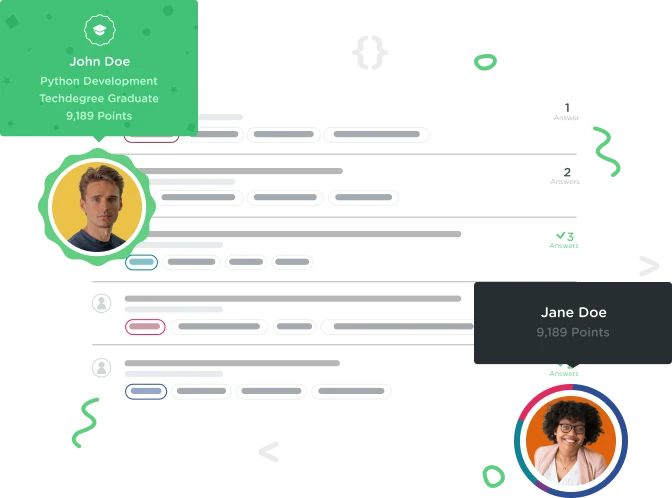

Tojo Alex
Courses Plus Student 13,331 Pointshelp needed
Hi
I have created a new class and created a public readonly field though this is the problem :
Did you define your Square class as an inner class of the Polygon class? Be sure to define the Square class before or after the definition for the Polygon class.
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
base NumSides = numSides;
}
}
class Square
{
public readonly int SideLength;
public Square (int sideLength)
{
base SideLength = sideLength;
}
}
}
3 Answers

Mikkel Madsen
4,229 PointsMost of what you did is correct. You just put the base wrong. it should come after the parenthesis in the Square method with a : between the base and the parenthesis. And then you will need a open and close parenthesis with the number 4.
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength;
public Square(int sideLength) : base(4)
{
SideLength = sideLength;
}
}
}
Hope this will help you.

Tojo Alex
Courses Plus Student 13,331 Pointsok i think I have done that though now I have the error : Does your Square class derive from the Polygon class?
This is my current code:
namespace Treehouse.CodeChallenges { class Polygon { public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square
{
public readonly int SideLength;
public Square (int sideLength)
{
base SideLength = sideLength;
}
}
}

Tojo Alex
Courses Plus Student 13,331 Pointsoh silly me thanks alot

Mikkel Madsen
4,229 PointsNo problem. Glad I could help. :)
Jake Basten
16,345 PointsJake Basten
16,345 PointsIt looks like some of your uses of the base keyword are not quite correct. Remember that Square is a Polygon, so that's where you should be using base - you don't need to use it in the Polygon class at all.
Because Square inherits from Polygon, Square will have a NumSides property as well as a SideLength property, so you need to make sure that both are set when you make a Square. The NumSides property which is inherited can be set when you declare the Square's constructor by using the base keyword and the base class' accepted constructor method signature. And the SideLength property can be set in the Square's constructor, the same way that NumSides is set in Polygon.