Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial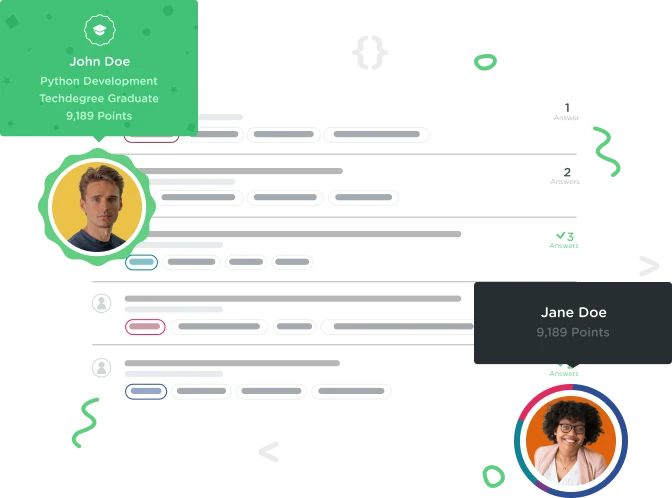

Gavin Eyquem
Front End Web Development Techdegree Student 20,735 PointsHelp needed
Getting confused.
My code isn't right, need a little bit of help.
var list = document.getElementsByTagName('ul')[0];
var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
let li = event.target.parentNode;
//let p = highlight.previousElementSibling;
let hightlight = li.previousElementSibling;
let ul = li.parentNode;
if(hightlight){
ul.insertBefore(hightlight, li);
}
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p>Element Selection</p><button>Highlight</button></li>
<li><p>Events</p><button>Highlight</button></li>
<li><p>Event Listening</p><button>Highlight</button></li>
<li><p>DOM Traversal</p><button>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
5 Answers

Siddharth Pandey
7,280 PointsRegarding your previous comment:
Am I any closer to getting it right?
Your code is:
var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) { if (e.target.tagName == 'BUTTON') { let li = e.target.parentNode; let highlight = li.previousElementSibling; let ul = li.parentNode; if(highlight){ ul.insertBefore(highlight, li); } } });
You need not select the 'listItem' all you have to do is select the paragraph which corresponds to the button pressed(HINT: which is a previousElementSibling of the button) and add the class "highlight" highlight is not a variable it is a class. I can give you the answer, but I think you want to figure it out yourself.

Siddharth Pandey
7,280 PointsI need more information to see what you are actually trying to achieve, but I did notice a syntax error over here
let li = event.target.parentNode;
right now the 'e' in your function is your event so it should just be:
let li = e.target.parentNode.
Also, I'm not sure what you're trying to do with this line:
if(hightlight) {
ul.insertBefore(hightlight, li);
}
If you're just checking if a variable called 'hightlight' exists and trying to insert it before the 'ul' element you do not need the 'if statement' you can just use the code inside the if statement, it should look like this:
ul.insertBefore(hightlight, li);
If you need any more help, comment below this post.

Gavin Eyquem
Front End Web Development Techdegree Student 20,735 PointsI'm getting more confused.
Here's my altered code var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) { if (e.target.tagName == 'BUTTON') { let li = e.target.parentNode; ul.insertBefore(hightlight, li); } });

Siddharth Pandey
7,280 PointsI'm not clear on what's confusing you can you elaborate, also what are you trying to create with this code.
If you are confused about the 'event' and 'e' thing that I told you about, here's a more in-depth explanation: Basically your function parameter 'e' is a reference to the event object, so you should be typing in e.target.tagName, if you want to type in 'event' instead of 'e' you can change the parameter in your function from e to event.
Your altered code does not define 'hightlight' before calling it into the insertBefore method, you must first define 'hightlight.' The rest looks good, though!

Gavin Eyquem
Front End Web Development Techdegree Student 20,735 PointsHi Siddharth,
Basically, confusion has kicked in because I have to go over the course material/code more than once in order to remember. I'm trying to understand how to use the method so that I can go on to the next video.
Sometimes I need to go over something more than once for it to be understood, thanks to you, I have a bit more help.

Siddharth Pandey
7,280 PointsWhat method are you trying to understand to use, I'd be happy to help!

Gavin Eyquem
Front End Web Development Techdegree Student 20,735 PointsIt's the bit inside the if statement.
I'm trying to work out how to do the sibling traversal in the objective.
You can see what I've tried already and I've taken your suggestions into action.

Siddharth Pandey
7,280 PointsI'm not sure what the objective is, but I'll give you a run-through of your code if it helps you to understand sibling traversal better.
var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
let li = event.target.parentNode;
//let p = highlight.previousElementSibling;
let hightlight = li.previousElementSibling;
let ul = li.parentNode;
if(hightlight){
ul.insertBefore(hightlight, li);
}
}
});
Your if statement says: "if the user has clicked on the element with the tagName "BUTTON" execute the following:"
1st line: Creates a variable called 'li' which is the parent node of the element with the tagName "BUTTON"(in your HTML, the element which is the parent of the "BUTTON" element is assigned to the variable 'li,' which in this case, is your actual list item).
2nd line: Creates a variable called 'hightlight' which is the previousElementSibling of the element of your 'li' variable(remember your 'li' variable corresponds to a list item, which is the parent of the button which the user presses, which is the list item on top of the list item which the user has selected).
3rd line: you select the 'ul' which is the parentNode of the list item(this selects the actual 'ul').
Lines 4 and 5: You check if a variable called "highlight" exists, and if so, you insert highlight before the selected list item(I know had told you to change this, but now that I'm looking through your project more, this is actually better practice).
If you still need help, you are welcome to respond, I'm happy to help.

Gavin Eyquem
Front End Web Development Techdegree Student 20,735 PointsNow I'm getting this......One or more of the buttons do not affect their previous sibling paragraphs.
Must be getting closer.

Gavin Eyquem
Front End Web Development Techdegree Student 20,735 PointsAm I any closer to getting it right?
var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) { if (e.target.tagName == 'BUTTON') { let li = e.target.parentNode; let highlight = li.previousElementSibling; let ul = li.parentNode; if(highlight){ ul.insertBefore(highlight, li); } } });
Gavin Eyquem
Front End Web Development Techdegree Student 20,735 PointsGavin Eyquem
Front End Web Development Techdegree Student 20,735 PointsWhat I'll do is go through the video/s again and try, again, to follow the logic of the code.
I'm getting frustrated now but I will try again.
Thankyou for your help!
Siddharth Pandey
7,280 PointsSiddharth Pandey
7,280 PointsYour Welcome! Glad to know you're persevering, that's a key step to being a good programmer, if you still aren't able to get it, I'll give you some more hints.
Good Luck,
Sid
Gavin Eyquem
Front End Web Development Techdegree Student 20,735 PointsGavin Eyquem
Front End Web Development Techdegree Student 20,735 PointsHi Siddharth,
After further research, I've successfully got it right.
I was close to smacking the wall.
Thank you for your help.