Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial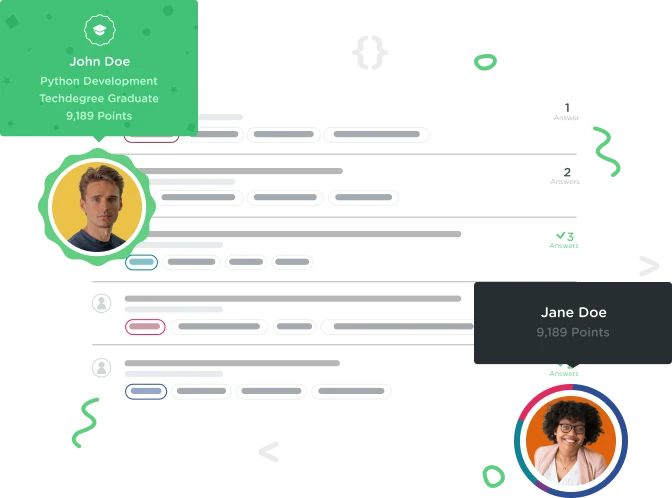

Raouf Tiliouine
15,192 PointsHelp needed on rails progress app
Hi,
I need help on making an app in which a teacher logs in and has a list of students, where he can add/edit/delete a student. When a student's name is clicked on, the teacher will then be redirected to a page where there are static stories in which the teacher can check or uncheck depending on student completion (mind you, the teacher cannot edit the stories, hence the static).
In conclusion, I would like to figure out 3 things:
- I would like to know the controllers/models/associations that are needed to make this work
- How someone could go about having the stories automatically created when a new student is created
- Obviously having the checkboxes save so when the teacher logs out, the changes are remembered
I have looked everywhere and still did not find a tutorial that goes through any of these topics in detail (eg. checkboxes).
I do not necessarily need an answer to all of these questions at once, but any help would be greatly appreciated

Raouf Tiliouine
15,192 PointsHi Brandon,
(btw sorry for the late response)
Well as it is right now, I have generated a scaffold like so:
------- rails g scaffold student name:string age:integer -------
I then generated a Story model with a name:string and a "done" boolean.
Is this the optimal way of doing this?
Also, I'm not sure on how I could go about storing a number of checkboxes in the database. Would I need to enter each name of each story with a boolean checkbox? Or should I maybe use a system of 0 and 1s, with 0 being incomplete and 1 being complete.
For the second questions in my original post, I think the answer might have to do with an initialize method. Maybe?!
That's all I know in this situation, and as for the other questions, I have no clue. I apologize for my lack of knowledge, I am quite new to Rails.
Thanks
2 Answers
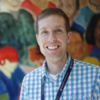
Brandon Barrette
20,485 PointsYou are quite close. You then need another join model called StudentStory that contains the student_id and the story_id. This is where I would but the "done" button. Then your associations would be:
class Story
has_many :student_stories
has_many :students, through: :student_stories
end
class StudentStory
belongs_to :student
belongs_to :story
end
class Student
has_many :student_stories
has_many :stories, through: :student_stories
end
You checkbox solution is then fixed because each checkbox would correspond to a row in the StudentStory table. If it exists, then the checkbox is checked, otherwise it is not. You would use an accepts_nested_attributes_for: :student_stories to achieve that.
Read more here: http://stackoverflow.com/questions/727300/nested-object-w-checkboxes-mass-assignment-even-with-accepts-nested-attribute
and here: http://stackoverflow.com/questions/8860579/rails-3-2-accepts-nested-attributes-for-and-join-models
And to answer your second question, you don't need to worry about it. The stories are independent of the students, so you can have all the stories show up for each student, then use checkboxes to select the ones you want.

Raouf Tiliouine
15,192 PointsI'm new to the join model concept, but I do have some basic knowledge on what is is. Just curious on what command I need to run to make that join model with all the necessary ids.

Raouf Tiliouine
15,192 PointsI would also love to know what to do after the join model, in terms of the forms and such.
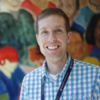
Brandon Barrette
20,485 PointsYou would need to actually generate a new model using the rails generate command
rails generate model student_story student:references story:references
Then you wouldn't need a controller for this, you'd just need to add accepts nested attributes for under the student model. This is some good video about nested attributes (totally worth the $9)
http://railscasts.com/episodes/196-nested-model-form-part-1
I'd create two forms on the student model, one to make new students (the standard) and one to add stories to students. This is where the join model would appear and going to a link say:
website.com/students/4/stories/edit
would bring up the form with all the checkboxes for that student.
If you have other general questions, I'd be glad to help, but that's about as far as I can go. I'd say start coding something up and you can always ask more specific questions in the forum if you get stuck. The only way to really learn is try, fail many times, and learn from those failures. I sure have done it and still do it everyday. I'm always learning new stuff. Good luck!

Raouf Tiliouine
15,192 PointsI actually got it to work! I did have to experiment with AJAX to make it a better user experience, but I think it turned out great in the end. Thanks for the helpful advice, I'll be sure to ask you for some help if i need it in future projects.
Thanks again!
Brandon Barrette
20,485 PointsBrandon Barrette
20,485 PointsHow about you post what you think they should be and we will help guide you =)
This way you are learning and honing your skills. I've built something like this before, so will gladly provide some advice.