Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial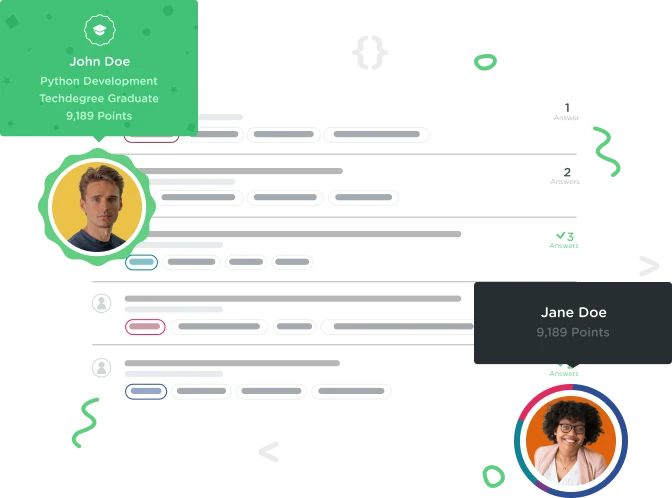

John Haas
1,756 PointsHelp on Ajax request
Was wondering if anyone could catch my error on the challenge question for Ajax. My error is "Uncaught SyntaxError: Unexpected token <". The reference for the error is "web-z03db0l8mk.treehouse-app.com/:1". My code starts after "//this is my code". Here is my javascript code:
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if(xhr.readyState === 4 && xhr.status === 200) {
var employees = JSON.parse(xhr.responseText);
var statusHTML = '<ul class="bulleted">';
for (var i=0; i<employees.length; i += 1) {
if (employees[i].inoffice === true) {
statusHTML += '<li class="in">';
} else {
statusHTML += '<li class="out">';
}
statusHTML += employees[i].name;
statusHTML += '</li>';
}
statusHTML += '</ul>';
document.getElementById('employeeList').innerHTML = statusHTML;
}
};
xhr.open('GET', '../data/employees.json');
xhr.send();
//this is my code
var xhr2 = new XMLHttpRequest();
xhr2.onreadystatechange = function() {
if (xhr2.readyState === 4 && xhr2.status === 200) {
var allStuff = JSON.parse(xhr2.responseText);
console.log(allStuff);
var roomStatus = '<ul class = "rooms">';
for (var i = 0; i < allStuff.length; i++) {
if (JSONdata[i].available == true) {
roomStatus += '<li class = "empty">'; //adds class empty
roomStatus += allStuff[i].room; //adds room number
roomStatus += '</li>';
} else {
roomStatus += '<li class = "full">'; //adds class full
roomStatus += allStuff[i].room; //adds class empty
roomStatus += '</li>';
}
}
roomStatus += '</ul>';
document.getElementById('roomList').innerHTML = roomStatus;
}
};
xhr2.open('GET', '../data/rooms/json');
xhr2.send();
2 Answers
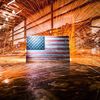
Andrew Shook
31,709 PointsWithout access to the code it'll be a little hard to debug, but my guess it that your AJAX request isn't returning a JSON its returning HTML. Comment out the line 26, the JSON.parse line, and replace it with a console.log(xhr2.responseText). This way you will be able to see what the AJAX request is returning. If its not JSON then you can't use JSON.parse() on it.
EDIT: so looked around in the console and have confirmed that your xhr2 object response property is HTML and not JSON. So the error is being caused because JSON.parse() isn't able to turn HTML into JSON.
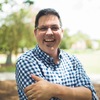
Dave McFarland
Treehouse TeacherHi John Haas
There are a couple of problems:
- The data file isn't loading correctly. You have
xhr2.open('GET', '../data/rooms/json');
But the path to the file is ../data/rooms.json
- In the callback you parse the JSON data and store in in a variable named
allStuff
but use the variableJSONData
when checking the availability:if (JSONdata[i].available == true)
Hope that helps
John Haas
1,756 PointsJohn Haas
1,756 PointsThanks for the help Andrew. It was returning HTML.