Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial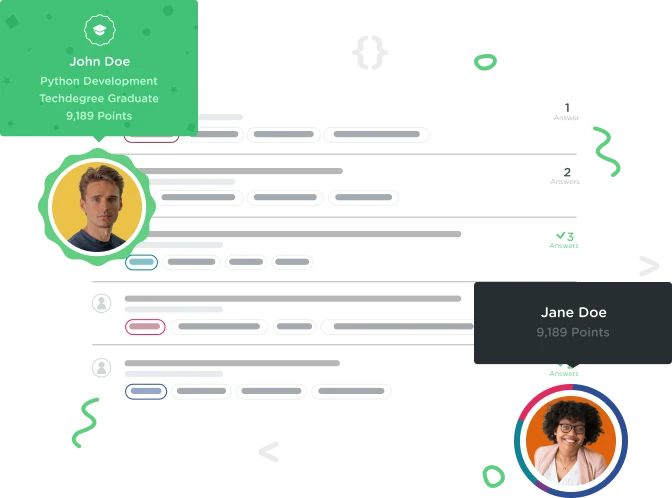

Dominick McCleary
3,012 Pointshelp on foreach loops
i absolutely cannot figure this out. can someone please tell me what i need to fill into the for each loop?
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
int total = 0;
foreach()
{
}
}
}
}
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int TongueLength { get; }
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
2 Answers

andren
28,558 PointsThe structure of a foreach loop is this:
foreach(Type variableName in List/Array)
{
}
Each time though the loop one item will be pulled from the list/array and will be assigned to the variable you declared which will be accessible within the loop. Here is a more practical example showing a foreach loop used for printing out the contents of a string array:
string[] names = {"Eric", "John", "Elizabeth", "William", "Tom", "Ellitot"};
foreach(string name in names)
{
Console.WriteLine(name);
}
/* This will print:
Eric
John
Elizabeth
William
Tom
Ellitot
*/
In the above program the name variable is set equal to the first item in the names array during the first loop ("Eric") then the second item during the second run ("John") and so on until the loop has gone though all of the items. After going through all of the items the loop exits automatically.
In this challenge you have to use the foreach loop to loop though an array of Frog objects so the structure is pretty similar to the for loop above:
foreach(Frog frog in frogs)
{
}
That is how the for loop itself has to be for this task, I'll leave figuring out the rest of the code to you. By looking at the examples above and using your existing C# knowledge it should not be too difficult to solve this task. Though if you get completely stuck I can post the solution for you if you want.
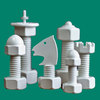
Steven Parker
231,275 Points
I'm guessing you don't want a total spoiler, so here's a few hints:
- you probably want to use the loop to examine each frog in the list
- so your loop might be something like:
foreach (var frog in frogs)
- then inside your loop, you might want to use total to accumulate the sum of the tongue lengths
- finally, after the loop you might divide the total by the number of frogs to get the average
- then you would return that average
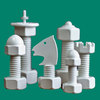
Steven Parker
231,275 Points
Okay, so maybe you do want a "spoiler":
So, just implementing the hints already given above:
int total = 0;
foreach (var frog in frogs)
{
total += frog.TongueLength; // accumulate sum of tongues
}
return total / frogs.Length; // divide by number of frogs and return average
But next time, show us the code that's causing the error so we can try to help you fix it.
Dominick McCleary
3,012 PointsDominick McCleary
3,012 Pointsok i just want a pointer as to what to fill into the loop. everything i try i get a compiler error.
Dominick McCleary
3,012 PointsDominick McCleary
3,012 PointsI am trying it in workspaces but I can't figure out how to total it all up and get the average. I just get compiler errors.