Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial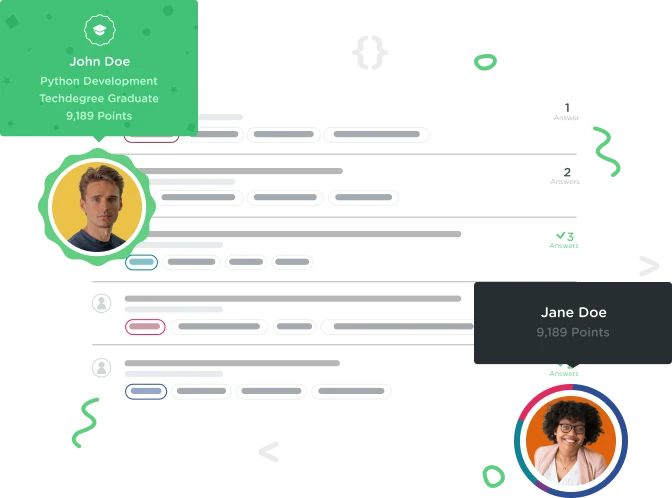

Tim Baggenstos
916 PointsHelp on string_factory.py
Hi, I don't really understand how to approach this challenge. Could someone please help?
Here is what it says: Let's test unpacking dictionaries in keyword arguments. You've used the string .format() method before to fill in blank placeholders. If you give the placeholder a name, though, like in template below, you fill it in through keyword arguments to .format(), like this: template.format(name="Kenneth", food="tacos") Write a function named string_factory that accepts a list of dictionaries as an argument. Return a new list of strings made by using ** for each dictionary in the list and the template string provided.
Thanks for you patience, Tim
# Example:
# values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
# string_factory(values)
# ["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
template = "Hi, I'm {name} and I love to eat {food}!"
2 Answers
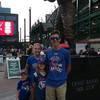
AJ Salmon
5,675 PointsHey Tim, This is a tricky one for sure, and I agree deciding how to start can be mildly infuriating at times, haha. Here's how I completed this challenge: First, you need to create the function and set the parameters for the argument. As the instructions say, this function takes a single argument; a list of dictionaries. Name it whatever you want, I keep it simple and straightforward:
# Example:
# values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
# string_factory(values)
# ["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
template = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(list_of_dicts):
Now that that's out of the way, we move on to the next stage. Here, before you do anything else, notice that the function needs to return a list. Perhaps you're familiar with this technique, but if not it's a very useful and necessary one- create an empty list that you can .append()
later. Again, I like simple names:
# Example:
# values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
# string_factory(values)
# ["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
template = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(list_of_dicts):
new_list = []
Now that we have the list we can append, we can get to the meat of the function. Because the challenge wants our returned list to contain a string for each dictionary in list_of_dicts
, we use a for loop, that loops through list_of_dicts
and performs the commanded action the same number of times a dictionary appears in our list of dictionaries.
# Example:
# values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
# string_factory(values)
# ["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
template = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(list_of_dicts):
new_list = []
for dict in list_of_dicts:
Now whatever we tell it to do will only happen once for every dictionary in list_of_dicts
. Finally, we can use the template string provided and our new_list we made to both create the desired strings and simultaneously append the list. Afterwords, return new_list
. This is where unpacking comes into play:
# Example:
# values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
# string_factory(values)
# ["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
template = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(list_of_dicts):
new_list = []
for dict in list_of_dicts:
new_list.append(template.format(**dict))
return new_list
Unpacking simply takes the dictionary we give it and turns the keys and values into variables. So when it unpacks the dictionary {'name' : 'AJ', 'food' : 'enchiladas'}
, it turns them into normal python variables (like writing name = 'AJ'
, or food = 'enchiladas'
) that can be used with .format()
, for all intents and purposes. Unpacking took me a while to understand, so if you're struggling, I suggest taking a little break and coming back to it later. I think I covered most of this code challenge, but if I did something without explaining why, or you have any other questions, please feel free to ask, and I will answer to the best of my ability :)

Tim Zander
2,994 PointsThank you
Tim Baggenstos
916 PointsTim Baggenstos
916 PointsThanks! It worked.
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsNice explanation!
Victoria Kiplagat
5,507 PointsVictoria Kiplagat
5,507 PointsThanks a lot AJ Salmon for the awesome explanation! This helped me a lot :)
AJ Salmon
5,675 PointsAJ Salmon
5,675 PointsHappy to hear it :)
Ashley Keeling
11,476 PointsAshley Keeling
11,476 PointsThanks for the help, I get it now
Tomme Lang
1,118 PointsTomme Lang
1,118 PointsWould like to see more explanations like this one. Step be step. Thanks AJ!