Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial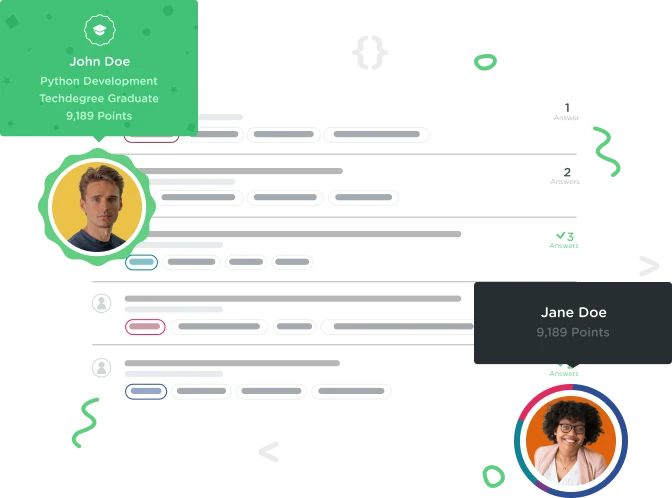

Alex Rogers
9,844 PointsHelp on this (Python Dictionaries)
I'm very lost.
I tried to use a for loop to iterate how many times a word came up in a string, but I get stuck on making sure that those words that come up more than once are not duplicated. I'm not really sure how to fix.
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
comparison = string.lower().split()
newDict = []
for word in comparison:
count = 0
act = word
for j in range(len(comparison)):
if comparison[j] == word:
count += 1
if count > 1:
1 Answer
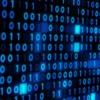
Alexander Davison
65,469 PointsFrom reading your code, I believe that you are looking at every word, then checking how many times that word occurs. Because of this, you have duplicates that you do not need. Of course, this solution might work. But wouldn't it be easier if you didn't need to deal with duplicates? What if the duplicates actually can help you?
You can actually do this. Instead of going through every word and seeing how many times it occurs, than removing duplicates, why don't you simply go through every word, and if the word is not in newDict
, set newDict[word]
to 0, and for every word add 1 to newDict[word]
?
Also note that you made a dictionary using []
, you should use {}
.
The (easier) solution in code:
def word_count(string):
words = string.lower().split()
my_dict = {}
for word in words:
if word not in my_dict:
my_dict[word] = 0
my_dict[word] += 1
return my_dict
I hope this helps!
Happy coding! ~Alex