Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial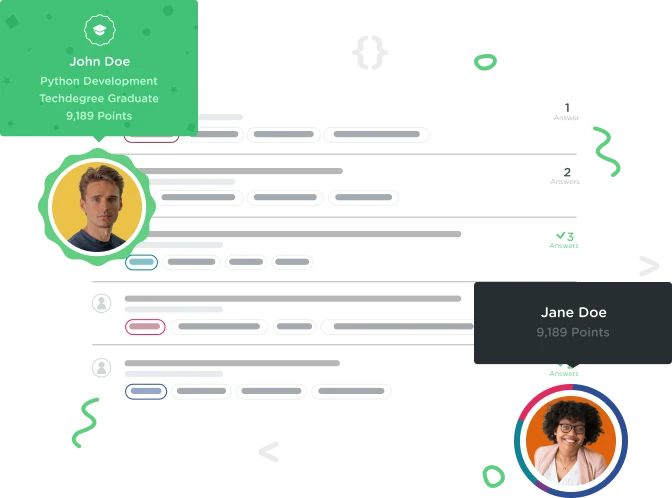

tafadzwa manzunzu
3,601 Pointshelp please
Create a public method in the NumberAnalysis class called NumbersGreaterThanFive that returns an IEnumerable<int>. Inside the method, use LINQ query syntax to return only the numbers in the _numbers field that are greater than 5.
using System.Collections.Generic;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public class NumberAnalysis
{
private List<int> _numbers;
public NumberAnalysis()
{
_numbers = new List<int> { 2, 4, 6, 8, 10 };
IEnumerable<int>NumbersGreaterThanFive = from n in _numbers where n >5 select n;
}
}
}
3 Answers
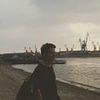
Calin Bogdan
14,921 PointsHowdy!
Instead of creating a method you created an Enumerable with that name in the constructor. You should create a method that returns that Enumerable.
Good luck!
SPOILER
public IEnumerable<int> NumbersGreaterThanFive()
{
return from n in _numbers where n > 5 select n;
}

Brendon Van der nest
12,826 PointsHello!
Here is how I did it.
using System.Collections.Generic;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public class NumberAnalysis
{
private List<int> _numbers;
public NumberAnalysis()
{
_numbers = new List<int> { 2, 4, 6, 8, 10 };
}
public IEnumerable<int> NumbersGreaterThanFive()
{
return _numbers.Where(x => x > 5);
}
}
}
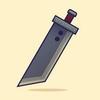
Allan Clark
10,810 PointsFirst, you are required to create a new method for this task. You currently have your linq query in the constructor method. Being that it gives you the name and return type the method signature should look like this:
public IEnumerable<int> NumbersGreaterThanFive()
Your query looks correct so I will leave the implementation up to you!
Happy coding!!!

tafadzwa manzunzu
3,601 Pointsthank you
tafadzwa manzunzu
3,601 Pointstafadzwa manzunzu
3,601 Pointsthank you very much