Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial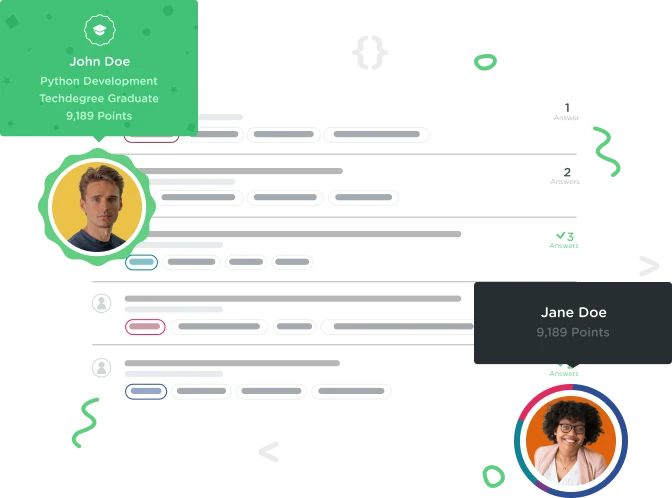

tafadzwa manzunzu
3,601 Pointshelp please
Implement the Subtract method so that the test passes.
public class Calculator
{
public double Result;
public Calculator(double number)
{
Result = number;
}
public void Add(double number)
{
Result += number;
}
public void Subtract(double number)
{
Result -= number;
}
}
using Xunit;
public class CalculatorTests
{
[Fact]
public void Initialization()
{
var expected = 1.1;
var target = new Calculator(1.1);
Assert.Equal(expected, target.Result, 1);
}
[Fact]
public void BasicAdd()
{
var target = new Calculator(1.1);
target.Add(2.2);
var expected = 3.3;
Assert.Equal(expected, target.Result, 1);
}
[Fact]
public void BasicSubtract()
{
var target = new Calculator(1.1);
target.Substract(0.2);
var expected = 0.9;
Assert.Equal(expected, target.Result);
Assert .Equal(double expected,double actual,int precision);
}
}
1 Answer
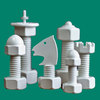
Steven Parker
231,236 PointsI see 3 issues, all in the BasicSubtract testing method:
- there is a reference to "target.Substract" (with an extra "s") instead of "target.Subtract"
- the (first) call to "Assert.Equal" is missing the third argument
- the second call to "Assert.Equal" is not needed and is improper syntax
tafadzwa manzunzu
3,601 Pointstafadzwa manzunzu
3,601 Pointsthank you Steven but the third argument am totally lost what is it ....?
Steven Parker
231,236 PointsSteven Parker
231,236 PointsThe third argument specifies the precision. Take a look at the call done in the BasicAdd function as an example.