Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial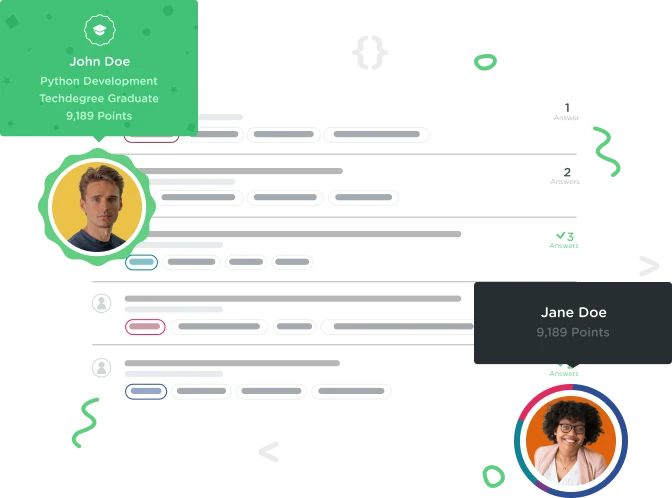
Stormy Silver
9,240 PointsHelp please
The code below creates an array of numbers, loops through them one at a time, and sums their values. PHP actually has a native function that does this same thing: array_sum(). [The array_sum() function receives an array as its one and only argument, and it sends back the sum as the return value.] Modify the code below: remove the foreach loop and the working sum variable, replacing them with a call to the array_sum() function instead.
<?php
$numbers = array(1,5,8);
$sum = 0; foreach($numbers as $number) { $sum = $sum + $number; }
echo $sum; ?>
1 Answer
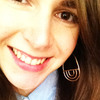
Anna Petry
14,474 PointsIf you call the 'array_sum' function, you'll need to pass it an array, like this:
array_sum($argument);
In this case your array is stored in the variable $numbers.
The function will add all of the values in your array and return the sum. In order to print/echo that return value to the screen, you need to use the keyword 'echo' before your function call, like this:
echo array_sum($argument);
Functions are super cool and powerful, but in order to use them effectively, you must assign their return value to a variable, echo it to the screen, or use that value in another function call, depending on the application.
Hope this helps!
Stone Preston
42,016 PointsStone Preston
42,016 Pointswhat have you tried so far?