Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial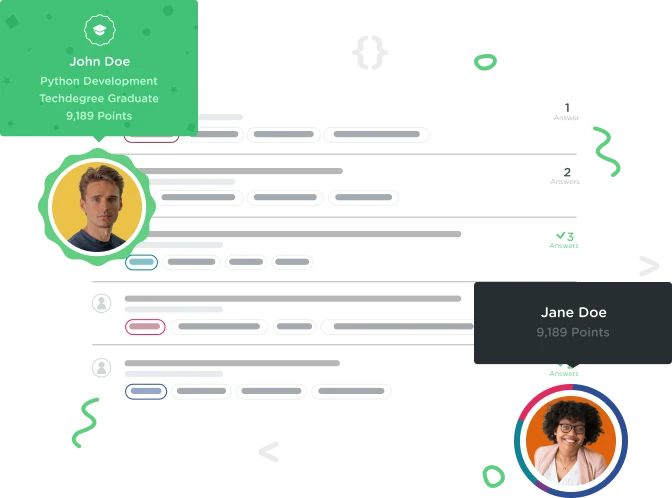
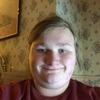
Richard Duffy
16,488 PointsHelp, please :).
Hello,
I would like to get all of my badge id using my json file: http://teamtreehouse.com/richardduffy.json in php but am unsure how to do it, I know how to do it for one but not for more than one.
Thank you.
7 Answers
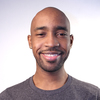
David Tonge
Courses Plus Student 45,640 PointsHey, I got a solution.
Here's the loop you need.
<?php
$url = file_get_contents("http://teamtreehouse.com/richardduffy.json");
$json = json_decode($url,true);
$badges = $json["badges"];
for ($i = 0; $i < count($badges) ; $i++)
{
echo $badges[$i]["icon_url"];
}
?>
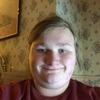
Richard Duffy
16,488 PointsCheers, thanks!
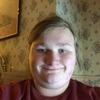
Richard Duffy
16,488 PointsHey, just another quick question how would i do it for points?
Here is my code:
<?php
$url = file_get_contents("http://teamtreehouse.com/richardduffy.json");
$json = json_decode($url,true);
$badges = $json["badges"];
$points = $json["points"];
for ($i = 0; $i < count($points) ; $i++)
{
echo '<li><p>';
echo $points[$i]["number"];
echo '</p></li>';
}
for ($i = 0; $i < count($badges) ; $i++)
{
echo '<li><p>';
echo '<img src="'.$badges[$i]["icon_url"].'">';
echo '</p></li>';
}
?>
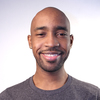
David Tonge
Courses Plus Student 45,640 PointsBasically, you could format this however you'd like.
$points = $json["points"];
<?php
foreach( $points as $key => $value ){
echo $key;
echo $value;
}
?>
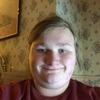
Richard Duffy
16,488 PointsAwesome thanks for your help, I will learn from this and use it my future projects :).
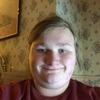
Richard Duffy
16,488 PointsCode:
<?php
$url = file_get_contents("http://teamtreehouse.com/richardduffy.json");
$json = json_decode($url,true);
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, inital-scale=1.0">
<title>Loading</title>
<link rel="stylesheet" href="css/style.css">
<link rel="stylesheet" href="css/global.css">
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
</head>
<body>
<div class="header">
<nav>
<ul>
<div class="col-1-1">
<li><a href="index.html">Home</a></li>
<li><a href="about.html">About</a></li>
<li class="active"><a href="links.php">Links</a></li>
<li><a href="contact.html">Contact</a></li>
</div>
</ul>
</nav>
</div>
<div class="box">
<div class="box1">
<div class="col-1-1">
<h1>My Treehouse Badges!</h1>
<table>
<tr>
<td>
<?php
echo '<img src="'.$json[badges][16][icon_url].'">';
?>
</table>
</div>
</div>
</div>
</body>
</html>
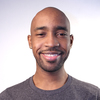
David Tonge
Courses Plus Student 45,640 PointsYou could use a foreach loop here.
<?php foreach( $json as $badgeInfo){ ?>
<td>
// your code here but you can use "$badgeInfo" instead
</td>
<?php } ?>
My code syntax could be off a bit I'll try to get to my main comp to check it soon.
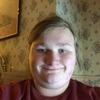
Richard Duffy
16,488 PointsThanks, I was thinking that but that would just pull each badge id, not the ones I have already it would go like 1 2 3 4 5 6 7... ect I need it to follow mine:
{
"name": "Richard Duffy",
"profile_name": "richardduffy",
"profile_url": "http://teamtreehouse.com/richardduffy",
"gravatar_url": "https://secure.gravatar.com/avatar/d6231feab0b1f49dea791c7c0de5bf1e?s=200&d=https%3A%2F%2Fwac.A8B5.edgecastcdn.net%2F80A8B5%2Fstatic-assets%2Fassets%2Fcontent%2Fdefault_avatar-1cc209c72dc4dddf588a2259cdb1d378.png&r=pg",
"gravatar_hash": "d6231feab0b1f49dea791c7c0de5bf1e",
"badges": [
{
"id": 49,
"name": "Newbie",
"url": "http://teamtreehouse.com/richardduffy",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/Generic_Newbie.png",
"earned_date": "2014-05-09T12:43:55.000Z",
"courses": []
},
{
"id": 912,
"name": "Beginning HTML and CSS",
"url": "http://teamtreehouse.com/library/how-to-make-a-website/beginning-html-and-css",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_html_howtomakeawebsite_stage01.png",
"earned_date": "2014-05-09T13:20:20.000Z",
"courses": [
{
"title": "How to Make a Website",
"url": "http://teamtreehouse.com/library/how-to-make-a-website",
"badge_count": 1
},
{
"title": "Beginning HTML and CSS",
"url": "http://teamtreehouse.com/library/how-to-make-a-website/beginning-html-and-css",
"badge_count": 1
}
]
},
{
"id": 922,
"name": "HTML First",
"url": "http://teamtreehouse.com/library/how-to-make-a-website/html-first",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/bagdes_html_howtobuildawebsite_stage02.png",
"earned_date": "2014-05-09T15:02:35.000Z",
"courses": [
{
"title": "How to Make a Website",
"url": "http://teamtreehouse.com/library/how-to-make-a-website",
"badge_count": 1
},
{
"title": "HTML First",
"url": "http://teamtreehouse.com/library/how-to-make-a-website/html-first",
"badge_count": 1
}
]
},
{
"id": 932,
"name": "Creating HTML Content",
"url": "http://teamtreehouse.com/library/how-to-make-a-website/creating-html-content",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/bagdes_html_howtobuildawebsite_stage03.png",
"earned_date": "2014-05-09T17:09:22.000Z",
"courses": [
{
"title": "How to Make a Website",
"url": "http://teamtreehouse.com/library/how-to-make-a-website",
"badge_count": 1
},
{
"title": "Creating HTML Content",
"url": "http://teamtreehouse.com/library/how-to-make-a-website/creating-html-content",
"badge_count": 1
}
]
},
{
"id": 942,
"name": "CSS: Cascading Style Sheets",
"url": "http://teamtreehouse.com/library/how-to-make-a-website/css-cascading-style-sheets",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/bagdes_html_howtobuildawebsite_stage04.png",
"earned_date": "2014-05-09T18:31:05.000Z",
"courses": [
{
"title": "How to Make a Website",
"url": "http://teamtreehouse.com/library/how-to-make-a-website",
"badge_count": 1
},
{
"title": "CSS: Cascading Style Sheets",
"url": "http://teamtreehouse.com/library/how-to-make-a-website/css-cascading-style-sheets",
"badge_count": 1
}
]
},
{
"id": 952,
"name": "Customizing Colors and Fonts",
"url": "http://teamtreehouse.com/library/how-to-make-a-website/customizing-colors-and-fonts",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_html_howtomakeawebsite_stage05.png",
"earned_date": "2014-05-09T19:35:57.000Z",
"courses": [
{
"title": "How to Make a Website",
"url": "http://teamtreehouse.com/library/how-to-make-a-website",
"badge_count": 1
},
{
"title": "Customizing Colors and Fonts",
"url": "http://teamtreehouse.com/library/how-to-make-a-website/customizing-colors-and-fonts",
"badge_count": 1
}
]
},
{
"id": 962,
"name": "Styling Web Pages and Navigation",
"url": "http://teamtreehouse.com/library/how-to-make-a-website/styling-web-pages-and-navigation",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_html_howtomakeawebsite_stage06.png",
"earned_date": "2014-05-09T21:29:16.000Z",
"courses": [
{
"title": "How to Make a Website",
"url": "http://teamtreehouse.com/library/how-to-make-a-website",
"badge_count": 1
},
{
"title": "Styling Web Pages and Navigation",
"url": "http://teamtreehouse.com/library/how-to-make-a-website/styling-web-pages-and-navigation",
"badge_count": 1
}
]
},
{
"id": 972,
"name": "Adding Pages to a Website",
"url": "http://teamtreehouse.com/library/how-to-make-a-website/adding-pages-to-a-website",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_html_howtomakeawebsite_stage07.png",
"earned_date": "2014-05-11T18:00:19.000Z",
"courses": [
{
"title": "How to Make a Website",
"url": "http://teamtreehouse.com/library/how-to-make-a-website",
"badge_count": 1
},
{
"title": "Adding Pages to a Website",
"url": "http://teamtreehouse.com/library/how-to-make-a-website/adding-pages-to-a-website",
"badge_count": 1
}
]
},
{
"id": 982,
"name": "Responsive Web Design and Testing",
"url": "http://teamtreehouse.com/library/how-to-make-a-website/responsive-web-design-and-testing",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_html_howtomakeawebsite_stage08.png",
"earned_date": "2014-05-11T18:58:47.000Z",
"courses": [
{
"title": "How to Make a Website",
"url": "http://teamtreehouse.com/library/how-to-make-a-website",
"badge_count": 1
},
{
"title": "Responsive Web Design and Testing",
"url": "http://teamtreehouse.com/library/how-to-make-a-website/responsive-web-design-and-testing",
"badge_count": 1
}
]
},
{
"id": 992,
"name": "Sharing a Website",
"url": "http://teamtreehouse.com/library/how-to-make-a-website/sharing-a-website",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_html_howtomakeawebsite_stage09.png",
"earned_date": "2014-05-11T19:11:17.000Z",
"courses": [
{
"title": "How to Make a Website",
"url": "http://teamtreehouse.com/library/how-to-make-a-website",
"badge_count": 1
},
{
"title": "Sharing a Website",
"url": "http://teamtreehouse.com/library/how-to-make-a-website/sharing-a-website",
"badge_count": 1
}
]
},
{
"id": 1002,
"name": "Debugging HTML and CSS Problems",
"url": "http://teamtreehouse.com/library/how-to-make-a-website/debugging-html-and-css-problems",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_html_howtomakeawebsite_stage10.png",
"earned_date": "2014-05-12T09:38:32.000Z",
"courses": [
{
"title": "How to Make a Website",
"url": "http://teamtreehouse.com/library/how-to-make-a-website",
"badge_count": 1
},
{
"title": "Debugging HTML and CSS Problems",
"url": "http://teamtreehouse.com/library/how-to-make-a-website/debugging-html-and-css-problems",
"badge_count": 1
}
]
},
{
"id": 180,
"name": "Getting Started with CSS",
"url": "http://teamtreehouse.com/library/css-foundations/getting-started-with-css",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_DD_CSS_Stage1.png",
"earned_date": "2014-05-12T11:21:27.000Z",
"courses": [
{
"title": "CSS Foundations",
"url": "http://teamtreehouse.com/library/css-foundations",
"badge_count": 1
},
{
"title": "Getting Started with CSS",
"url": "http://teamtreehouse.com/library/css-foundations/getting-started-with-css",
"badge_count": 1
}
]
},
{
"id": 26,
"name": "Introduction",
"url": "http://teamtreehouse.com/library/html/introduction",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/HTML_Basics.png",
"earned_date": "2014-05-12T13:47:51.000Z",
"courses": [
{
"title": "HTML",
"url": "http://teamtreehouse.com/library/html",
"badge_count": 1
},
{
"title": "Introduction",
"url": "http://teamtreehouse.com/library/html/introduction",
"badge_count": 1
}
]
},
{
"id": 28,
"name": "Text",
"url": "http://teamtreehouse.com/library/html/text",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/HTML_Text.png",
"earned_date": "2014-05-12T14:40:33.000Z",
"courses": [
{
"title": "HTML",
"url": "http://teamtreehouse.com/library/html",
"badge_count": 1
},
{
"title": "Text",
"url": "http://teamtreehouse.com/library/html/text",
"badge_count": 1
}
]
},
{
"id": 27,
"name": "Lists",
"url": "http://teamtreehouse.com/library/html/lists",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/HTML_Lists.png",
"earned_date": "2014-05-12T16:27:24.000Z",
"courses": [
{
"title": "HTML",
"url": "http://teamtreehouse.com/library/html",
"badge_count": 1
},
{
"title": "Lists",
"url": "http://teamtreehouse.com/library/html/lists",
"badge_count": 1
}
]
},
{
"id": 81,
"name": "Links",
"url": "http://teamtreehouse.com/library/html/links",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/HTML_Links.png",
"earned_date": "2014-05-12T16:53:19.000Z",
"courses": [
{
"title": "HTML",
"url": "http://teamtreehouse.com/library/html",
"badge_count": 1
},
{
"title": "Links",
"url": "http://teamtreehouse.com/library/html/links",
"badge_count": 1
}
]
},
{
"id": 77,
"name": "Objects",
"url": "http://teamtreehouse.com/library/html/objects",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/HTML_Objects.png",
"earned_date": "2014-05-12T19:10:12.000Z",
"courses": [
{
"title": "HTML",
"url": "http://teamtreehouse.com/library/html",
"badge_count": 1
},
{
"title": "Objects",
"url": "http://teamtreehouse.com/library/html/objects",
"badge_count": 1
}
]
},
{
"id": 38,
"name": "Tables",
"url": "http://teamtreehouse.com/library/html/tables",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/HTML_Tables.png",
"earned_date": "2014-05-12T20:13:38.000Z",
"courses": [
{
"title": "HTML",
"url": "http://teamtreehouse.com/library/html",
"badge_count": 1
},
{
"title": "Tables",
"url": "http://teamtreehouse.com/library/html/tables",
"badge_count": 1
}
]
},
{
"id": 29,
"name": "Forms",
"url": "http://teamtreehouse.com/library/html/forms",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/HTML_Forms.png",
"earned_date": "2014-05-13T09:30:39.000Z",
"courses": [
{
"title": "HTML",
"url": "http://teamtreehouse.com/library/html",
"badge_count": 1
},
{
"title": "Forms",
"url": "http://teamtreehouse.com/library/html/forms",
"badge_count": 1
}
]
},
{
"id": 76,
"name": "Basics",
"url": "http://teamtreehouse.com/library/introduction-to-programming/basics",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/Intro_Programming_Basics.png",
"earned_date": "2014-05-13T12:13:54.000Z",
"courses": [
{
"title": "Introduction to Programming",
"url": "http://teamtreehouse.com/library/introduction-to-programming",
"badge_count": 1
},
{
"title": "Basics",
"url": "http://teamtreehouse.com/library/introduction-to-programming/basics",
"badge_count": 1
}
]
},
{
"id": 181,
"name": "Selectors",
"url": "http://teamtreehouse.com/library/css-foundations/selectors",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_DD_CSS_Stage2.png",
"earned_date": "2014-05-14T08:53:37.000Z",
"courses": [
{
"title": "CSS Foundations",
"url": "http://teamtreehouse.com/library/css-foundations",
"badge_count": 1
},
{
"title": "Selectors",
"url": "http://teamtreehouse.com/library/css-foundations/selectors",
"badge_count": 1
}
]
},
{
"id": 401,
"name": "Fundamentals of C",
"url": "http://teamtreehouse.com/library/objectivec-basics/fundamentals-of-c",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_DD_ObjectiveC_Stage1.png",
"earned_date": "2014-05-14T12:49:41.000Z",
"courses": [
{
"title": "Objective-C Basics",
"url": "http://teamtreehouse.com/library/objectivec-basics",
"badge_count": 1
},
{
"title": "Fundamentals of C",
"url": "http://teamtreehouse.com/library/objectivec-basics/fundamentals-of-c",
"badge_count": 1
}
]
},
{
"id": 1102,
"name": "Cocoapods",
"url": "http://teamtreehouse.com/library/ios-tools/cocoapods",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_iOS_iOSTools_Stage1.png",
"earned_date": "2014-05-14T22:50:29.000Z",
"courses": [
{
"title": "iOS Tools",
"url": "http://teamtreehouse.com/library/ios-tools",
"badge_count": 1
},
{
"title": "CocoaPods",
"url": "http://teamtreehouse.com/library/ios-tools/cocoapods",
"badge_count": 1
}
]
},
{
"id": 361,
"name": "HTML Email Basics",
"url": "http://teamtreehouse.com/library/html-email-design/html-email-basics",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_htmlemail_Stage1.png",
"earned_date": "2014-05-16T09:44:20.000Z",
"courses": [
{
"title": "HTML Email Design",
"url": "http://teamtreehouse.com/library/html-email-design",
"badge_count": 1
},
{
"title": "HTML Email Basics",
"url": "http://teamtreehouse.com/library/html-email-design/html-email-basics",
"badge_count": 1
}
]
},
{
"id": 187,
"name": "Getting Started with PHP",
"url": "http://teamtreehouse.com/library/build-a-simple-php-application/getting-started-with-php",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_eCommerce_Stage1.png",
"earned_date": "2014-05-16T13:42:47.000Z",
"courses": [
{
"title": "Build a Simple PHP Application",
"url": "http://teamtreehouse.com/library/build-a-simple-php-application",
"badge_count": 1
},
{
"title": "Getting Started with PHP",
"url": "http://teamtreehouse.com/library/build-a-simple-php-application/getting-started-with-php",
"badge_count": 1
}
]
},
{
"id": 386,
"name": "Starting a Freelance Career",
"url": "http://teamtreehouse.com/library/how-to-freelance/starting-a-freelance-career",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_HowToFreelance_Stage1.png",
"earned_date": "2014-05-22T12:40:56.000Z",
"courses": [
{
"title": "How to Freelance ",
"url": "http://teamtreehouse.com/library/how-to-freelance",
"badge_count": 1
},
{
"title": "Starting a Freelance Career",
"url": "http://teamtreehouse.com/library/how-to-freelance/starting-a-freelance-career",
"badge_count": 1
}
]
},
{
"id": 343,
"name": "Fair Use and Public Domain",
"url": "http://teamtreehouse.com/library/copyright-basics/fair-use-and-public-domain",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_DD_Copyright_Stage2-53.png",
"earned_date": "2014-05-24T17:04:41.000Z",
"courses": [
{
"title": "Copyright Basics",
"url": "http://teamtreehouse.com/library/copyright-basics",
"badge_count": 1
},
{
"title": "Fair Use and Public Domain",
"url": "http://teamtreehouse.com/library/copyright-basics/fair-use-and-public-domain",
"badge_count": 1
}
]
},
{
"id": 344,
"name": "Licensing",
"url": "http://teamtreehouse.com/library/copyright-basics/licensing",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_DD_Copyright_Stage3-54.png",
"earned_date": "2014-05-24T17:42:00.000Z",
"courses": [
{
"title": "Copyright Basics",
"url": "http://teamtreehouse.com/library/copyright-basics",
"badge_count": 1
},
{
"title": "Licensing",
"url": "http://teamtreehouse.com/library/copyright-basics/licensing",
"badge_count": 1
}
]
},
{
"id": 345,
"name": "Protecting and Sharing Your Work",
"url": "http://teamtreehouse.com/library/copyright-basics/protecting-and-sharing-your-work",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_DD_Copyright_Stage4-55.png",
"earned_date": "2014-05-24T18:09:03.000Z",
"courses": [
{
"title": "Copyright Basics",
"url": "http://teamtreehouse.com/library/copyright-basics",
"badge_count": 1
},
{
"title": "Protecting and Sharing Your Work",
"url": "http://teamtreehouse.com/library/copyright-basics/protecting-and-sharing-your-work",
"badge_count": 1
}
]
},
{
"id": 342,
"name": "Getting Started with Copyright",
"url": "http://teamtreehouse.com/library/copyright-basics/getting-started-with-copyright",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_DD_Copyright_Stage1-52.png",
"earned_date": "2014-05-24T19:01:44.000Z",
"courses": [
{
"title": "Copyright Basics",
"url": "http://teamtreehouse.com/library/copyright-basics",
"badge_count": 1
},
{
"title": "Getting Started with Copyright",
"url": "http://teamtreehouse.com/library/copyright-basics/getting-started-with-copyright",
"badge_count": 1
}
]
},
{
"id": 160,
"name": "Why Start a Business?",
"url": "http://teamtreehouse.com/library/how-to-start-a-business/why-start-a-business",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_StartBusiness_Stage1.png",
"earned_date": "2014-05-24T21:22:22.000Z",
"courses": [
{
"title": "How to Start a Business (old)",
"url": "http://teamtreehouse.com/library/how-to-start-a-business-old",
"badge_count": 1
},
{
"title": "Why Start a Business?",
"url": "http://teamtreehouse.com/library/how-to-start-a-business/why-start-a-business",
"badge_count": 1
}
]
},
{
"id": 532,
"name": "Introduction to UI Design",
"url": "http://teamtreehouse.com/library/mobile-app-design-for-ios/introduction-to-ui-design",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_mobiledesign_Stage1.png",
"earned_date": "2014-05-26T22:04:29.000Z",
"courses": [
{
"title": "Mobile App Design for iOS",
"url": "http://teamtreehouse.com/library/mobile-app-design-for-ios",
"badge_count": 1
},
{
"title": "Introduction to UI Design",
"url": "http://teamtreehouse.com/library/mobile-app-design-for-ios/introduction-to-ui-design",
"badge_count": 1
}
]
},
{
"id": 182,
"name": "Advanced Selectors",
"url": "http://teamtreehouse.com/library/css-foundations/advanced-selectors",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_DD_CSS_Stage3.png",
"earned_date": "2014-06-02T10:38:32.000Z",
"courses": [
{
"title": "CSS Foundations",
"url": "http://teamtreehouse.com/library/css-foundations",
"badge_count": 1
},
{
"title": "Advanced Selectors",
"url": "http://teamtreehouse.com/library/css-foundations/advanced-selectors",
"badge_count": 1
}
]
},
{
"id": 184,
"name": "Values and Units",
"url": "http://teamtreehouse.com/library/css-foundations/values-and-units",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_DD_CSS_Stage4.png",
"earned_date": "2014-06-09T13:30:27.000Z",
"courses": [
{
"title": "CSS Foundations",
"url": "http://teamtreehouse.com/library/css-foundations",
"badge_count": 1
},
{
"title": "Values and Units",
"url": "http://teamtreehouse.com/library/css-foundations/values-and-units",
"badge_count": 1
}
]
},
{
"id": 195,
"name": "Text, Fonts, and Lists",
"url": "http://teamtreehouse.com/library/css-foundations/text-fonts-and-lists",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_DD_CSS_Stage5.png",
"earned_date": "2014-06-15T00:05:37.000Z",
"courses": [
{
"title": "CSS Foundations",
"url": "http://teamtreehouse.com/library/css-foundations",
"badge_count": 1
},
{
"title": "Text, Fonts, and Lists",
"url": "http://teamtreehouse.com/library/css-foundations/text-fonts-and-lists",
"badge_count": 1
}
]
},
{
"id": 78,
"name": "Control Structures",
"url": "http://teamtreehouse.com/library/introduction-to-programming/control-structures",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/Intro_Programming_ControlStructures.png",
"earned_date": "2014-06-17T11:55:04.000Z",
"courses": [
{
"title": "Introduction to Programming",
"url": "http://teamtreehouse.com/library/introduction-to-programming",
"badge_count": 1
},
{
"title": "Control Structures",
"url": "http://teamtreehouse.com/library/introduction-to-programming/control-structures",
"badge_count": 1
}
]
},
{
"id": 1622,
"name": "AJAX Concepts",
"url": "http://teamtreehouse.com/library/ajax-basics/ajax-concepts",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_JavaScript_AJAXBasics_Stage1.png",
"earned_date": "2014-07-01T17:31:22.000Z",
"courses": [
{
"title": "AJAX Basics",
"url": "http://teamtreehouse.com/library/ajax-basics",
"badge_count": 1
},
{
"title": "AJAX Concepts",
"url": "http://teamtreehouse.com/library/ajax-basics/ajax-concepts",
"badge_count": 1
}
]
},
{
"id": 1632,
"name": "Programming AJAX",
"url": "http://teamtreehouse.com/library/ajax-basics/programming-ajax",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_JavaScript_AJAXBasics_Stage2.png",
"earned_date": "2014-07-08T12:55:30.000Z",
"courses": [
{
"title": "AJAX Basics",
"url": "http://teamtreehouse.com/library/ajax-basics",
"badge_count": 1
},
{
"title": "Programming AJAX",
"url": "http://teamtreehouse.com/library/ajax-basics/programming-ajax",
"badge_count": 1
}
]
},
{
"id": 201,
"name": "CSS Box Model",
"url": "http://teamtreehouse.com/library/css-foundations/the-box-model",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_DD_CSS_Stage6.png",
"earned_date": "2014-07-13T11:52:04.000Z",
"courses": [
{
"title": "CSS Foundations",
"url": "http://teamtreehouse.com/library/css-foundations",
"badge_count": 1
},
{
"title": "The Box Model",
"url": "http://teamtreehouse.com/library/css-foundations/the-box-model",
"badge_count": 1
}
]
},
{
"id": 1942,
"name": "Say Hello to Python",
"url": "http://teamtreehouse.com/library/python-basics/say-hello-to-python",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_python_basics_stage1.png",
"earned_date": "2014-07-23T19:26:35.000Z",
"courses": [
{
"title": "Python Basics",
"url": "http://teamtreehouse.com/library/python-basics",
"badge_count": 1
},
{
"title": "Say Hello to Python",
"url": "http://teamtreehouse.com/library/python-basics/say-hello-to-python",
"badge_count": 1
}
]
},
{
"id": 1962,
"name": "Ins & Outs",
"url": "http://teamtreehouse.com/library/python-basics/ins-outs",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_python_basics_stage2.png",
"earned_date": "2014-07-24T06:29:43.000Z",
"courses": [
{
"title": "Python Basics",
"url": "http://teamtreehouse.com/library/python-basics",
"badge_count": 1
},
{
"title": "Ins & Outs",
"url": "http://teamtreehouse.com/library/python-basics/ins-outs",
"badge_count": 1
}
]
},
{
"id": 1972,
"name": "Things That Count",
"url": "http://teamtreehouse.com/library/python-basics/things-that-count",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_python_basics_stage3.png",
"earned_date": "2014-07-28T20:58:24.000Z",
"courses": [
{
"title": "Python Basics",
"url": "http://teamtreehouse.com/library/python-basics",
"badge_count": 1
},
{
"title": "Things That Count",
"url": "http://teamtreehouse.com/library/python-basics/things-that-count",
"badge_count": 1
}
]
},
{
"id": 80,
"name": "Objects and Arrays",
"url": "http://teamtreehouse.com/library/introduction-to-programming/objects-and-arrays",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/Intro_Programming_ObjectsArrays.png",
"earned_date": "2014-08-11T10:07:02.000Z",
"courses": [
{
"title": "Introduction to Programming",
"url": "http://teamtreehouse.com/library/introduction-to-programming",
"badge_count": 1
},
{
"title": "Objects and Arrays",
"url": "http://teamtreehouse.com/library/introduction-to-programming/objects-and-arrays",
"badge_count": 1
}
]
},
{
"id": 79,
"name": "Functions",
"url": "http://teamtreehouse.com/library/introduction-to-programming/functions",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/Intro_Programming_Functions.png",
"earned_date": "2014-08-11T10:20:34.000Z",
"courses": [
{
"title": "Introduction to Programming",
"url": "http://teamtreehouse.com/library/introduction-to-programming",
"badge_count": 1
},
{
"title": "Functions",
"url": "http://teamtreehouse.com/library/introduction-to-programming/functions",
"badge_count": 1
}
]
},
{
"id": 2352,
"name": "How Ruby Works",
"url": "http://teamtreehouse.com/library/ruby-basics/how-ruby-works",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_ruby_basics_Stage1.png",
"earned_date": "2014-08-11T10:43:31.000Z",
"courses": [
{
"title": "Ruby Basics",
"url": "http://teamtreehouse.com/library/ruby-basics",
"badge_count": 1
},
{
"title": "How Ruby Works",
"url": "http://teamtreehouse.com/library/ruby-basics/how-ruby-works",
"badge_count": 1
}
]
},
{
"id": 2362,
"name": "Ruby Strings (Ruby Basics)",
"url": "http://teamtreehouse.com/library/ruby-basics/ruby-strings",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_ruby_basics_Stage2.png",
"earned_date": "2014-08-11T11:17:17.000Z",
"courses": [
{
"title": "Ruby Basics",
"url": "http://teamtreehouse.com/library/ruby-basics",
"badge_count": 1
},
{
"title": "Ruby Strings",
"url": "http://teamtreehouse.com/library/ruby-basics/ruby-strings",
"badge_count": 1
}
]
},
{
"id": 2372,
"name": "Ruby Numbers (Ruby Basics)",
"url": "http://teamtreehouse.com/library/ruby-basics/ruby-numbers",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_ruby_basics_Stage3.png",
"earned_date": "2014-08-11T11:33:54.000Z",
"courses": [
{
"title": "Ruby Basics",
"url": "http://teamtreehouse.com/library/ruby-basics",
"badge_count": 1
},
{
"title": "Ruby Numbers",
"url": "http://teamtreehouse.com/library/ruby-basics/ruby-numbers",
"badge_count": 1
}
]
},
{
"id": 2412,
"name": "Ruby Methods (Ruby Basics)",
"url": "http://teamtreehouse.com/library/ruby-basics/ruby-methods",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_ruby_basics_Stage4.png",
"earned_date": "2014-08-11T12:10:20.000Z",
"courses": [
{
"title": "Ruby Basics",
"url": "http://teamtreehouse.com/library/ruby-basics",
"badge_count": 1
},
{
"title": "Ruby Methods",
"url": "http://teamtreehouse.com/library/ruby-basics/ruby-methods",
"badge_count": 1
}
]
},
{
"id": 192,
"name": "Creating the Menu and Footer",
"url": "http://teamtreehouse.com/library/build-a-simple-php-application/creating-the-menu-and-footer",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_eCommerce_Stage2.png",
"earned_date": "2014-08-12T08:55:59.000Z",
"courses": [
{
"title": "Build a Simple PHP Application",
"url": "http://teamtreehouse.com/library/build-a-simple-php-application",
"badge_count": 1
},
{
"title": "Creating the Menu and Footer",
"url": "http://teamtreehouse.com/library/build-a-simple-php-application/creating-the-menu-and-footer",
"badge_count": 1
}
]
},
{
"id": 462,
"name": "Installing a Ruby Development Environment",
"url": "http://teamtreehouse.com/library/installing-a-ruby-development-environment/installing-a-ruby-development-environment",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_environment_Stage1.png",
"earned_date": "2014-08-13T17:44:36.000Z",
"courses": [
{
"title": "Installing a Ruby Development Environment",
"url": "http://teamtreehouse.com/library/installing-a-ruby-development-environment",
"badge_count": 1
},
{
"title": "Installing a Ruby Development Environment",
"url": "http://teamtreehouse.com/library/installing-a-ruby-development-environment/installing-a-ruby-development-environment",
"badge_count": 1
}
]
},
{
"id": 163,
"name": "Getting Started With Rails",
"url": "http://teamtreehouse.com/library/build-a-simple-ruby-on-rails-application/getting-started-with-rails",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_SimpleFacebook_Stage1.png",
"earned_date": "2014-08-14T12:28:02.000Z",
"courses": [
{
"title": "Build a Simple Ruby on Rails Application",
"url": "http://teamtreehouse.com/library/build-a-simple-ruby-on-rails-application",
"badge_count": 1
},
{
"title": "Getting Started with Rails",
"url": "http://teamtreehouse.com/library/build-a-simple-ruby-on-rails-application/getting-started-with-rails",
"badge_count": 1
}
]
},
{
"id": 166,
"name": "Rails Frontend Development",
"url": "http://teamtreehouse.com/library/build-a-simple-ruby-on-rails-application/frontend-development",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_SimpleFacebook_Stage2.png",
"earned_date": "2014-08-14T14:34:11.000Z",
"courses": [
{
"title": "Build a Simple Ruby on Rails Application",
"url": "http://teamtreehouse.com/library/build-a-simple-ruby-on-rails-application",
"badge_count": 1
},
{
"title": "Frontend Development",
"url": "http://teamtreehouse.com/library/build-a-simple-ruby-on-rails-application/frontend-development",
"badge_count": 1
}
]
},
{
"id": 168,
"name": "Ruby on Rails Authentication",
"url": "http://teamtreehouse.com/library/build-a-simple-ruby-on-rails-application/creating-an-authentication-system",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/badges_SimpleFacebook_Stage3.png",
"earned_date": "2014-08-14T17:23:08.000Z",
"courses": [
{
"title": "Build a Simple Ruby on Rails Application",
"url": "http://teamtreehouse.com/library/build-a-simple-ruby-on-rails-application",
"badge_count": 1
},
{
"title": "Creating an Authentication System",
"url": "http://teamtreehouse.com/library/build-a-simple-ruby-on-rails-application/creating-an-authentication-system",
"badge_count": 1
}
]
},
{
"id": 90,
"name": "Ruby Basics",
"url": "http://teamtreehouse.com/library/ruby-foundations/ruby-basics",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/Ruby_Basics.png",
"earned_date": "2014-08-15T11:25:20.000Z",
"courses": [
{
"title": "Ruby Foundations",
"url": "http://teamtreehouse.com/library/ruby-foundations",
"badge_count": 1
},
{
"title": "Ruby Basics",
"url": "http://teamtreehouse.com/library/ruby-foundations/ruby-basics",
"badge_count": 1
}
]
},
{
"id": 104,
"name": "Objects, Classes, and Variables",
"url": "http://teamtreehouse.com/library/ruby-foundations/objects-classes-and-variables",
"icon_url": "https://wac.A8B5.edgecastcdn.net/80A8B5/achievement-images/ROR_LanguageConstructs.png",
"earned_date": "2014-08-15T11:42:40.000Z",
"courses": [
{
"title": "Ruby Foundations",
"url": "http://teamtreehouse.com/library/ruby-foundations",
"badge_count": 1
},
{
"title": "Objects, Classes, and Variables",
"url": "http://teamtreehouse.com/library/ruby-foundations/objects-classes-and-variables",
"badge_count": 1
}
]
}
],
"points": {
"total": 3765,
"HTML": 682,
"CSS": 794,
"Design": 92,
"JavaScript": 490,
"Ruby": 770,
"PHP": 168,
"WordPress": 6,
"iOS": 198,
"Android": 12,
"Development Tools": 30,
"Business": 330,
"Python": 97
}
}
David Tonge
Courses Plus Student 45,640 PointsDavid Tonge
Courses Plus Student 45,640 PointsPost the code that you're going to use to pull the info for one of your badges then I'm pretty sure someone will be able to help you from there.