Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial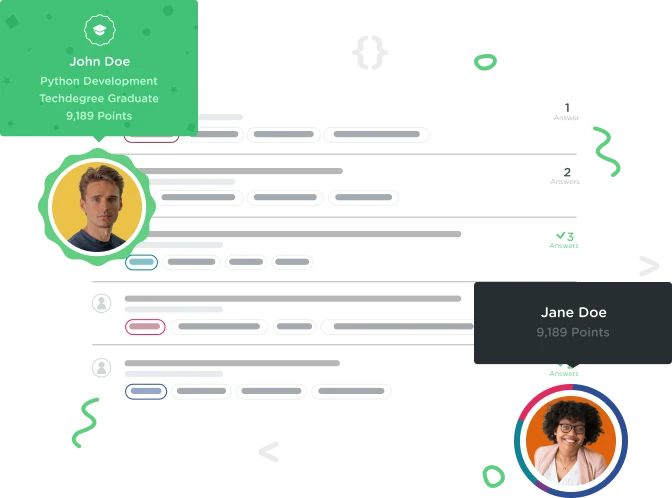

Yiu Ming Lai
5,861 PointsHelp please challenge task 2.
I am getting this, anyone know what i did wrong?
LexicalAnalysis.cs(23,23): error CS1520: Class, struct, or interface method must have a return type Compilation failed: 1 error(s), 0 warnings
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class LexicalAnalysis
{
public Dictionary<string, int> WordCount = new Dictionary<string, int>();
public void AddWord(string word)
{
if ( !WordCount.ContainsKey(word))
{
WordCount.Add(word,1);
}
else
{
WordCount[word] +=1;
}
}
public Dictionary<string, int> newList = new Dictionary<string, int>();
public static WordsWithCountGreaterThan(int num)
{
foreach(var word in WordCount)
{
int itemValue = word.Value;
if( itemValue >= num)
{
newList.Add(word.Key,word.Value);
}
}
return newList;
}
}
}
2 Answers
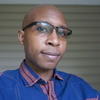
Tony Thigpen
36,903 PointsI believe this is what you may be looking for:
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class LexicalAnalysis
{
public Dictionary<string, int> WordCount = new Dictionary<string, int>();
public void AddWord(string word)
{
if (!WordCount.ContainsKey(word))
{
WordCount.Add(word, 1);
}
else
{
WordCount[word] += 1;
}
}
public Dictionary<string, int> WordsWithCountGreaterThan(int num)
{
Dictionary<string, int> newList = new Dictionary<string, int> { };
//newList should be defined locally here.
foreach (var word in WordCount)
{
if (word.Value > num)
//can combine word.Value with "> num" to shorten
{
newList.Add(word.Key, word.Value);
}
}
return newList;
}
}
}
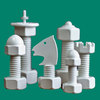
Steven Parker
231,269 Points
You left off the return type in the definition of WordsWithCountGreaterThan.
You wrote:
public static WordsWithCountGreaterThan(int num)
But it should be:
public Dictionary<string, int> WordsWithCountGreaterThan(int num)
You have a couple of additional issues to address:
- You need to define newList before it is used.
- The challenge says to check for "greater than" but your code has "
>=
" (greater or equal).