Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial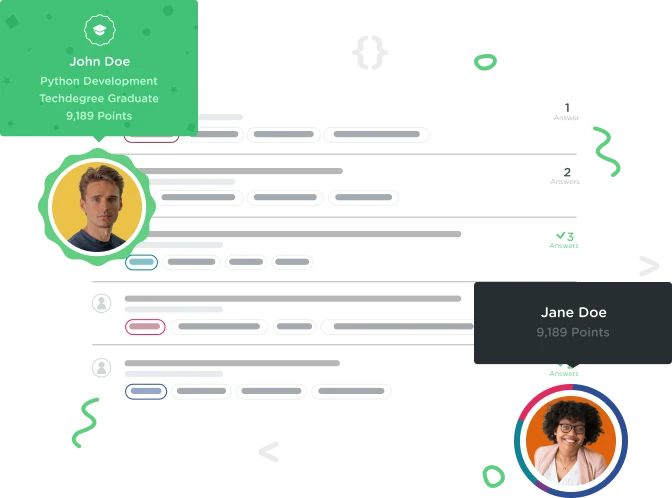
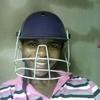
Samson Chapuramhuka
7,390 PointsHelp please, my second time to post this same question.
Now I need you to update the create_user method so that it sets the User instance's password using the User.hash_password method you just created. You should see the TODO for where to add this.
import datetime
from argon2 import PasswordHasher
from peewee import *
DATABASE = SqliteDatabase('recipes.sqlite')
HASHER = PasswordHasher()
class User(Model):
username = CharField(unique=True)
email = CharField(unique=True)
password = CharField()
@classmethod
def create_user(cls, username, password):
try:
cls.get(cls.username**username)
except cls.DoesNotExist:
user = PasswordHasher()
hash_password = user.hash_password("pass$word") #Here the class instance is calling the static method we just made
return hash_password
@staticmethod
def hash_password(password): #function takes in user password and hashes it
return HASHER.hash(password)
class Recipe(Model):
name = CharField()
created_at = DateTimeField(default=datetime.datetime.now)
class Meta:
database = DATABASE
class Ingredient(Model):
name = CharField()
description = CharField()
quantity = DecimalField()
measurement_type = CharField()
recipe = ForeignKeyField(Recipe)
class Meta:
database = DATABASE
def initialize():
DATABASE.connect()
DATABASE.create_tables([User, Recipe, Ingredient], safe=True)
DATABASE.close()
1 Answer
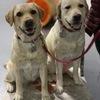
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Samson,
Let's start with the errors in this line:
hash_password = user.hash_password("pass$word") #Here the class instance is calling the static method we just made
First, you're never hashing the password provided by the user (in the password
variable), you're always hashing the string "pass$word". Thus you'll always get the wrong hash unless, by some amazing coincidence, the user specified that their password is "pass$word".
Next, you assign the hashed password to the hash_password
variable, but you never update the user
object with this hashed password (remember that User
objects have a password
attribute).
Now let's look at the code in the rest of the method.
Here is what the provided create_user
method looks like in the challenge:
@classmethod
def create_user(cls, username, password):
try:
cls.get(cls.username**username)
except cls.DoesNotExist:
user = cls(username=username)
# TODO: hash user password here?
user.save()
return user
else:
raise Exception("User already exists")
Observe that method saves a user to the database and then returns that user (or raises an exception if the user already exists).
Now let's look at your method:
@classmethod
def create_user(cls, username, password):
try:
cls.get(cls.username**username)
except cls.DoesNotExist:
user = PasswordHasher()
hash_password = user.hash_password("pass$word") #Here the class instance is calling the static method we just made
return hash_password
Your method never saves the user to the database. Since the original method did this, we should assume that this is required functionality.
Your method returns a hashed password string instead of a User object. Since the original method returned a user, we should assume that program will only function correctly when create_user
returns a User object (or raises an exception in the case of an existing user) and not a string.
Hope these hints point you in the right direction.
Cheers
Alex
Samson Chapuramhuka
7,390 PointsSamson Chapuramhuka
7,390 PointsThank you Sir, done!