Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial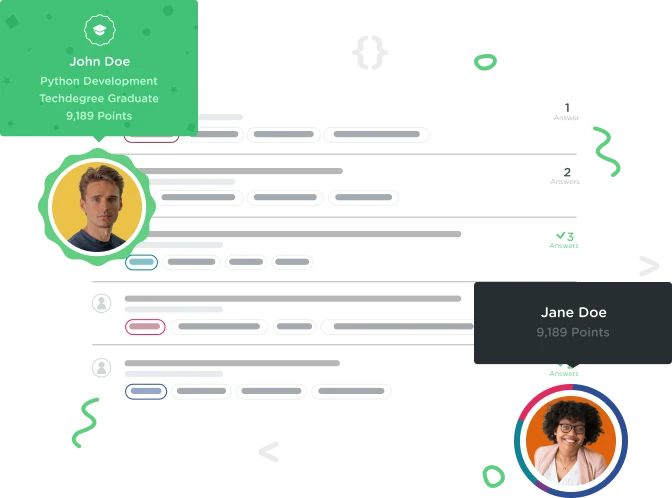

Petter Jonasson
808 PointsHelp. Pls make a place wher you can find the answers for the problems you get after a video.
I've created a tool to help manage lines at tech conferences. The organizers would like to split the attendees into two lines during the registration process. I've added notes, examples and a new lesson in the Example.java tab.
Fix the getLineNumberFor method to return a 1 if the first character of lastName is between A and M or else return 2 if it is between N and Z.
This questions after the videos is almost killing my coding intrest. Pls can i get help.
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
return lineNumber;
}
}
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
1 Answer

Yanuar Prakoso
15,196 PointsHi Petter...
All you have to do is tell Java to check if the first character of the lastName variable passed as method argument is less than 'M' character or not. Do not worry Java knows the alphabetical order really well when it comes to String and char type variables. This is how the code should looks like:
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
if (lastName.toUpperCase().charAt(0) <= 'M') {//<- If the first character (index 0) of lastName smaller or equal 'M' then:
lineNumber = 1;//<- it will go to Line 1
} else {lineNumber = 2;}//<- other than that (meaning the first character is greater than 'M' it goes to Line 2
return lineNumber;
}
/*PS: I use lastName.toUpperCase to ensure all characters in lastName is all Capitalized first, however
* you do not have to actually since all test in the Example class already wrote the first character of lastName
* using capital letters (for example : Zimmerman)
*/
}
I hope this can help a little. Keep the good work and happy coding